Comparing version 1.3.2 to 1.3.3
/** | ||
* Returns true if input number type is a literal | ||
*/ | ||
type IsNumberLiteral<T extends number> = [T] extends [number] ? [number] extends [T] ? false : true : false; | ||
type IsBooleanLiteral<T extends boolean> = [T] extends [boolean] ? [boolean] extends [T] ? false : true : false; | ||
/** | ||
* Returns true if any elements in boolean array are the literal true (not false or boolean) | ||
*/ | ||
type Any<Arr extends boolean[]> = Arr extends [ | ||
infer Head extends boolean, | ||
...infer Rest extends boolean[] | ||
] ? IsBooleanLiteral<Head> extends true ? Head extends true ? true : Any<Rest> : Any<Rest> : false; | ||
/** | ||
* Returns true if every element in boolean array is the literal true (not false or boolean) | ||
*/ | ||
type All<Arr extends boolean[]> = IsBooleanLiteral<Arr[number]> extends true ? Arr extends [infer Head extends boolean, ...infer Rest extends boolean[]] ? Head extends true ? Any<Rest> : false : true : false; | ||
/** | ||
* Returns true if string input type is a literal | ||
*/ | ||
type IsStringLiteral<T extends string> = [T] extends [string] ? [string] extends [T] ? false : Uppercase<T> extends Uppercase<Lowercase<T>> ? Lowercase<T> extends Lowercase<Uppercase<T>> ? true : false : false : false; | ||
type IsStringLiteralArray<Arr extends string[] | readonly string[]> = IsStringLiteral<Arr[number]> extends true ? true : false; | ||
/** | ||
* Splits a string into an array of substrings. | ||
@@ -6,3 +28,3 @@ * T: The string to split. | ||
*/ | ||
type Split<T, delimiter extends string = ''> = T extends `${infer first}${delimiter}${infer rest}` ? [first, ...Split<rest, delimiter>] : T extends '' ? [] : [T]; | ||
type Split<T extends string, delimiter extends string = ''> = IsStringLiteral<T | delimiter> extends true ? T extends `${infer first}${delimiter}${infer rest}` ? [first, ...Split<rest, delimiter>] : T extends '' ? [] : [T] : string[]; | ||
/** | ||
@@ -22,3 +44,6 @@ * A strongly-typed version of `String.prototype.split`. | ||
*/ | ||
type CharAt<T extends string, index extends number> = Split<T>[index]; | ||
type CharAt<T extends string, index extends number> = All<[ | ||
IsStringLiteral<T>, | ||
IsNumberLiteral<index> | ||
]> extends true ? Split<T>[index] : string; | ||
/** | ||
@@ -38,6 +63,6 @@ * A strongly-typed version of `String.prototype.charAt`. | ||
*/ | ||
type Join<T extends readonly string[], delimiter extends string = ''> = string[] extends T ? string : T extends readonly [ | ||
type Join<T extends readonly string[], delimiter extends string = ''> = All<[IsStringLiteralArray<T>, IsStringLiteral<delimiter>]> extends true ? T extends readonly [ | ||
infer first extends string, | ||
...infer rest extends string[] | ||
] ? rest extends [] ? first : `${first}${delimiter}${Join<rest, delimiter>}` : ''; | ||
] ? rest extends [] ? first : `${first}${delimiter}${Join<rest, delimiter>}` : '' : string; | ||
/** | ||
@@ -68,3 +93,3 @@ * A strongly-typed version of `Array.prototype.join`. | ||
