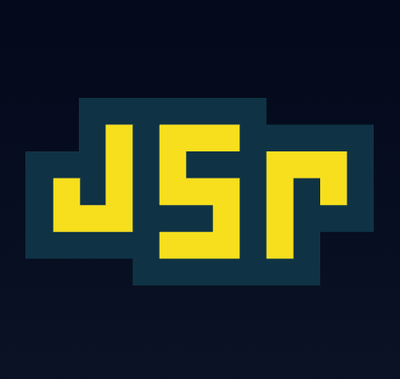
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
symbol-observable
Advanced tools
The symbol-observable package is primarily used to polyfill the Observable symbol, which is a proposed addition to the ECMAScript standard. It allows JavaScript objects to be observable, meaning that other objects can subscribe to them and react to changes. This is particularly useful in reactive programming models and libraries, such as RxJS, where data streams and their transformations are a core concept.
Polyfill for Observable Symbol
This code demonstrates how to use the symbol-observable package to make an object observable. It defines a simple observable that emits 'hi' every second. An observer subscribes to this observable to log the emitted values. This showcases how symbol-observable can be used to implement the observer pattern in JavaScript.
"use strict";
const symbolObservable = require('symbol-observable').default;
console.log(typeof symbolObservable); // 'symbol' in environments with native Symbol support
const obj = {};
obj[symbolObservable] = function () {
return {
subscribe(observer) {
const intervalID = setInterval(() => observer.next('hi'), 1000);
return {
unsubscribe() {
clearInterval(intervalID);
}
};
}
};
};
const observable = obj[symbolObservable]();
const subscription = observable.subscribe({
next(val) { console.log(val); },
complete() { console.log('Done'); }
});
// Later:
// subscription.unsubscribe();
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. Unlike symbol-observable, which provides the basic polyfill for the Observable symbol, RxJS offers a comprehensive suite of operators for transforming, combining, and managing asynchronous streams of data.
Zen Observable is a lightweight implementation of Observables for JavaScript. It provides an Observable class that can be used directly, without needing a polyfill. Compared to symbol-observable, Zen Observable offers more out-of-the-box functionality, including a full implementation of the Observable spec, rather than just providing the symbol.
This will polyfill Symbol.observable
if Symbol
exists, but will not polyfill Symbol
if it doesn't exist. Meant to be used as a "ponyfill", meaning you're meant to use the module's exported symbol value as described below. This is all done to ensure that everyone is using the same version of the symbol (or string depending on the environment), as per the nature of symbols in JavaScript.
$ npm install --save symbol-observable
const symbolObservable = require('symbol-observable').default;
console.log(symbolObservable);
//=> Symbol(observable)
import Symbol_observable from 'symbol-observable';
console.log(Symbol_observable);
//=> Symbol(observable)
You can do something like what you see below to make any object "observable" by libraries like RxJS, XStream and Most.js.
Things to know:
next
, error
or complete
on your observer after error
or complete
was called.next
, error
or complete
after unsubscribe
is called on the returned object.import Symbol_observable from 'symbol-observable';
someObject[Symbol_observable] = () => {
return {
subscribe(observer) {
const handler = e => observer.next(e);
someObject.addEventListener('data', handler);
return {
unsubscribe() {
someObject.removeEventListener('data', handler);
}
}
},
[Symbol_observable]() { return this }
}
}
Often, it's not very hard, but it can get tricky in some cases.
MIT © Sindre Sorhus and Ben Lesh
FAQs
Symbol.observable ponyfill
The npm package symbol-observable receives a total of 11,509,042 weekly downloads. As such, symbol-observable popularity was classified as popular.
We found that symbol-observable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.