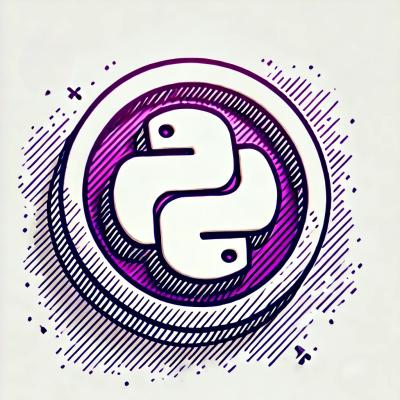
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
styled-jsx
Advanced tools
The styled-jsx npm package is a CSS-in-JS library that allows you to write encapsulated and scoped CSS to style your components in a React application. It is specifically designed to work with React and Next.js and provides a way to include styles directly within JavaScript or TypeScript files.
Scoped Styles
This feature allows you to write CSS that is scoped to a component. The styles will not leak to other parts of the application.
<style jsx>{`p { color: red; }`}</style>
Global Styles
With styled-jsx, you can also define global styles that apply to the entire application, not just scoped to a single component.
<style jsx global>{`body { background: black; }`}</style>
Dynamic Styles
styled-jsx supports dynamic styles, allowing you to use JavaScript variables and expressions to determine the styles at runtime.
`<style jsx>{
`p { color: ${color}; }`
}</style>`
Preprocessing
You can use preprocessors with styled-jsx to include external stylesheets or use features like nesting or variables.
<style jsx>{`
@import 'styles/shared.css';
p { color: red; }
`}</style>
styled-components is another CSS-in-JS library that allows you to use template literals to write actual CSS code in your JavaScript files. It also handles scoping and supports dynamic styling. It differs from styled-jsx in its API and the way styles are applied to components.
Emotion is a performant and flexible CSS-in-JS library. It allows you to style applications quickly with string or object styles. It has a similar API to styled-components and includes features like composition, theming, and server-side rendering.
Linaria is a zero-runtime CSS-in-JS library that extracts CSS to separate files during the build process, rather than including styles in the JavaScript bundle. This can result in better performance compared to styled-jsx, which includes styles in the JS bundle.
Full, scoped and component-friendly CSS support for JSX (rendered on the server or the client).
Firstly, install the package:
$ npm install --save styled-jsx
Next, add styled-jsx/babel
to plugins
in your babel configuration:
{
"plugins": [
"styled-jsx/babel"
]
}
Now add <style jsx>
to your code and fill it with CSS:
export default () => (
<div>
<p>only this paragraph will get the style :)</p>
{ /* you can include <Component />s here that include
other <p>s that don't get unexpected styles! */ }
<style jsx>{`
p {
color: red;
}
`}</style>
</div>
)
<style scoped>
, but the styles get injected only once per componentThe example above transpiles to the following:
import _JSXStyle from 'styled-jsx/style'
export default () => (
<div data-jsx='cn2o3j'>
<p data-jsx='cn2o3j'>only this paragraph will get the style :)</p>
<_JSXStyle data-jsx='cn2o3j' css={`p[data-jsx=cn2o3j] {color: red;}`} />
</div>
)
Data attributes give us style encapsulation and _JSXStyle
is heavily optimized for:
Notice that the parent <div>
above also gets a data-jsx
atribute. We do this so that
you can target the "root" element, in the same manner that
:host
works with Shadow DOM.
If you want to target only the host, we suggest you use a class:
export default () => (
<div className="root">
<style jsx>{`
.root {
color: green;
}
`}</style>
</div>
)
To skip scoping entirely, you can make the global-ness of your styles explicit by adding global.
export default () => (
<div>
<style jsx global>{`
body {
background: red
}
`}</style>
</div>
)
The advantage of using this over <style>
is twofold: no need
to use dangerouslySetInnerHTML
to avoid escaping issues with CSS
and take advantage of styled-jsx
's de-duping system to avoid
the global styles being inserted multiple times.
Sometimes it's useful to skip prefixing. We support :global()
,
inspired by css-modules.
This is very useful in order to, for example, generate an unprefixed class that
you can pass to 3rd-party components. For example, to style
react-select
which supports passing a custom class via optionClassName
:
import Select from 'react-select'
export default () => (
<div>
<Select optionClassName="react-select" />
<style jsx>{`
/* "div" will be prefixed, but ".react-select" won't */
div :global(.react-select) {
color: red
}
`}</style>
</div>
)
className
togglingTo make a component's visual representation customizable from the outside world, there are two options. The first one is to pass properties that toggle class names.
const Button = (props) => (
<button className={ 'large' in props && 'large' }>
{ props.children }
<style jsx>{`
button {
padding: 20px;
background: #eee;
color: #999
}
.large {
padding: 50px
}
`}</style>
</button>
)
Then you would use this component as either <Button>Hi</Button>
or <Button large>Big</Button>
.
style
Imagine that you wanted to make the padding in the button above completely customizable. You can override the CSS you configure via inline-styles:
const Button = ({ padding, children }) => (
<button style={{ padding }}>
{ children }
<style jsx>{`
button {
padding: 20px;
background: #eee;
color: #999
}
`}</style>
</button>
)
In this example, the padding defaults to the one set in <style>
(20
), but the user can pass a custom one via <Button padding={30}>
.
It is possible to use constants like so:
import { colors, spacing } from '../theme'
import { invertColor } from '../theme/utils'
const Button = ({ children }) => (
<button>
{ children }
<style jsx>{`
button {
padding: ${ spacing.medium };
background: ${ colors.primary };
color: ${ invertColor(colors.primary) };
}
`}</style>
</button>
)
N.B. Only constants defined outside of the component scope are allowed here.
If you want to use or toggle dynamic values depending on the component state
or props
then we recommend to use one of the techniques from the Dynamic styles section
styled-jsx/server
The main export flushes your styles to an array of React.Element
:
import React from 'react'
import ReactDOM from 'react-dom/server'
import flush from 'styled-jsx/server'
import App from './app'
export default (req, res) => {
const app = ReactDOM.renderToString(<App />)
const styles = flush()
const html = ReactDOM.renderToStaticMarkup(<html>
<head>{ styles }</head>
<body>
<div id="root" dangerouslySetInnerHTML={{__html: app}} />
</body>
</html>)
res.end('<!doctype html>' + html)
}
We also expose flushToHTML
to return generated HTML:
import React from 'react'
import ReactDOM from 'react-dom/server'
import { flushToHTML } from 'styled-jsx/server'
import App from './app'
export default (req, res) => {
const app = ReactDOM.renderToString(<App />)
const styles = flushToHTML()
const html = `<!doctype html>
<html>
<head>${styles}</head>
<body>
<div id="root">${app}</div>
</body>
</html>`
res.end(html)
}
It's paramount that you use one of these two functions so that the generated styles can be diffed when the client loads and duplicate styles are avoided.
murmurhash2
(minimal and fast hashing) and an efficient style injection logic.FAQs
Full CSS support for JSX without compromises
The npm package styled-jsx receives a total of 5,188,336 weekly downloads. As such, styled-jsx popularity was classified as popular.
We found that styled-jsx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.