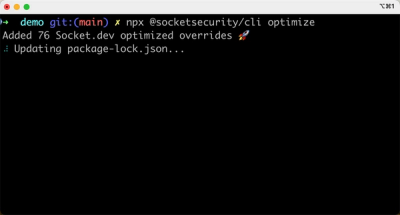
Product
Introducing Socket Optimize
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
syshub-rest-module
Advanced tools
This package provides a generic library for the communication from an Angular SPA with a NT-Ware uniFLOW sysHUB backend server based on HTTPS Rest API with OAuth2 flow.
The syshub-rest-module provides an encapsulated module for communication between an Angular single-page application (SPA) and an NT-Ware uniFLOW sysHUB server. Communication takes place via the OAuth2-based rest interface provided by the sysHUB server, optionally via basic authentication.
The RestService included in this module provides generic methods for HTTP GET, POST, DELETE, PATCH and PUT operations as well as type-safe methods like getCurrentUser()
. After a user logs in via OAuth, the module takes care of renewing the session token shortly before expiry.
Follow the installation instructions below to integrate the module into an Angular app.
Run npm i syshub-rest-module
in your project root to add this library as dependency.
After installing the module, create a configuration for the credentials, for example in your environment.ts
file. If there is no environment.ts
yet, see this article on StackOverflow.
Make sure that your configuration implements the Env
interface, so that type checking prevents errors already during the development of the app. For each property within the Env
type, type hints are implemented, please take note of them. For example, it is not intended to enable OAuth and Basic Auth in parallel (as described in the type hint). If both are activated anyway, an error will be thrown.
import { Env, SyshubVersion } from 'syshub-rest-module';
export const environment: Env = {
syshub: {
host: "http://localhost:8088/",
version: SyshubVersion.sysHUB_2023,
basic: {
enabled: false,
username: "foo",
password: "foo!bar",
provider: "<syshubApiserverName>",
},
oauth: {
enabled: true,
clientId: "<syshubAuthserverClientId>",
clientSecret: "<syshubAuthserverClientSecret>",
scope: "private+public"
}
}
};
The Env
data type in the environment.ts
file forms the basic configuration unit for the RestModule and is intended to ensure that only valid properties are set. This detailed specification describes all properties and their permitted values and effects. The description is also stored within the module so that it is shown in the code editor.
Env
is not mandatory. It contains the property syshub
(Type RestSettings
, see below), which must be used in the constructor of the Settings
class. If environment
is not declared as Env
, it must be otherwise ensured that syshub
conforms to the type RestSettings
.
Root element for the Settings
class constructor that is essential for configuring the RestService.
host
: Required (type string URL); URL to the sysHUB Webserver root, for example: http://localhost:8088/
.
version
: Optional, required for sysHUB 2021 server; Pick one value from the enum:
SyshubVersion.sysHUB_2021
SyshubVersion.sysHUB_2022
SyshubVersion.sysHUB_2023
SyshubVersion.DEFAULT
which is the same as SyshubVersion.sysHUB_2023
basic
: Optional, required for basic authentication (type object)
enabled
: Required (type boolean); true
if basic auth is to be enabled, default false
.username
: Optional, required for basic authentication (type string)password
: Optional, required for basic authentication (type string)provider
: Optional, required for basic authentication (type string); The name of the API server provider from the sysHUB configuration.oauth
: Optional, required for OAuth (type object)
enabled
: Required (type boolean); true
if OAuth is to be enabled, default false
.clientId
: Optional, required for OAuth (type string); The name of the Auth server client.clientSecret
: Optional, required for OAuth (type string); The name of the Auth server client secret.scope
: Optional (type string); The scope as defined in the sysHUB Auth server, replace ;
with +
. Allowed values: private
, public
, private+public
, or public+private
. Default: public
.storeKey
: Optional (type string); The name that is used to store the credentials in the local browser cache. Default: authmod-session
.options
: Optional (type object)
autoConnect
, Optional (type boolean), Not yet implemented.autoLogoutOn401
, Optional (type boolean), If the sysHUB Rest API returns HTTP Status 401, this property causes the Rest Service to remove the user's session information from the browser cache. Default: true
.autoLogoutTimer
, Optional (type boolean), Not yet implemented.The Rest API has changed significantly from version 2021 to version 2022 of the server. The API is now available via /webapi/
instead of the old /cosmos-webapi/
, which is why this module contains a version switch in the configuration. If the switch is not set, the latest version, currently 2023, is always used. To use the module with an old 2021 server, change the version accordingly.