*/ | ||
type Length<T extends string> = Split<T>['length']; | ||
type Length<T extends string> = IsStringLiteral<T> extends true ? Split<T>['length'] : number; | ||
/** | ||
@@ -95,3 +120,3 @@ * A strongly-typed version of `String.prototype.length`. | ||
*/ | ||
type DropSuffix<sentence extends string, suffix extends string> = sentence extends `${infer rest}${suffix}` ? rest : sentence; | ||
type DropSuffix<sentence extends string, suffix extends string> = string extends sentence | suffix ? string : sentence extends `${infer rest}${suffix}` ? rest : sentence; | ||
/** | ||
@@ -103,4 +128,4 @@ * Returns a tuple of the given length with the given type. | ||
declare namespace Math { | ||
type Subtract<A extends number, B extends number> = TupleOf<A> extends [...infer U, ...TupleOf<B>] ? U['length'] : 0; | ||
type IsNegative<T extends number> = `${T}` extends `-${number}` ? true : false; | ||
type Subtract<A extends number, B extends number> = number extends A | B ? number : TupleOf<A> extends [...infer U, ...TupleOf<B>] ? U['length'] : 0; | ||
type IsNegative<T extends number> = number extends T ? boolean : `${T}` extends `-${number}` ? true : false; | ||
type Abs<T extends number> = `${T}` extends `-${infer U extends number}` ? U : T; | ||
@@ -116,3 +141,6 @@ type GetPositiveIndex<T extends string, I extends number> = IsNegative<I> extends false ? I : Subtract<Length<T>, Abs<I>>; | ||
*/ | ||
type Slice<T extends string, startIndex extends number = 0, endIndex extends number = Length<T>> = T extends `${infer head}${infer rest}` ? startIndex extends 0 ? endIndex extends 0 ? '' : `${head}${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : `${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : ''; | ||
type Slice<T extends string, startIndex extends number = 0, endIndex extends number = Length<T>> = All<[ | ||
IsStringLiteral<T>, | ||
IsNumberLiteral<startIndex | endIndex> | ||
]> extends true ? T extends `${infer head}${infer rest}` ? startIndex extends 0 ? endIndex extends 0 ? '' : `${head}${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : `${Slice<rest, Math.Subtract<Math.GetPositiveIndex<T, startIndex>, 1>, Math.Subtract<Math.GetPositiveIndex<T, endIndex>, 1>>}` : '' : string; | ||
/** | ||
@@ -134,3 +162,3 @@ * A strongly-typed version of `String.prototype.slice`. | ||
*/ | ||
type EndsWith<T extends string, S extends string, P extends number = Length<T>> = Math.IsNegative<P> extends false ? P extends Length<T> ? S extends Slice<T, Math.Subtract<Length<T>, Length<S>>, Length<T>> ? true : false : EndsWith<Slice<T, 0, P>, S, Length<T>> : false; | ||
type EndsWith<T extends string, S extends string, P extends number = Length<T>> = All<[IsStringLiteral<T | S>, IsNumberLiteral<P>]> extends true ? Math.IsNegative<P> extends false ? P extends Length<T> ? S extends Slice<T, Math.Subtract<Length<T>, Length<S>>, Length<T>> ? true : false : EndsWith<Slice<T, 0, P>, S, Length<T>> : false : boolean; | ||
/** | ||
