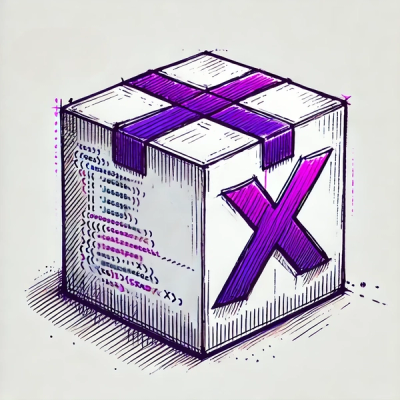
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Communication and Synchronization between browser tabs/windows. Works cross-domain.
sysend.js is a small library that allows to send messages between pages that are open in the same browser. It also supports Cross-Domain communication (Cross-Origin). The library doesn't have any dependencies and uses the HTML5 LocalStorage API or BroadcastChannel API. If your browser don't support BroadcastChannel (see Can I Use) then you can send any object that can be serialized to JSON. With BroadcastChannel you can send any object (it will not be serialized to string but the values are limited to the ones that can be copied by the structured cloning algorithm). You can also send empty notifications.
Tested on:
GNU/Linux: in Chromium 34, Firefox 29, Opera 12.16 (64bit)
Windows 10 64bit: in IE11 and Edge 38, Chrome 56, Firefox 51
MacOS X El Captain: Safari 9, Chrome 56, Firefox 51
All cross-domain communication is disabled by default with Safari 7+. Because of a feature that blocks 3rd party tracking for iframe, and any iframe used for cross-domain communication runs in a sandboxed environment. That's why this library like any other solution for cross-domain communication, don't work on Safari.
Since version 115 Google Chrome introduced Third-party storage partitioning. Because of this feature, Cross-domain communication only works on subdomains. There will probably be a way to share the context using some kind of permission API, that in the future may also land in Safari (hopefully). More information about this can be found in #54. Information about the API can also be found in Google Chrome documentation: Storage Partitioning
There is a new API: Storage Access API. It's available when you register for the Origin Trial.
You can register two and more domains, you will have a token that you need to add to the HTML files (in the head tag):
<meta http-equiv="origin-trial" content="<TOKEN>" />
You can also use the HTTP header:
Origin-Trial: <TOKEN>
Right now, the API only works with localStorage fallback (when inside iframes).
Include sysend.js
file in your html, you can grab the file from npm:
npm install sysend
or bower
bower install sysend
you can also get it from unpkg.com CDN:
https://unpkg.com/sysend
or jsDelivr:
https://cdn.jsdelivr.net/npm/sysend
jsDelivr will minify the file. From my testing it's faster than unpkg.com.
window.onload = function() {
sysend.on('foo', function(data) {
console.log(data.message);
});
var input = document.getElementsByTagName('input')[0];
document.getElementsByTagName('button')[0].onclick = function() {
sysend.broadcast('foo', { message: input.value });
};
};
Tracking is high level API build on top of on()
and broadcast()
, that allows to manage windows/tabs. You can sent message directly to other windows/tabs:
sysend.track('message', ({data, origin}) => {
console.log(`${origin} send message "${data}"`);
});
sysend.post('<ID>', 'Hello other window/tab');
and listen to events like:
sysend.track('open', (data) => {
console.log(`${data.id} window/tab just opened`);
});
Other tracking events includes: close/primary/secondary executed when window/tab is closed or become primary or secondary. Track method was added in version 1.6.0. Another required event is ready
(added in 1.10.0) that should be used when you want to get list of windows/tabs:
sysend.track('ready', () => {
sysend.list().then(tabs => {
console.log(tabs);
});
});
with list()
method and open
/close
events you can implement dynamic list of windows/tab. That will change when new window/tab is open or close.
let list = [];
sysend.track('open', data => {
if (data.id !== sysend.id) {
list.push(data);
populate_list(list);
}
});
sysend.track('close', data => {
list = list.filter(tab => data.id !== tab.id);
populate_list(list);
});
sysend.track('ready', () => {
sysend.list().then(tabs => {
list = tabs;
populate_list(list);
});
});
function populate_list() {
select.innerHTML = '';
list.forEach(tab => {
const option = document.createElement('option');
option.value = tab.id;
option.innerText = tab.id;
select.appendChild(option);
});
}
In version 1.16.0 this code was abstracted into:
sysend.track('update', (list) => {
populate_list(list);
});
This can be simplified with point free style:
sysend.track('update', populate_list);
In version 1.15.0 new API was added called rpc()
(build on top of tracking mechanism) that allow to use RPC (Remote Procedure Call) between open windows/tabs.
const rpc = sysend.rpc({
get_message() {
return document.querySelector('input').value;
}
});
button.addEventListener('click', () => {
rpc.get_message('<ID>').then(message => {
console.log(`Message from other tab is "${message}"`);
}).catch(e => {
console.log(`get_message (ERROR) ${e.message}`);
});
});
If you want to add support for Cross-Domain communication, you need to call proxy method with url on target domain that have proxy.html file.
sysend.proxy('https://jcubic.pl');
sysend.proxy('https://terminal.jcubic.pl');
on Firefox you need to add CORS for the proxy.html that will be loaded into iframe (see Cross-Domain LocalStorage).
if you want to send custom data you can use serializer (new in 1.4.0) this API was created for localStorage that needs serialization.