In your app.module.ts
make sure that the HttpClientModule
is imported and add the three providers Settings
, RestService
and HTTP_INTERCEPTORS
. The minimal configuration looks something like this:
import { HTTP_INTERCEPTORS, HttpClient, HttpClientModule } from '@angular/common/http';
import { NgModule } from '@angular/core';
import { RestService, RestSettings, Settings, SyshubInterceptor } from 'syshub-rest-module';
@NgModule({
imports: [
HttpClientModule,
],
providers: [
{ provide: Settings, multi: false, useValue: new Settings(<RestSettings>(environment.syshub)) },
{ provide: RestService, multi: false, deps: [Settings, HttpClient] },
{ provide: HTTP_INTERCEPTORS, multi: true, useClass: SyshubInterceptor, deps: [Settings, RestService] },
],
})
export class AppModule { }
The Settings
provider requires an initialised object of the class Settings
, whose constructor in turn expects an object corresponding to the type RestSettings
. For an example, see object environment.syshub
in the Install/Setup configuration chapter.
In each component that needs access to the sysHUB Rest API, the RestService
must be imported and injected in the constructor of the component. Minimal example:
import { RestService } from 'syshub-rest-module';
@Component({})
export class FooComponent {
constructor(private restService: RestService) {}
}
The user login is programmed with a few lines of code. In the following example, the onSubmitBtnClicked()
method is called by clicking a button in the HTML and the contents of the variables username
and password
are sent to the sysHUB server via the login()
method of the RestService. The response is then either true
in case of success or an error containing further information. In case of success, the Rest Service saves the received token and takes care of its renewal.
import { Component } from '@angular/core';
import { RestService } from 'syshub-rest-module';
@Component({
selector: 'app-login-min',
templateUrl: './login-min.component.html',
styleUrls: ['./login-min.component.scss']
})
export class LoginMinComponent {
username: string = 'foo';
password: string = '<foobar!>';
constructor(private restService: RestService) { }
onSubmitBtnClicked(): void {
this.restService.login(this.username, this.password).subscribe((response) => {
if (response === null) // Initial status, not yet any response from server
return;
if (response === true) // Login successfull
console.log(`User ${this.username} logged in`);
else // Error while logging in, see details in response
console.log(response);
});
}
}
Before sending a request to the server's Rest API, it should be ensured that a user is logged in. For this, either the method getIsLoggedIn()
of the RestService can be queried or subscribed to isLoggedIn
. isLoggedIn
reports every change of the login status and so it is also possible to react on successful logout and login.
In the following example code (which supplements the code from the previous section Userlogin example), the properties loggedIn
and sub
have been added. In the constructor, RestService.isLoggedIn
is subscribed to and any status change is stored in loggedIn
. The subscription itself is stored in sub
and terminated when the component is exited.
import { Component, OnDestroy } from '@angular/core';
import { Subscription } from 'rxjs';
import { RestService } from 'syshub-rest-module';
@Component({
selector: 'app-login-min',
templateUrl: './login-min.component.html',
styleUrls: ['./login-min.component.scss']
})
export class LoginMinComponent implements OnDestroy {
username: string = 'foo';
password: string = '<foobar!>';
loggedIn: boolean = false;
sub?: Subscription;
constructor(private restService: RestService) {
this.sub = this.restService.isLoggedIn.subscribe((state) => this.loggedIn = state);
}
ngOnDestroy(): void {
this.sub?.unsubscribe();
}
onSubmitBtnClicked(): void {
this.restService.login(this.username, this.password).subscribe((response) => {
if (response === null) // Initial status, not yet any response from server
return;
if (response === true) // Login successfull
console.log(`User ${this.username} logged in`);
else // Error while logging in, see details in response
console.log(response);
});
}
}
Calling Rest API endpoints is quite simple with the RestService. Call one of the generic methods get()
, post()
, delete()
, patch()
or put()
of the RestService to reach any endpoint. For post()
, patch()
and put()
a payload is required in addition to the endpoint. The content of the payload can be seen in the Swagger definition.