@@ -152,3 +180,3 @@ * A strongly-typed version of `String.prototype.endsWith`. | ||
*/ | ||
type Includes<T extends string, S extends string, P extends number = 0> = Math.IsNegative<P> extends false ? P extends 0 ? T extends `${string}${S}${string}` ? true : false : Includes<Slice<T, P>, S, 0> : Includes<T, S, 0>; | ||
type Includes<T extends string, S extends string, P extends number = 0> = string extends T | S ? boolean : Math.IsNegative<P> extends false ? P extends 0 ? T extends `${string}${S}${string}` ? true : false : Includes<Slice<T, P>, S, 0> : Includes<T, S, 0>; | ||
/** | ||
@@ -169,3 +197,6 @@ * A strongly-typed version of `String.prototype.includes`. | ||
*/ | ||
type Repeat<T extends string, times extends number = 0> = times extends 0 ? '' : Math.IsNegative<times> extends false ? Join<TupleOf<times, T>> : never; | ||
type Repeat<T extends string, times extends number = 0> = All<[ | ||
IsStringLiteral<T>, | ||
IsNumberLiteral<times> | ||
]> extends true ? times extends 0 ? '' : Math.IsNegative<times> extends false ? Join<TupleOf<times, T>> : never : string; | ||
/** | ||
@@ -186,3 +217,3 @@ * A strongly-typed version of `String.prototype.repeat`. | ||
*/ | ||
type PadEnd<T extends string, times extends number = 0, pad extends string = ' '> = Math.IsNegative<times> extends false ? Math.Subtract<times, Length<T>> extends infer missing extends number ? `${T}${Slice<Repeat<pad, missing>, 0, missing>}` : never : T; | ||
type PadEnd<T extends string, times extends number = 0, pad extends string = ' '> = All<[IsStringLiteral<T | pad>, IsNumberLiteral<times>]> extends true ? Math.IsNegative<times> extends false ? Math.Subtract<times, Length<T>> extends infer missing extends number ? `${T}${Slice<Repeat<pad, missing>, 0, missing>}` : never : T : string; | ||
/** | ||
@@ -204,3 +235,3 @@ * A strongly-typed version of `String.prototype.padEnd`. | ||
*/ | ||
type PadStart<T extends string, times extends number = 0, pad extends string = ' '> = Math.IsNegative<times> extends false ? Math.Subtract<times, Length<T>> extends infer missing extends number ? `${Slice<Repeat<pad, missing>, 0, missing>}${T}` : never : T; | ||
type PadStart<T extends string, times extends number = 0, pad extends string = ' '> = All<[IsStringLiteral<T | pad>, IsNumberLiteral<times>]> extends true ? Math.IsNegative<times> extends false ? Math.Subtract<times, Length<T>> extends infer missing extends number ? `${Slice<Repeat<pad, missing>, 0, missing>}${T}` : never : T : string; | ||
/** | ||
@@ -222,3 +253,3 @@ * A strongly-typed version of `String.prototype.padStart`. | ||
*/ | ||
type Replace<sentence extends string, lookup extends string | RegExp, replacement extends string = ''> = lookup extends string ? sentence extends `${infer rest}${lookup}${infer rest2}` ? `${rest}${replacement}${rest2}` : sentence : string; | ||
type Replace<sentence extends string, lookup extends string | RegExp, replacement extends string = ''> = lookup extends string ? IsStringLiteral<lookup | sentence | replacement> extends true ? sentence extends `${infer rest}${lookup}${infer rest2}` ? `${rest}${replacement}${rest2}` : sentence : string : string; | ||
