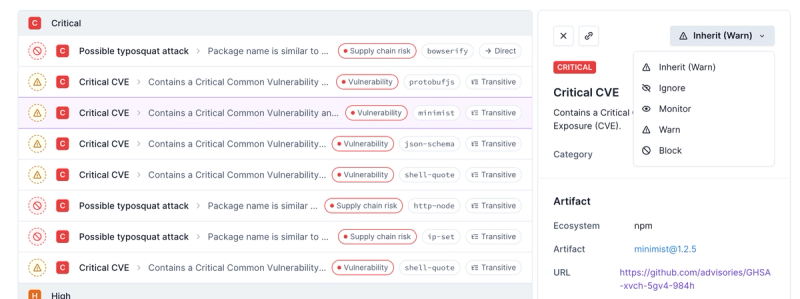
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
svgicons2svgfont
Advanced tools
Readme
Read a set of SVG icons and ouput a SVG font
svgicons2svgfont is a simple tool to merge multiple icons to an SVG font.
'rect', 'line', 'circle', 'ellipsis', 'polyline' and 'polygon' shapes will be converted to pathes. Multiple pathes will be merged.
Transform attributes support is currenly experimental, report issues if any.
You can test this library with the frontend generator.
You may want to convert fonts to icons, if so use svgfont2svgicons.
const SVGIcons2SVGFontStream = require('svgicons2svgfont');
const fs = require('fs');
const fontStream = new SVGIcons2SVGFontStream({
fontName: 'hello'
});
// Setting the font destination
fontStream.pipe(fs.createWriteStream('fonts/hello.svg'))
.on('finish',function() {
console.log('Font successfully created!')
})
.on('error',function(err) {
console.log(err);
});
// Writing glyphs
const glyph1 = fs.createReadStream('icons/icon1.svg');
glyph1.metadata = {
unicode: ['\uE001\uE002'],
name: 'icon1'
};
fontStream.write(glyph1);
// Multiple unicode values are possible
const glyph2 = fs.createReadStream('icons/icon1.svg');
glyph2.metadata = {
unicode: ['\uE002', '\uEA02'],
name: 'icon2'
};
fontStream.write(glyph2);
// Either ligatures are available
const glyph3 = fs.createReadStream('icons/icon1.svg');
glyph3.metadata = {
unicode: ['\uE001\uE002'],
name: 'icon1-icon2'
};
fontStream.write(glyph3);
// Do not forget to end the stream
fontStream.end();
All options are available except the log
one by using this pattern:
--{LOWER_CASE(optionName)}={optionValue}
.
svgicons2svgfont --fontname=hello -o font/destination/file.svg icons/directory/*.svg
Note that you won't be able to customize icon names or icons unicodes by
passing options but by using the following convention to name your icons files:
${icon.unicode}-${icon.name}.svg
where icon.unicode
is a comma separated
list of unicode strings (ex: 'uEA01,uE001,uE001uE002', note that the last
string is in fact a ligature).
There is a few more options for the CLI interface, you can list all of them:
svgicons2svgfont --help
# Usage: svgicons2svgfont [options] <icons ...>
#
# Options:
#
# -V, --version output the version number
# -v, --verbose tell me everything!
# -o, --output [/dev/stdout] file to write output to
# -f, --fontname [value] the font family name you want [iconfont]
# -i, --fontId [value] the font id you want [fontname]
# -st, --style [value] the font style you want
# -we, --weight [value] the font weight you want
# -w, --fixedWidth creates a monospace font of the width of the largest input icon
# -c, --centerhorizontally calculate the bounds of a glyph and center it horizontally
# -n, --normalize normalize icons by scaling them to the height of the highest icon
# -h, --height [value] the output font height [MAX(icons.height)] (icons will be scaled so the highest has this height)
# -r, --round [value] setup the SVG path rounding [10e12]
# -d, --descent [value] the font descent [0]
# -a, --ascent [value] the font ascent [height - descent]
# -s, --startunicode [value] the start unicode codepoint for unprefixed files [0xEA01]
# -a, --prependUnicode prefix files with their automatically allocated unicode code point
# -m, --metadata content of the metadata tag
# -h, --help output usage information
Type: String
Default value: 'iconfont'
The font family name you want.
Type: String
Default value: the options.fontName value
The font id you want.
Type: String
Default value: ''
The font style you want.
Type: String
Default value: ''
The font weight you want.
Type: Boolean
Default value: false
Creates a monospace font of the width of the largest input icon.
Type: Boolean
Default value: false
Calculate the bounds of a glyph and center it horizontally.
Type: Boolean
Default value: false
Normalize icons by scaling them to the height of the highest icon.
Type: Number
Default value: MAX(icons.height)
The outputted font height (defaults to the height of the highest input icon).
Type: Number
Default value: 10e12
Setup SVG path rounding.
Type: Number
Default value: 0
The font descent. It is usefull to fix the font baseline yourself.
Warning: The descent is a positive value!
Type: Number
Default value: fontHeight - descent
The font ascent. Use this options only if you know what you're doing. A suitable value for this is computed for you.
Type: String
Default value: undefined
The font metadata. You can set any character data in but it is the be suited place for a copyright mention.
Type: Function
Default value: console.log
Allows you to provide your own logging function. Set to function(){}
to
disable logging.
grunt-svgicons2svgfont and grunt-webfont.
Try gulp-iconfont and gulp-svgicons2svgfont.
Use stylus-iconfont.
You can combine this plugin's CLI interface with svg2ttf, ttf2eot, ttf2woff and ttf2woff2. You can also use webfonts-generator.
Feel free to push your code if you agree with publishing under the MIT license.
FAQs
Read a set of SVG icons and ouput a SVG font
The npm package svgicons2svgfont receives a total of 136,061 weekly downloads. As such, svgicons2svgfont popularity was classified as popular.
We found that svgicons2svgfont demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.