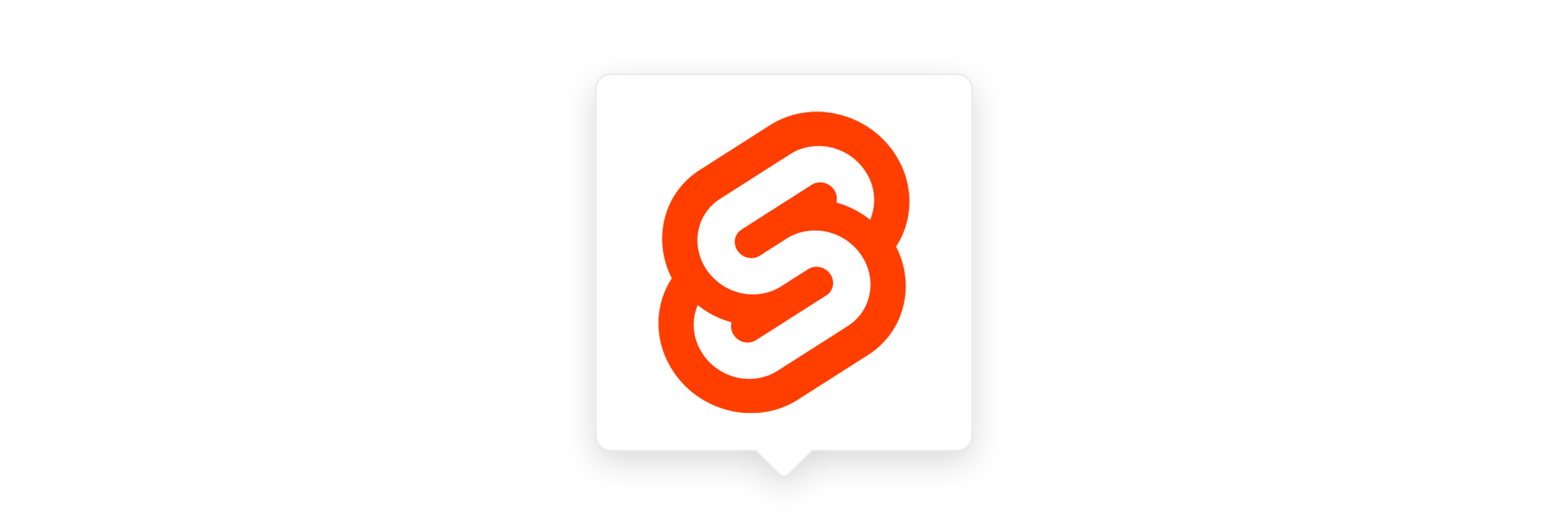
svelte-popperjs

Popper for Svelte with actions, no wrapper components or component bindings required!
Other Popper libraries for Svelte (including the official @popperjs/svelte
library) use a wrapper component that takes the required DOM elements as props. Not only does this require multiple bind:this
, you also have to pollute your script
tag with multiple DOM references.
We can do better with Svelte actions!
Installation
$ npm i -D svelte-popperjs
Since Svelte automatically bundles all required dependencies, you only need to install this package as a dev dependency with the -D
flag.
Usage
createPopperActions
returns a pair of actions to be used on the reference and popper elements.
The content action takes an options object for configuring the popper instance.
Example
A Svelte version of the standard tutorial.
styles not included*
<script>
import { createPopperActions } from 'svelte-popperjs';
const [popperRef, popperContent] = createPopperActions();
const popperOptions = {
modifiers: [
{ name: 'offset', options: { offset: [0, 8] } }
],
};
let showTooltip = false;
</script>
<button
use:popperRef
on:mouseenter={() => showTooltip = true}
on:mouseleave={() => showTooltip = false}
>
My button
</button>
{#if showTooltip}
<div id="tooltip" use:popperContent={popperOptions}>
My tooltip
<div id="arrow" data-popper-arrow />
</div>
{/if}
Popper Instance
If access is needed to the raw Popper instance created by the actions, you can reference the third element returned by createPopperActions
. The third element is a function that will return the current Popper instance used by the actions.
Using the raw Popper instance to manually recompute the popper's position.
<script>
import { createPopperActions } from 'svelte-popperjs';
const [popperRef, popperContent, getInstance] = createPopperActions();
async function refreshTooltip() {
const newState = await getInstance().update();
}
</script>