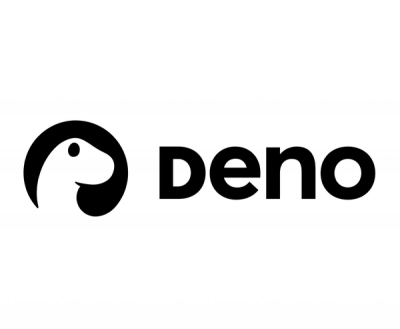
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
symtable.js
Advanced tools
An imperative symbol table library in Javascript
npm install symtable.js
var Symtable = require('symtable.js');
var globalIds = new Map();
globalIds.set('window', 1);
globalIds.set('document', 2);
// Initialize a new symbol table with a map of symbols in the global scope.
var S = new Symtable(globalIds);
// Start a new nested scope
S.enterScope();
// Add a symbol to the table
S.add('a', '3');
S.add('b', 4);
// Find the value of a Symbol
S.find('a'); // 3
S.find('k'); // null
// Check the current scope for a given symbol
S.checkScope('a'); // true
S.checkScope('window'); // false. Since this exists in the global scope and the current scope.
// Exit the current scope
S.exitScope();
enterScope()
- Start a new nested scope.
add(x, y)
- Add a new symbol x
with associated data y
.
find(x)
- Finds current x
in the whole symbol table using the most closely nested rule. Returns null
otherwise.
checkScope(x)
- Returns true
if x
is defined in the current scope.
exitScope()
- Exit the current scope
FAQs
An imperative symbol table library in Javascript
The npm package symtable.js receives a total of 0 weekly downloads. As such, symtable.js popularity was classified as not popular.
We found that symtable.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.