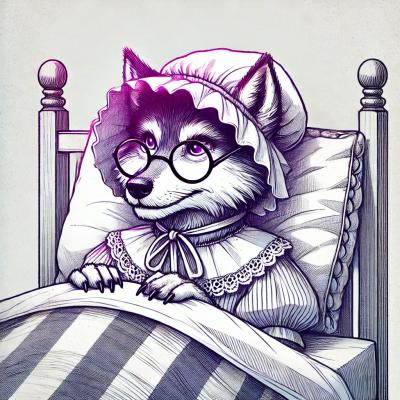
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
swagger-snippet
Advanced tools
Generates code snippets for given Swagger / Open API Specification files
Generates code snippets for given Swagger / Open API specification files.
This package takes as input a Swagger 2.0 / Open API specification. Optionally, validates that specification. Translates the specification into an HTTP Archive 1.2 request object. Uses the HTTP Snippet library to generate code snippets for every API endpoint defined in the specification in various languages & tools (cURL
, Node
, Python
, Ruby
, Java
, Go
, C#
...).
npm i --save swagger-snippet
let SwaggerSnippet = require('swagger-snippet')
// define input:
var swagger = ... // a Swagger / Open API specification
var targets = ['node_unirest', 'c'] // array of targets for code snippets. See list below...
var validateSpec = true // whether to validate the specification
SwaggerSnippet(swagger, targets, validateSpec, function(err, data) {
if (err) return
console.log(data) // prints array of snippets, see "Output" below.
})
The output of this library is an array, where every entry corresponds to an endpoint (URL path + HTTP method) in the given specification. Every entry contains the method
, url
, a human-readable description
, and the corresponding resource
- all of these values stem from the specification. In addition, within the snippets
list, an object containing a code snippet for every chosen target is provided.
For example:
[
...
{
"method": "GET",
"url": "https://api.instagram.com/v1/users/{user-id}/relationship",
"description": "Get information about a relationship to another user.",
"resource": "relationship",
"snippets": [
{
"id": "node",
"title": "Node + Native",
"content": "var http = require(\"https\");\n\nvar options = {\n \"method\": \"GET\",\n \"hostname\": \"api.instagram.com\",\n \"port\": null,\n \"path\": \"/v1/users/%7Buser-id%7D/relationship\",\n \"headers\": {\n \"access_token\": \"REPLACE_KEY_VALUE\"\n }\n};\n\nvar req = http.request(options, function (res) {\n var chunks = [];\n\n res.on(\"data\", function (chunk) {\n chunks.push(chunk);\n });\n\n res.on(\"end\", function () {\n var body = Buffer.concat(chunks);\n console.log(body.toString());\n });\n});\n\nreq.end();"
}
]
}
...
]
Currently, swagger-snippet supports the following targets (depending on the HTTP Snippet library):
c_libcurl
(default)csharp_restsharp
(default)go_native
(default)java_okhttp
java_unirest
(default)javascript_jquery
javascript_xhr
(default)node_native
(default)node_request
node_unirest
objc_nsurlsession
(default)ocaml_cohttp
(default)php_curl
(default)php_http1
php_http2
python_python3
(default)python_requests
ruby_native
(default)shell_curl
(default)shell_httpie
shell_wget
swift_nsurlsession
(default)If only the language is provided (e.g., c
), the default library will be selected.
License: MIT
FAQs
Generates code snippets for given Swagger / Open API Specification files
The npm package swagger-snippet receives a total of 36 weekly downloads. As such, swagger-snippet popularity was classified as not popular.
We found that swagger-snippet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.