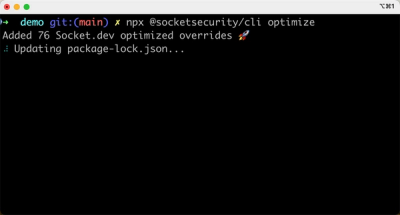
Product
Introducing Socket Optimize
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
syshub-rest-module
Advanced tools
This package provides a generic library for the communication from an Angular SPA with a NT-Ware uniFLOW sysHUB backend server based on HTTPS Rest API with OAuth2 flow.
This package provides a generic library for the communication from an Angular SPA with a NT-Ware uniFLOW sysHUB backend server based on HTTPS Rest API with OAuth2 flow. The contained Rest Service provides methods for login and logout-functionality and an automatic token refresh. Furthermore you can use the get- and post-methods to send a request to any sysHUB Rest API endpoint. The package contains anything needed for the communication, just at it as a provider to your application and provide it with settings like hostname and credentials.
Run npm i syshub-rest-module
in your project root to add this library as dependency.
After installing the module, create a configuration for the credentials, for example in your environment.ts
file. If there is no environment.ts
yet, see this article on StackOverflow.
import { SyshubVersion } from 'syshub-rest-module';
export const environment = {
syshub: {
host: "http://localhost:8088/",
version: SyshubVersion.sysHUB_2022,
basic: {
enabled: false,
username: "foo",
password: "foo!bar",
provider: "<syshubApiserverName>",
},
oauth: {
enabled: true,
clientId: "<syshubAuthserverClientId>",
clientSecret: "<syshubAuthserverClientSecret>",
}
}
};
Make sure to enable either basic auth or oauth. Basic auth requires an authentication provider that you must set up in API Server configuration. This is not required for OAuth authentication. For a detailed explanation of the configuration values see chapter Configuration.
The Rest API had a huge change from 2021 to 2022 version of the server. The API is now available in the url path /webapi/
instead of the old /cosmos-webapi/
. Therefore this module contains a version switch in the configuration. If the switch is not set, it will always use the latest version, currently 2022. In order to use the module with an old 2021 server change the version accordingly.
In your app.module.ts
add the following three providers:
import { RestService, RestSettings, Settings, SyshubInterceptor } from 'syshub-rest-module';
...
providers: [
{ provide: Settings, multi: false, useValue: new Settings(<RestSettings>(environment.syshub)) },
{ provide: RestService, multi: false, deps: [Settings, HttpClient] },
{ provide: HTTP_INTERCEPTORS, multi: true, useClass: SyshubInterceptor, deps: [Settings, RestService] }
],
The Settings-provider requires an initialized object of type Settings
, where the constructor expects an object that implements the RestSettings
interface. For an example, see above the environment.syshub
object in the Install/Setup configuration chapter.
In any component you require access to the REST API import the RestService
:
import { RestService } from 'syshub-rest-module';
Within your components you are now able to access all service methods and properties. For example:
import { RestService } from 'syshub-rest-module';
...
export class AppComponent {
...
constructor(private restService: RestService) {}
...
sendLogin(): void {
this.restService.login(this.username, this.password).subscribe((response) => {
console.log(response)
if (response == null) // initial status
return;
if (response === true) {
// login successfull
}
else {
// login failed
}
});
}
...
}
To send an HTTP GET to the Rest API use get()
method from the RestService, for example:
this.restService.get('currentUser').subscribe({
next(value) {
console.log(value)
},
error(err) {
console.log(err)
},
});
Make sure the user is already logged in (subscribe to RestService.isLoggedIn
or call RestService.getIsLoggedIn()
). If user is not yet loggedin the endpoint will return HTTP Status code 401 (Unauthorized).
To send an HTTP POST to the Rest API use post()
method from the RestService, for example:
this.restService.post('jobs', myJobObject).subscribe({
next(value) {
console.log(value)
},
error(err) {
console.log(err)
},
});
Make sure the user is already logged in (subscribe to RestService.isLoggedIn
or call RestService.getIsLoggedIn()
). If user is not yet loggedin the endpoint will return HTTP Status code 401 (Unauthorized). Also make sure that your provided object matches the definition from sysHUB as you can find it in the Swagger UI.
The sysHUB Rest API also expects the HTTP methods Delete, Patch or Put depending on the endpoint. Therefore the RestService provides those methods too: RestService.delete()
, RestService.patch()
and RestService.put()
.
As they are work almost identically to the Get and Post methods, there's no separate usage guide here. Just replace RestService.get()
with RestService.delete()
to call a Delete-Endpoint. Replace RestService.post()
with RestService.patch()
or RestService.put()
to call the Patch and Put endpoints. The later ones also expect an object to be sent to the Webserver.
The configuration of the RestService is done with a Settings
object which requires a RestSettings
object. The later one can easily be created within the environments
-files or at runtime (see Install and Usage).
The RestSettings
object must have basic or oauth enabled and may allow some other values:
host
: Required (type string Url); Url to the sysHUB Webserer root, like http://localhost:8088/
.version
: Optional, required for sysHUB 2021 server; Pick one value from the enum:
SyshubVersion.sysHUB_2021
SyshubVersion.sysHUB_2022
SyshubVersion.DEFAULT
which is the same as SyshubVersion.sysHUB_2022
basic
: Optional, required for basic authentication (type object)
enabled
: Required (type boolean); true
if basic auth to be enabled, default false
.username
: Optional, required for basic authentication (type string)password
: Optional, required for basic authentication (type string)provider
: Optional, required for basic authentication (type string); The name of the Api server provider from the sysHUB configurationoauth
: Optional, required for OAuth (type object)
enabled
: Required (type boolean); true
if OAuth to be enabled, default false
.clientId
: Optional, required for OAuth (type string); The name of the Auth server clientclientSecret
: Optional, required for OAuth (type string); The name of the Auth server client secretscope
: Optional (type string); The scope as defined in the sysHUB Auth server, replace ;
with +
. Allowed values: private
, public
, private+public
or public+private
, Default: public
storeKey
: Optional (type string); The name that is used to store the credentials in the local browser cache, Default: authmod-session
options
: Optional (type object)
autoConnect
, Optional (type boolean), Not yet implementedautoLogoutOn401
, Optional (type boolean), If the sysHUB Rest API returns HTTP Status 401, this property causes the Rest Service to remove the users session information from the browser cache, Default: true
autoLogoutTimer
, Optional (type boolean), Not yet implemented[1.1.0] - 2023-03-02
version
to environment.syshub
. This version switch uses the enum SyshubVersion
which is content of this module. If the version switch is not provided default will be sysHUB 2022.FAQs
This package provides a generic library for the communication from an Angular SPA with a NT-Ware uniFLOW sysHUB backend server based on HTTPS Rest API with OAuth2 flow.
The npm package syshub-rest-module receives a total of 2 weekly downloads. As such, syshub-rest-module popularity was classified as not popular.
We found that syshub-rest-module demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
Product
We're excited to announce that Socket now supports the Java programming language.
Security News
Socket detected a malicious Python package impersonating a popular browser cookie library to steal passwords, screenshots, webcam images, and Discord tokens.