Important: The actual call to the sysHUB server only takes place when the result is subscribed to, nothing happens before that.
this.restService.get('currentUser').subscribe((response) => {
if (response.status == HttpStatusCode.Ok) {
// Ok response
/**
* The sysHUB server may return a status code other than 200/OK.
* The specification for this can be found in Swagger.
*/
} else {
//Failed response
}
});
this.restService.post('jobs', {...}).subscribe((response) => {
if (response.status == HttpStatusCode.Ok) {
// Ok response
/**
* The sysHUB server may return a status code other than 200/OK.
* The specification for this can be found in Swagger.
*/
} else {
//Failed response
}
});
The sysHUB Rest API also expects the HTTP methods Delete, Patch or Put depending on the endpoint. Accordingly, the RestService also provides these methods.
Since they work almost identically to the get()
and post()
methods, there are no separate instructions for their use here. Just replace RestService.get()
with RestService.delete()
to call a delete endpoint. Replace RestService.post()
with RestService.patch()
or RestService.put()
to call the patch and put endpoints. The latter also expect an object to be sent to the web server.
As of version 2.0, this module also includes specialised, type-safe methods to call endpoints specifically and not use the generic way of e.g. get()
. These methods are programmed so that both the parameters for the rest of the API call are typed, as are the return values from the sysHUB server. To do this, the method interposes itself between your calling code and the underlying generic method, typing all variables.
As an example, consider the method getCurrentUser()
: This method calls the endpoint currentUser
via HTTP GET and types the return value from the sysHUB server as type SyshubUserAccount
. This data type is also included in the module and contains all the properties that the sysHUB server is guaranteed to deliver. Your calling code will then receive either this object or an object of type Error
as a response, for example because no user is logged in.
The following list is sorted by topic and endpoint (like Swagger).
Method | Return type | Scope | Verb | Endpoint |
---|---|---|---|---|
Backup and Restore operations | ||||
backupSyshub() | SyshubResponseSimple | public | POST | /webapi/v3/backuprestore/backup?folder=... |
restoreSyshub() | SyshubResponseSimple | public | POST | /webapi/v3/backuprestore/restore?folder=... |
getBackupMetadata() | SyshubBackupMeta | public | GET | /webapi/v3/backuprestore/metadata?folder=... |
Certificate operations | ||||
getCertStoreItems() | SyshubCertStoreItem | public | GET | /webapi/v3/certificate/list/... |
Console operations | ||||
runConsoleCommand() | string[] | public | POST | /webapi/v3/consolecommands/execute/... |
runConsoleCommandHelp() | { [key: string]: string } (key = command, value = description of the command) | public | POST | /webapi/v3/consolecommands/execute/HELP |
runConsoleCommandMem() | SyshubMemCommandResult | public | POST | /webapi/v3/consolecommands/execute/MEM |
runConsoleCommandP() | SyshubPCommandLine[] | public | POST | /webapi/v3/consolecommands/execute/P |
Job operations | ||||
getJobs() | JobsResponse / SyshubJob[] | public | GET | /webapi/v3/jobs |
createJob() | JobResponse / SyshubJob | public | POST | /webapi/v3/jobs |
Server operations | ||||
getServerInformation() | SyshubServerInformation | public | GET | /webapi/v3/list/information |
getServerProperties() | { [key: string]: string } | private | GET | /webapi/v3/server/properties |
User operations | ||||
getCurrentUser() | SyshubUserAccount | public | GET | /webapi/v3/currentUser |
Workflow operations | ||||
getWorkflowExecutions() | SyshubWorkflowExecution[] | public | GET | /webapi/v3/workflows/execute |
runWorkflow() | [string, number] (string = Status Url, number = HTTP Status) | public | POST | /webapi/v3/workflows/execute |
getWorkflowExecution() | SyshubWorkflowExecution[] | public | GET | /webapi/v3/workflows/execute?uuid=... |
runWorkflowAlias() | any | public | POST | /webapi/v3/execute/alias/... |
This method calls the POST endpoint /webapi/v3/backuprestore/backup?folder=...
