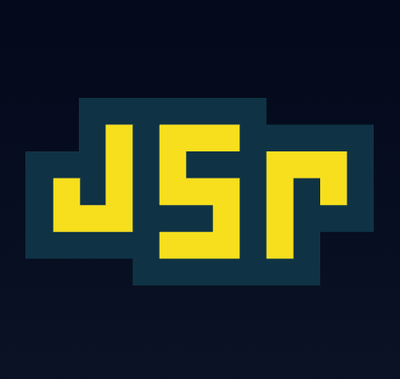
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
sync-request
Advanced tools
The sync-request npm package allows you to make synchronous HTTP requests in Node.js. This can be useful for scenarios where you need to ensure that a request completes before moving on to the next line of code, although it is generally recommended to use asynchronous requests to avoid blocking the event loop.
GET Request
This feature allows you to make a synchronous GET request to a specified URL. The response body can be accessed and logged.
const request = require('sync-request');
const res = request('GET', 'https://jsonplaceholder.typicode.com/posts/1');
console.log(res.getBody('utf8'));
POST Request
This feature allows you to make a synchronous POST request with a JSON payload. The response body can be accessed and logged.
const request = require('sync-request');
const res = request('POST', 'https://jsonplaceholder.typicode.com/posts', {
json: { title: 'foo', body: 'bar', userId: 1 }
});
console.log(res.getBody('utf8'));
Custom Headers
This feature allows you to make a synchronous GET request with custom headers. The response body can be accessed and logged.
const request = require('sync-request');
const res = request('GET', 'https://jsonplaceholder.typicode.com/posts/1', {
headers: {
'User-Agent': 'request'
}
});
console.log(res.getBody('utf8'));
Axios is a promise-based HTTP client for the browser and Node.js. Unlike sync-request, Axios is asynchronous and uses promises, making it more suitable for non-blocking operations.
Node-fetch is a lightweight module that brings `window.fetch` to Node.js. It is also asynchronous and uses promises, providing a more modern and flexible approach compared to sync-request.
Request is a simplified HTTP client for Node.js with support for various HTTP methods and features. It is asynchronous and has been deprecated, but it was widely used before its deprecation.
Make synchronous web requests with cross platform support.
npm install sync-request
request(method, url, options)
e.g.
var request = require('sync-request');
var res = request('GET', 'http://example.com');
console.log(res.getBody());
Method:
An HTTP method (e.g. GET
, POST
, PUT
, DELETE
or HEAD
). It is not case sensitive.
URL:
A url as a string (e.g. http://example.com
). Relative URLs are allowed in the browser.
Options:
qs
- an object containing querystring values to be appended to the uriheaders
- http headers (default: {}
)body
- body for PATCH, POST and PUT requests. Must be a Buffer
or String
(only strings are accepted client side)json
- sets body
but to JSON representation of value and adds Content-type: application/json
. Does not have any affect on how the response is treated.cache
- Can be 'memory'
or 'file'
, and enables a local cache of content. A separate process is still spawned even for cache requests. This option is only used if running in node.jsReturns:
A Response
object.
Note that even for status codes that represent an error, the request function will still return a response. You can call getBody
if you want to error on invalid status codes. The response has the following properties:
statusCode
- a number representing the HTTP status codeheaders
- http response headersbody
- a string if in the browser or a buffer if on the serverIt also has a method res.getBody(encoding?)
which looks like:
function getBody(encoding) {
if (this.statusCode >= 300) {
var err = new Error('Server responded with status code ' + this.statusCode + ':\n' + this.body.toString(encoding));
err.statusCode = this.statusCode;
err.headers = this.headers;
err.body = this.body;
throw err;
}
return encoding ? this.body.toString(encoding) : this.body;
}
Internally, this uses a separate worker process that is run using either childProcess.spawnSync if available, or falling back to spawn-sync if not. The fallback will attempt to install a native module for synchronous execution, and fall back to doing busy waiting for a file to exist. All this ultimatley means that the module is totally cross platform and does not require native code compilation support.
The worker then makes the actual request using then-request so this has almost exactly the same API as that.
This can also be used in a web browser via browserify because xhr has built in support for synchronous execution. Note that this is not recommended as it will be blocking.
MIT
FAQs
Make synchronous web requests
We found that sync-request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.