/** | ||
@@ -240,3 +271,3 @@ * A strongly-typed version of `String.prototype.replace`. | ||
*/ | ||
type ReplaceAll<sentence extends string, lookup extends string | RegExp, replacement extends string = ''> = lookup extends string ? sentence extends `${infer rest}${lookup}${infer rest2}` ? `${rest}${replacement}${ReplaceAll<rest2, lookup, replacement>}` : sentence : string; | ||
type ReplaceAll<sentence extends string, lookup extends string | RegExp, replacement extends string = ''> = lookup extends string ? IsStringLiteral<lookup | sentence | replacement> extends true ? sentence extends `${infer rest}${lookup}${infer rest2}` ? `${rest}${replacement}${ReplaceAll<rest2, lookup, replacement>}` : sentence : string : string; | ||
/** | ||
@@ -258,3 +289,3 @@ * A strongly-typed version of `String.prototype.replaceAll`. | ||
*/ | ||
type StartsWith<T extends string, S extends string, P extends number = 0> = Math.IsNegative<P> extends false ? P extends 0 ? T extends `${S}${string}` ? true : false : StartsWith<Slice<T, P>, S, 0> : StartsWith<T, S, 0>; | ||
type StartsWith<T extends string, S extends string, P extends number = 0> = All<[IsStringLiteral<T | S>, IsNumberLiteral<P>]> extends true ? Math.IsNegative<P> extends false ? P extends 0 ? T extends `${S}${string}` ? true : false : StartsWith<Slice<T, P>, S, 0> : StartsWith<T, S, 0> : boolean; | ||
/** | ||
@@ -274,3 +305,3 @@ * A strongly-typed version of `String.prototype.startsWith`. | ||
*/ | ||
type TrimStart<T extends string> = T extends ` ${infer rest}` ? TrimStart<rest> : T; | ||
type TrimStart<T extends string> = IsStringLiteral<T> extends true ? T extends ` ${infer rest}` ? TrimStart<rest> : T : string; | ||
/** | ||
@@ -288,3 +319,3 @@ * A strongly-typed version of `String.prototype.trimStart`. | ||
*/ | ||
type TrimEnd<T extends string> = T extends `${infer rest} ` ? TrimEnd<rest> : T; | ||
type TrimEnd<T extends string> = IsStringLiteral<T> extends true ? T extends `${infer rest} ` ? TrimEnd<rest> : T : string; | ||
/** | ||
@@ -344,3 +375,3 @@ * A strongly-typed version of `String.prototype.trimEnd`. | ||
*/ | ||
type Truncate<T extends string, Size extends number, Omission extends string = '...'> = Math.IsNegative<Size> extends true ? Omission : Math.Subtract<Length<T>, Size> extends 0 ? T : Join<[Slice<T, 0, Math.Subtract<Size, Length<Omission>>>, Omission]>; | ||
type Truncate<T extends string, Size extends number, Omission extends string = '...'> = All<[IsStringLiteral<T | Omission>, IsNumberLiteral<Size>]> extends true ? Math.IsNegative<Size> extends true ? Omission : Math.Subtract<Length<T>, Size> extends 0 ? T : Join<[Slice<T, 0, Math.Subtract<Size, Length<Omission>>>, Omission]> : string; | ||
/** | ||
@@ -363,3 +394,3 @@ * A strongly typed function to truncate a string if it's longer than the given maximum string length. | ||
*/ | ||
