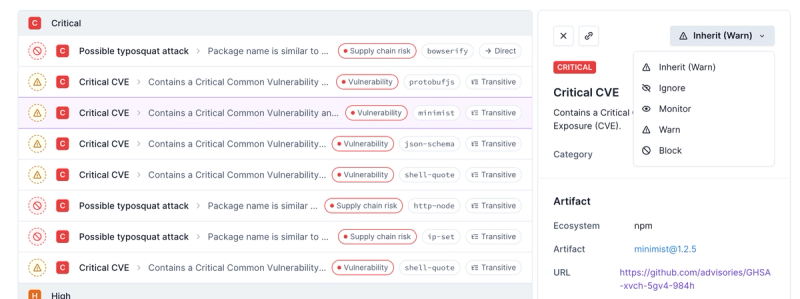
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
svg-pathdata
Advanced tools
Package description
The svg-pathdata npm package is a powerful tool for parsing, manipulating, and serializing SVG path data. It allows developers to easily work with SVG path strings, converting them into a more manageable format, modifying their properties, and converting them back into strings. This package is particularly useful for applications that need to dynamically generate or alter SVG graphics.
Parsing SVG Path Data
This feature allows you to parse a string containing SVG path data into a more manageable object format. This is useful for reading and manipulating individual commands within an SVG path.
"const {SVGPathData, SVGPathDataParser} = require('svg-pathdata');\nconst pathData = new SVGPathData('M10 10L90 90');"
Serializing SVG Path Data
After manipulating or generating SVG path data, this feature enables you to convert the path data back into a string format. This is essential for rendering the modified SVG graphics on the web.
"const {SVGPathData} = require('svg-pathdata');\nconst pathData = new SVGPathData('M10 10L90 90');\nconst serializedPath = pathData.encode();"
Transforming SVG Path Data
This feature provides a set of transformation functions (such as translate, rotate, scale) that can be applied to the path data. It's useful for dynamically altering the appearance of SVG graphics without manually recalculating path commands.
"const {SVGPathData, SVGPathDataTransformer} = require('svg-pathdata');\nconst pathData = new SVGPathData('M10 10L90 90').transform(SVGPathDataTransformer.translate(10, 20));"
Similar to svg-pathdata, svgpath provides tools for parsing and modifying SVG path data. It offers a fluent API for transforming paths (translate, rotate, scale, etc.). Compared to svg-pathdata, svgpath might be preferred for its chainable transformations, but it lacks some of the direct manipulation capabilities.
This package focuses on parsing SVG path data into a more readable and manipulable array format. While it provides a solid parsing capability, it does not offer the extensive manipulation and serialization features found in svg-pathdata, making it more suitable for applications that primarily need to interpret path data.
Changelog
v1.0.4 (2016/11/07 15:18 +00:00)
Readme
Manipulating SVG path data (path[d] attribute content) simply and efficiently.
This library is fully node based (based on current stream implementation) but you can also use it in modern browsers with the browserified build or in your own build using Browserify.
## Reading PathData
var pathData = new SVGPathData ('\
M 10 10 \
H 60 \
V 60 \
L 10 60 \
Z \
');
console.log(pathData.commands);
// {"commands":[{
// "type": SVGPathData.MOVE_TO,
// "relative": false,
// "x": 10, "y": 10
// },{
// "type": SVGPathData.HORIZ_LINE_TO,
// "relative": false,
// "x": 60
// },{
// "type": SVGPathData.VERT_LINE_TO,
// "relative":false,
// "y": 60
// },{
// "type": SVGPathData.LINE_TO,
// "relative": false,
// "x": 10,
// "y": 60
// },{
// "type": SVGPathData.CLOSE_PATH
// }
// ]}
## Reading streamed PathData
var parser = new SVGPathData.Parser();
parser.on('data', function(cmd) {
console.log(cmd);
});
parser.write(' ');
parser.write('M 10');
parser.write(' 10');
// {
// "type": SVGPathData.MOVE_TO,
// "relative": false,
// "x": 10, "y": 10
// }
parser.write('H 60');
// {
// "type": SVGPathData.HORIZ_LINE_TO,
// "relative": false,
// "x": 60
// }
parser.write('V');
parser.write('60');
// {
// "type": SVGPathData.VERT_LINE_TO,
// "relative": false,
// "y": 60
// }
parser.write('L 10 60 \
Z');
// {
// "type": SVGPathData.LINE_TO,
// "relative": false,
// "x": 10,
// "y": 60
// }
// {
// "type": SVGPathData.CLOSE_PATH
// }
parser.end();
## Outputting PathData
var pathData = new SVGPathData ('\
M 10 10 \
H 60 \
V 60 \
L 10 60 \
Z \
');
console.log(pathData.encode());
// "M10 10H60V60L10 60Z"
## Streaming PathData out
var encoder = new SVGPathData.Encoder();
encoder.setEncoding('utf8');
encode.on('data', function(str) {
console.log(str);
});
encoder.write({
"type": SVGPathData.MOVE_TO,
"relative": false,
"x": 10, "y": 10
});
// "M10 10"
encoder.write({
"type": SVGPathData.HORIZ_LINE_TO,
"relative": false,
"x": 60
});
// "H60"
encoder.write({
"type": SVGPathData.VERT_LINE_TO,
"relative": false,
"y": 60
});
// "V60"
encoder.write({
"type": SVGPathData.LINE_TO,
"relative": false,
"x": 10,
"y": 60
});
// "L10 60"
encoder.write({"type": SVGPathData.CLOSE_PATH});
// "Z"
encode.end();
## Transforming PathData This library was made to live decoding/transform/encoding SVG PathData. Here is an example of that kind of use.
console.log(
new SVGPathData ('\
m 10,10 \
h 60 \
v 60 \
l 10,60 \
z'
)
.toAbs()
.encode()
);
// "M10,10 H70 V70 L80,130 Z"
Here, we take SVGPathData from stdin and output it transformed to stdout.
// stdin to parser
process.stdin.pipe(new SVGPathData.Parser())
// parser to transformer to absolute
.pipe(new SVGPathData.Transformer(SVGPathData.Transformer.TO_ABS))
// transformer to encoder
.pipe(new SVGPathData.Encoder())
// encoder to stdout
.pipe(process.stdout);
You can find every supported transformations in this file of course, you can create yours by using this format:
function SET_X_TO(xValue) {
xValue = xValue || 10; // Provide default values or throw errors for options
function(command) {
command.x = xValue; // transform command objects and return them
return command;
};
};
// Synchronous usage
new SVGPathData('...')
.transform(SET_X_TO, 25)
.encode();
// Streaming usage
process.stdin.pipe(new SVGPathData.Parser())
.pipe(new SVGPathData.Transformer(SET_X_TO, 25))
.pipe(new SVGPathData.Encoder())
.pipe(process.stdout);
Clone this project, run:
npm install; npm test
Build:
npm build
FAQs
Manipulate SVG path data (path[d] attribute content) simply and efficiently.
The npm package svg-pathdata receives a total of 1,364,739 weekly downloads. As such, svg-pathdata popularity was classified as popular.
We found that svg-pathdata demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.