Example serializer can be json-dry:
sysend.serializer(function(data) {
return Dry.stringify(data);
}, function(string) {
return Dry.parse(string);
});
or JSON5:
sysend.serializer(function(data) {
return JSON5.stringify(string);
}, function(string) {
return JSON5.parse(string);
});
Since version 1.10.0 as a security mesure Cross-Domain communication has been limited to only those domains that are allowed.
To allow domain to listen to sysend communication you need to specify channel inside iframe. You need add your origins to the
sysend.channel()
function (origin is combination of protocol domain and optional port).
sysend object:
function | description | arguments | Version |
---|---|---|---|
on(name, callback) | add event handler for specified name | name - string - The name of the eventcallback - function (object, name) => void | 1.0.0 |
off(name [, callback]) | remove event handler for given name, if callback is not specified it will remove all callbacks for given name | name - string - The name of the eventcallback - optional function (object, name) => void | 1.0.0 |
broadcast(name [, object]) | send any object and fire all events with specified name (in different pages that register callback using on). You can also just send notification without an object | name - string - The name of the event object - optional any data | 1.0.0 |
proxy(<urls>) | create iframe proxy for different domain, the target domain/URL should have proxy.html file. If url domain is the same as page domain, it's ignored. So you can put both proxy calls on both | url - string | 1.3.0 |
serializer(to_string, from_string) | add serializer and deserializer functions | both arguments are functions (data: any) => string | 1.4.0 |
emit(name, [, object]) | same as broadcast() but also invoke the even on same page | name - string - The name of the event object - optional any data | 1.5.0 |
post(<window_id>, [, object]) | send any data to other window | window_id - string of the target window (use 'primary' to send to primary window)object - any data | 1.6.0 / 'primary' target 1.14.0 |
list() | returns a Promise of objects {id:<UUID>, primary} for other windows, you can use those to send a message with post() | NA | 1.6.0 |
track(event, callback) | track inter window communication events | event - any of the strings: "open" , "close" , "primary" , "secondary" , "message" , "update" callback - different function depend on the event: * "message" - {data, origin} - where data is anything the post() sends, and origin is id of the sender.* "open" - {count, primary, id} when new window/tab is opened* "close" - {count, primary, id, self} when window/tab is closed* "primary" and "secondary" function has no arguments and is called when window/tab become secondary or primary.* "ready" - event when tracking is ready. | 1.6.0 except ready - 1.10.0 and update - 1.16.0 |
untrack(event [,callback]) | remove single event listener all listeners for a given event | event - any of the strings 'open' , 'close' , 'primary' , 'secondary' , 'message' , or 'update' . | 1.6.0 |
isPrimary() | function returns true if window is primary (first open or last that remain) | NA | 1.6.0 |
channel() | function restrict cross domain communication to only allowed domains. You need to call this function on proxy iframe to limit number of domains (origins) that can listen and send events. | any number of origins (e.g. 'http://localhost:8080' or 'https://jcubic.github.io') you can also use valid URL. | 1.10.0 |
useLocalStorage([toggle]) | Function set or toggle localStorage mode. | argument is optional and can be true or false . | 1.14.0 |
rpc(object): Promise<fn(id, ...args): Promise> | Function create RPC async functions which accept first additional argument that is ID of window/tab that it should sent request to. The other window/tab call the function and return value resolve original promise. | The function accept an object with methods and return a Promise that resolve to object with same methods but async. | 1.15.0 |
To see details of using the API, see demo.html source code or TypeScript definition file.
The story of this library came from my question on StackOverflow from 2014: Sending notifications between instances of the page in the same browser, with hint from user called Niet the Dark Absol, I was able to create a PoC of the solution using localStorage. I quickly created a library from my solution. I've also explained how to have Cross-Domain LocalStorage. The blog post have steady number of visitors (actually it's most viewed post on that blog).
And the name of the library is just random word "sy" and "send" suffix. But it can be an backronym for Synchronizing Send as in synchronizing application between browser tabs.
The library was featured in:
Copyright (C) 2014 Jakub T. Jankiewicz
Released under the MIT license
This is free software; you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
FAQs
Communication and Synchronization between browser tabs/windows. Works cross-domain.
The npm package sysend receives a total of 1,947 weekly downloads. As such, sysend popularity was classified as popular.
We found that sysend demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.