type IsSeparator<T extends string> = T extends Separator ? true : false; | ||
type IsSeparator<T extends string> = IsStringLiteral<T> extends true ? T extends Separator ? true : false : boolean; | ||
@@ -371,11 +402,11 @@ type UpperChars = 'A' | 'B' | 'C' | 'D' | 'E' | 'F' | 'G' | 'H' | 'I' | 'J' | 'K' | 'L' | 'M' | 'N' | 'O' | 'P' | 'Q' | 'R' | 'S' | 'T' | 'U' | 'V' | 'W' | 'X' | 'Y' | 'Z'; | ||
*/ | ||
type IsUpper<T extends string> = T extends UpperChars ? true : false; | ||
type IsUpper<T extends string> = IsStringLiteral<T> extends true ? T extends UpperChars ? true : false : boolean; | ||
/** | ||
* Checks if the given character is a lower case letter. | ||
*/ | ||
type IsLower<T extends string> = T extends LowerChars ? true : false; | ||
type IsLower<T extends string> = IsStringLiteral<T> extends true ? T extends LowerChars ? true : false : boolean; | ||
/** | ||
* Checks if the given character is a letter. | ||
*/ | ||
type IsLetter<T extends string> = IsUpper<T> extends true ? true : IsLower<T> extends true ? true : false; | ||
type IsLetter<T extends string> = IsStringLiteral<T> extends true ? T extends LowerChars | UpperChars ? true : false : boolean; | ||
@@ -386,3 +417,3 @@ type Digit = '0' | '1' | '2' | '3' | '4' | '5' | '6' | '7' | '8' | '9'; | ||
*/ | ||
type IsDigit<T extends string> = T extends Digit ? true : false; | ||
type IsDigit<T extends string> = IsStringLiteral<T> extends true ? T extends Digit ? true : false : boolean; | ||
@@ -393,3 +424,3 @@ /** | ||
*/ | ||
type IsSpecial<T extends string> = IsLetter<T> extends true ? false : IsDigit<T> extends true ? false : IsSeparator<T> extends true ? false : true; | ||
type IsSpecial<T extends string> = IsStringLiteral<T> extends true ? IsLetter<T> extends true ? false : IsDigit<T> extends true ? false : IsSeparator<T> extends true ? false : true : boolean; | ||
@@ -402,3 +433,3 @@ /** | ||
*/ | ||
type Words<sentence extends string, word extends string = '', prev extends string = ''> = string extends sentence ? string[] : sentence extends `${infer curr}${infer rest}` ? IsSeparator<curr> extends true ? Reject<[word, ...Words<rest>], ''> : prev extends '' ? Reject<Words<rest, curr, curr>, ''> : [false, true] extends [IsDigit<prev>, IsDigit<curr>] ? [ | ||
type Words<sentence extends string, word extends string = '', prev extends string = ''> = IsStringLiteral<sentence | word | prev> extends true ? sentence extends `${infer curr}${infer rest}` ? IsSeparator<curr> extends true ? Reject<[word, ...Words<rest>], ''> : prev extends '' ? Reject<Words<rest, curr, curr>, ''> : [false, true] extends [IsDigit<prev>, IsDigit<curr>] ? [ | ||
word, | ||
@@ -421,3 +452,3 @@ ...Words<rest, curr, curr> | ||
...Words<rest, `${prev}${curr}`, curr> | ||
] : Reject<Words<rest, `${word}${curr}`, curr>, ''> : Reject<[word], ''>; | ||
] : Reject<Words<rest, `${word}${curr}`, curr>, ''> : Reject<[word], ''> : string[]; | ||
/** | ||
@@ -786,2 +817,2 @@ * A strongly typed function to extract the words from a sentence. | ||
