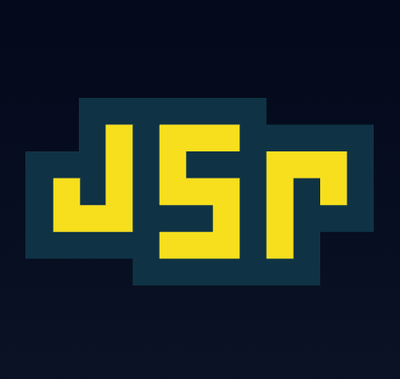
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The swr npm package is a React Hooks library for remote data fetching. The name 'SWR' is derived from stale-while-revalidate, a HTTP cache invalidation strategy popularized by HTTP RFC 5861. SWR is a strategy to first return the data from cache (stale), then send the fetch request (revalidate), and finally come with the up-to-date data.
Data Fetching
SWR provides a hook called useSWR for data fetching. You can pass a key and a fetcher function, and it will return the data, error, and other useful values for handling the UI state.
import useSWR from 'swr'
function Profile() {
const { data, error } = useSWR('/api/user', fetcher)
if (error) return <div>Failed to load</div>
if (!data) return <div>Loading...</div>
return <div>Hello, {data.name}!</div>
}
Automatic Revalidation
SWR automatically revalidates data when a user focuses on the window or when the network is reconnected. It can also be configured to revalidate data at a fixed interval.
import useSWR from 'swr'
function Profile() {
const { data } = useSWR('/api/user', fetcher, {
refreshInterval: 3000
})
// data will be revalidated every 3 seconds
return <div>{data.name}</div>
}
Local Mutation
SWR allows you to mutate the local data immediately and revalidate it in the background. This provides an optimistic UI update experience.
import useSWR, { mutate } from 'swr'
function updateUsername(name) {
mutate('/api/user', { ...data, name }, false)
fetch('/api/user', {
method: 'POST',
body: JSON.stringify({ name })
}).then(() => {
mutate('/api/user')
})
}
React Query is another library for fetching, caching, and updating data in React applications. It provides more advanced features like query cancellation, background fetching, and even pagination helpers. React Query is often compared to SWR for its similar use cases but offers a different API and additional features.
Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. It is more complex and powerful than SWR, designed specifically for GraphQL, and provides features like caching, optimistic UI, and subscription support.
Axios is a promise-based HTTP client for the browser and Node.js. While it is not a hook-based data fetching library like SWR, it is often used for making HTTP requests in React applications. Developers would use Axios for fetching data and then manage the caching and state themselves or with additional libraries.
This repo is a demo on how to setup selenium tests with it's webdriverjs library from scratch. The example includes the following:
There are many examples about how to write a few lines of code to get selenium webdriverjs working. Usually they show you how to get the code, create a driver, go to some website and grab the title to prove it works. However, in real applications we want to have something more organized and scalable, that's when the selenium based test frameworks come to help.
Despite the good parts, the problem with using these frameworks, say webdriverio or nightwatch, is they can be too complicated or overkill for a specific projects. They also have a lot dependencies that may not be well maintained, which could cause unstable test outcomes. More and more people went from using the test frameworks, to finally built one that best fit their requirements.
I want to show you it's actually not that hard to create a test runner that works well, with just a few dependencies besides selenium-webdriver, and highly customizable to fit your own needs.
FAQs
React Hooks library for remote data fetching
The npm package swr receives a total of 1,842,139 weekly downloads. As such, swr popularity was classified as popular.
We found that swr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.