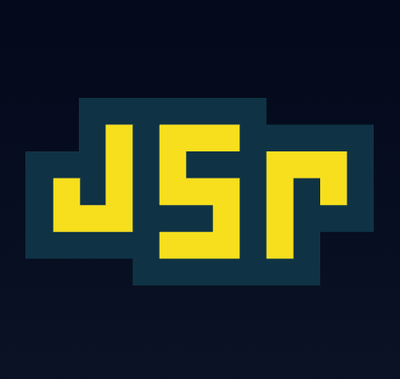
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The strtok3 npm package is a streaming tokenizer for Buffer and string inputs in Node.js. It allows developers to parse through binary data or strings efficiently by defining tokenizers that can extract pieces of data sequentially. This is particularly useful for reading binary files or network streams where data structures are defined in terms of sequences or patterns of bytes.
Tokenizing fixed-length binary data
This feature allows for reading fixed-length binary data from a file. The example demonstrates reading a 32-bit unsigned integer from the beginning of a file.
const strtok3 = require('strtok3');
const { token } = require('strtok3/core');
async function parseBinaryFile(filePath) {
const tokenizer = await strtok3.fromFile(filePath);
const header = await tokenizer.readToken(token.UINT32_LE);
console.log('Header:', header);
}
Tokenizing a stream of data
This feature is used for tokenizing data directly from a stream. The example shows how to read a size as a 32-bit unsigned integer and then read a buffer of that size from the stream.
const strtok3 = require('strtok3');
const { token } = require('strtok3/core');
const fs = require('fs');
const stream = fs.createReadStream('path/to/file');
const tokenizer = strtok3.fromStream(stream);
async function readData() {
const size = await tokenizer.readToken(token.UINT32_LE);
const data = await tokenizer.readToken(new token.BufferType(size));
console.log('Data:', data.toString());
}
binary-parser is a package for building efficient binary data parsers. It is similar to strtok3 in that it helps parse binary data, but it uses a declarative approach to define the structure of the binary data instead of the imperative approach used by strtok3.
A promise based streaming tokenizer for NodeJS. This node module is a successor of strtok2.
The strtok3
contains one class, ReadStreamTokenizer
, which is constructed with a
a stream.Readable.
The ReadStreamTokenizer
has one method readToken
which takes a token as an argument
and returns a Promise
resolving the decoded token value.
The token is basically a description what to read form the stream. A basic set of token types can be found here: token-types.
Below is an example of parsing the the first byte from a readable stream as an unsigned-integer:
import * as strtok3 from "strtok3";
import * as stream from "stream";
import * as Token from "token-types";
let readableStream: stream.Readable;
// Assign readable
strtok3.fromStream(readableStream).then((tokenizer) => {
return tokenizer.readToken<number>(Token.UINT8).then((myUint8Number) => {
console.log("My number: %s", myUint8Number);
});
})
var strtok3 = require('strtok3');
var Token = require('token-types');
var readableStream;
// Assign readable
strtok3.fromStream(readableStream).then( function(streamTokenizer) {
return streamTokenizer.readToken(Token.UINT8).then( function(myUint8Number) {
console.log('My number: %s', myUint8Number);
});
})
The same can be done from a file:
import * as strtok3 from "strtok3";
import * as Token from "token-types";
strtok3.fromFile("somefile.bin").then((tokenizer) => {
return tokenizer.readToken<number>(Token.UINT8).then((myUint8Number) => {
console.log("My number: %s", myUint8Number);
});
})
var strtok3 = require('strtok3');
var Token = require('token-types');
strtok3.fromFile('somefile.bin').then( function(streamTokenizer) {
return streamTokenizer.readToken(Token.UINT8).then( function(myUint8Number) {
console.log('My number: %s', myUint8Number);
});
})
FAQs
A promise based streaming tokenizer
The npm package strtok3 receives a total of 4,197,945 weekly downloads. As such, strtok3 popularity was classified as popular.
We found that strtok3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.