export { CamelCase, CamelKeys, CharAt, Concat, ConstantCase, ConstantKeys, DeepCamelKeys, DeepConstantKeys, DeepDelimiterKeys, DeepKebabKeys, DeepPascalKeys, DeepSnakeKeys, DelimiterCase, DelimiterKeys, Digit, EndsWith, Includes, IsDigit, IsLetter, IsLower, IsSeparator, IsSpecial, IsUpper, Join, KebabCase, KebabKeys, Length, PadEnd, PadStart, PascalCase, PascalKeys, Repeat, Replace, ReplaceAll, Reverse, Separator, Slice, SnakeCase, SnakeKeys, Split, StartsWith, TitleCase, Trim, TrimEnd, TrimStart, Truncate, Words, camelCase, camelKeys, capitalize, charAt, concat, constantCase, constantKeys, deepCamelKeys, deepConstantKeys, deepDelimiterKeys, deepKebabKeys, deepPascalKeys, deepSnakeKeys, delimiterCase, delimiterKeys, endsWith, includes, join, kebabCase, kebabKeys, length, lowerCase, padEnd, padStart, pascalCase, pascalKeys, repeat, replace, replaceAll, reverse, slice, snakeCase, snakeKeys, split, startsWith, titleCase, toCamelCase, toConstantCase, toDelimiterCase, toKebabCase, toLowerCase, toPascalCase, toSnakeCase, toTitleCase, toUpperCase, trim, trimEnd, trimStart, truncate, uncapitalize, upperCase, words }; | ||
export { CamelCase, CamelKeys, CharAt, Concat, ConstantCase, ConstantKeys, DeepCamelKeys, DeepConstantKeys, DeepDelimiterKeys, DeepKebabKeys, DeepPascalKeys, DeepSnakeKeys, DelimiterCase, DelimiterKeys, EndsWith, Includes, IsDigit, IsLetter, IsLower, IsSeparator, IsSpecial, IsUpper, Join, KebabCase, KebabKeys, Length, PadEnd, PadStart, PascalCase, PascalKeys, Repeat, Replace, ReplaceAll, Reverse, Slice, SnakeCase, SnakeKeys, Split, StartsWith, TitleCase, Trim, TrimEnd, TrimStart, Truncate, Words, camelCase, camelKeys, capitalize, charAt, concat, constantCase, constantKeys, deepCamelKeys, deepConstantKeys, deepDelimiterKeys, deepKebabKeys, deepPascalKeys, deepSnakeKeys, delimiterCase, delimiterKeys, endsWith, includes, join, kebabCase, kebabKeys, length, lowerCase, padEnd, padStart, pascalCase, pascalKeys, repeat, replace, replaceAll, reverse, slice, snakeCase, snakeKeys, split, startsWith, titleCase, toCamelCase, toConstantCase, toDelimiterCase, toKebabCase, toLowerCase, toPascalCase, toSnakeCase, toTitleCase, toUpperCase, trim, trimEnd, trimStart, truncate, uncapitalize, upperCase, words }; |
@@ -77,12 +77,2 @@ 'use strict'; | ||
// src/native/to-lower-case.ts | ||
function toLowerCase(str) { | ||
return str.toLowerCase(); | ||
} | ||
// src/native/to-upper-case.ts | ||
function toUpperCase(str) { | ||
return str.toUpperCase(); | ||
} | ||
// src/native/trim-start.ts | ||
@@ -103,2 +93,12 @@ function trimStart(str) { | ||
// src/native/to-lower-case.ts | ||
function toLowerCase(str) { | ||
return str.toLowerCase(); | ||
} | ||
// src/native/to-upper-case.ts | ||
function toUpperCase(str) { | ||
return str.toUpperCase(); | ||
} | ||
// src/utils/reverse.ts | ||
@@ -115,3 +115,6 @@ function reverse(str) { | ||
return sentence; | ||
return join([sentence.slice(0, length2 - omission.length), omission]); | ||
return join([ | ||
sentence.slice(0, length2 - omission.length), | ||
omission | ||
]); | ||
} | ||
@@ -146,3 +149,3 @@ | ||
// src/utils/capitalize.ts | ||
// src/utils/word-case/capitalize.ts | ||
function capitalize(str) { | ||
@@ -152,13 +155,2 @@ return join([toUpperCase(charAt(str, 0)), slice(str, 1)]); | ||
// src/utils/word-case/delimiter-case.ts | ||
function delimiterCase(str, delimiter) { | ||
return join(words(str), delimiter); | ||
} | ||
var toDelimiterCase = delimiterCase; | ||
// src/utils/word-case/lower-case.ts | ||
function lowerCase(str) { | ||
return toLowerCase(delimiterCase(str, " ")); | ||
} | ||
// src/internal/internals.ts | ||
@@ -178,3 +170,3 @@ function typeOf(t) { | ||
// src/utils/uncapitalize.ts | ||
// src/utils/word-case/uncapitalize.ts | ||
function uncapitalize(str) { | ||
@@ -190,2 +182,8 @@ return join([toLowerCase(charAt(str, 0)), slice(str, 1)]); | ||
// src/utils/word-case/delimiter-case.ts | ||
function delimiterCase(str, delimiter) { | ||
return join(words(str), delimiter); | ||
} | ||
var toDelimiterCase = delimiterCase; | ||
// src/utils/word-case/constant-case.ts | ||
@@ -215,2 +213,7 @@ function constantCase(str) { | ||
// src/utils/word-case/lower-case.ts | ||
function lowerCase(str) { | ||
return toLowerCase(delimiterCase(str, " ")); | ||
} | ||
// src/utils/word-case/upper-case.ts | ||
@@ -217,0 +220,0 @@ function upperCase(str) { |
{ | ||
"name": "string-ts", | ||
"version": "1.3.2", | ||
"version": "1.3.3", | ||
"description": "Strongly-typed string functions.", | ||
@@ -5,0 +5,0 @@ "main": "./dist/index.js", |
@@ -0,1 +1,5 @@ | ||
<p align="center"> | ||
<img alt="StringTs Banner" src="./docs/string-ts-banner.png" width="800" /> | ||
</p> | ||
[](https://www.npmjs.org/package/string-ts) | ||
@@ -6,6 +10,4 @@  | ||
# string-ts | ||
## Strongly-typed string functions for all! | ||
Strongly-typed string functions for all! | ||
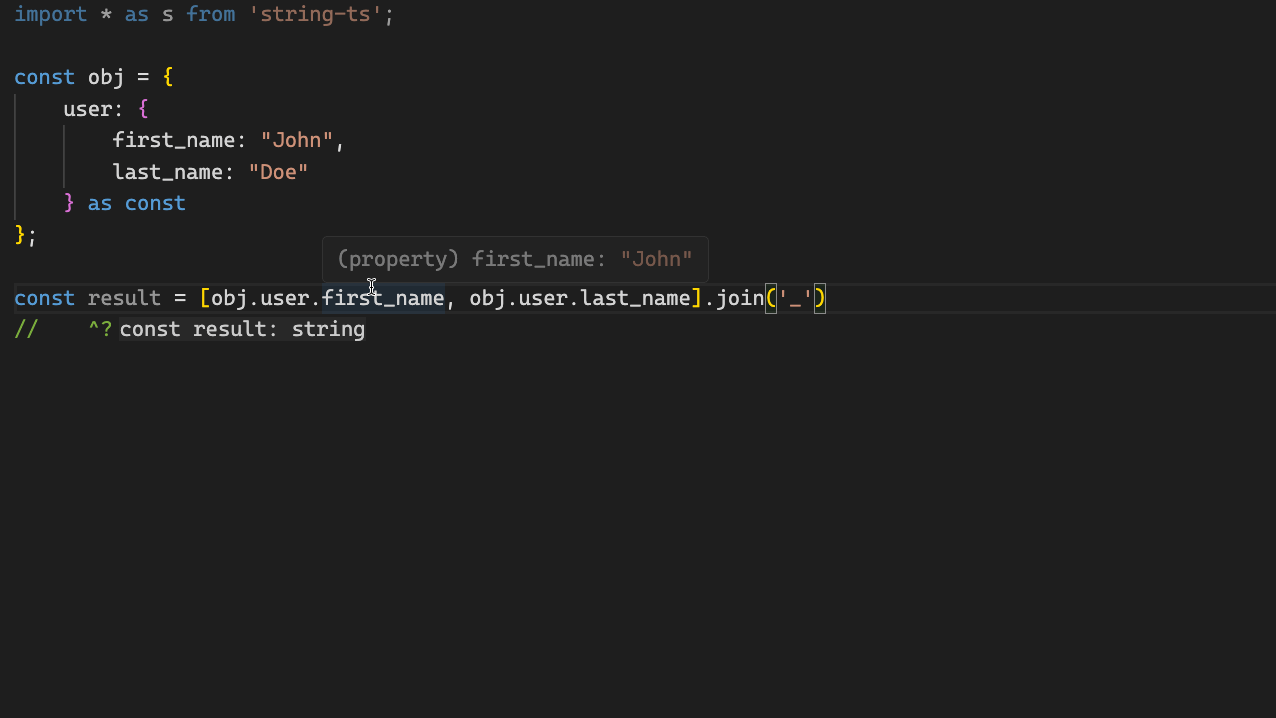 | ||
@@ -42,2 +44,4 @@ | ||
[I still don't get the purpose of this library 🤔](#%EF%B8%8F-interview) | ||
### In-depth example | ||
@@ -928,2 +932,8 @@ | ||
## 🎙️ Interview | ||
For a deeper dive into the code, reasoning, and how this library came to be, check out this interview with the author of StringTs on the Michigan TypeScript Meetup. | ||
[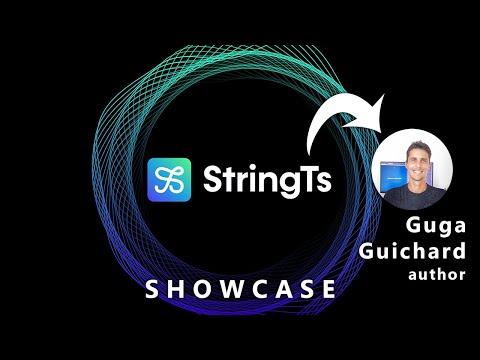](https://www.youtube.com/watch?v=dOXpkAmmahw) | ||
## 🐝 Contributors | ||
@@ -956,4 +966,8 @@ | ||
StringTs logo by [NUMI](https://github.com/numi-hq/open-design): | ||
[<img src="https://raw.githubusercontent.com/numi-hq/open-design/main/assets/numi-lockup.png" alt="NUMI Logo" style="width: 200px;"/>](https://numi.tech/?ref=string-ts) | ||
## 🫶 Acknowledgements | ||
This library got a lot of inspiration from libraries such as [lodash](https://github.com/lodash/lodash), [ts-reset](https://github.com/total-typescript/ts-reset), [type-fest](https://github.com/sindresorhus/type-fest), [HOTScript](https://github.com/gvergnaud/hotscript), and many others. |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
119668
7
1308
970
0