.
string backupName
Name for this backup.string backupDescription
Description text for this backup.string folderpath
Backup folder that will be created inside sysHUB root folder.string[] includeOptions
Array of options to backup. You can use the enum SyshubBackupTypesEnum
provided with this module. This enum contains all available Options that may be used with this endpoint (Usage: Object.keys(SyshubBackupTypesEnum).filter((item) => isNaN(Number(item)))
to create a string[]
of all available objects).SyshubResponseSimple
This method calls the POST endpoint /webapi/v3/jobs
.
SyshubJob job
Job definition.JobResponse
which contains the location header to query information about the created job and the job object as content.This method calls the GET endpoint /webapi/v3/backuprestore/metadata?folder=...
.
string folderpath
Backup folder that will be created inside sysHUB root folder.SyshubBackupMeta
This method calls the GET endpoint /webapi/v3/certificate/list/...
.
store: 'keystore' | 'truststore'
SyshubCertStoreItem
This method calls the GET endpoint /webapi/v3/currentUser
.
SyshubUserAccount
This method calls the GET endpoint /webapi/v3/jobs
.
limit: number|undefined
: If set limit the returned number of job objects; Must also set offsetoffset: number|undefined
: If set offset of the first returned job object; Must also set limitorderby: string|undefined
: Sort by this column and direction, e.g. id.desc
search: string|undefined
: RSQL like filterJobsResponse
which contains content of type SyshubJob[]
and header which contains number of matched jobsThis method calls the GET endpoint /webapi/v3/server/list/information
.
SyshubServerInformation
This method calls the GET endpoint /webapi/v3/server/properties
.
{ [key: string]: string }
This method calls the GET endpoint /webapi/v3/workflows/execute?uuid=...
and returns a list of running workflow executions started via Rest API and matching the given uuid param.
SyshubWorkflowExecution[]
; Be aware that the documentation states the endpoint returns an SyshubWorkflowExecution
object although in sysHUB 2022 and 2023 it returns an array with one object inside (if uuid is found). This may change in upcoming versions to match the documentation.This method calls the GET endpoint /webapi/v3/workflows/execute
and returns a list of running workflow executions started via Rest API.
SyshubWorkflowExecution[]
This method calls the POST endpoint /webapi/v3/backuprestore/restore?folder=...
.
string folderpath
Backup folder that will be created inside sysHUB root folder.string[] includeOptions
Array of options to backup. You can use the enum SyshubBackupTypesEnum
provided with this module. This enum contains all available Options that may be used with this endpoint (Usage: Object.keys(SyshubBackupTypesEnum).filter((item) => isNaN(Number(item)))
to create a string[]
of all available objects).SyshubResponseSimple
This method calls the POST endpoint /webapi/v3/consolecommands/execute/...
.
cmd: string
The command to executeparams: string[] = []
An array of parameters to send along with the commandstring[]
You may use one of the specialiced methods to get the string[]
converted to a javascript object.
This method calls the POST endpoint /webapi/v3/consolecommands/execute/HELP
.
{ [key: string]: string }
(key = command, value = description of the command)This method calls the POST endpoint /webapi/v3/consolecommands/execute/MEM
.
SyshubMemCommandResult
This method calls the POST endpoint /webapi/v3/consolecommands/execute/P
.
SyshubPCommandLine[]
This method calls the POST endpoint /webapi/v3/workflows/execute
and starts an workflow execution optionally with job context.
string uuid
sysHUB Workflow Uuid to executebool async = true
Determines whether to run the workflow synchronous or asynchronous.number|undefined jobId
If set the workflow will be executed with the given job as currentjob.[string, number]
string
: An Url sent by the sysHUB server refering to another Rest API Endpoint including the Uuid of the execution. This Uuid can be used in other Rest API calls to get the result of the execution.number
: The HTTP Status code as this endpoint may return 201 or 202This method calls the endpoint /webapi/v3/workflows/execute/alias/...
based on the given method (param 3) and returns whatever the workflow returns.
string alias
sysHUB Workflow Alias nameany|undefined payload
Any payload you want to send to the workflows httpbody object'DELETE'|'GET'|'POST'|'PUT' method
Give one method to use for the communication with sysHUB, default is 'POST'response
dictionary objectFAQs
This package provides a generic library for the communication from an Angular SPA with a NT-Ware uniFLOW sysHUB backend server based on HTTPS Rest API with OAuth2 flow.
The npm package syshub-rest-module receives a total of 2 weekly downloads. As such, syshub-rest-module popularity was classified as not popular.
We found that syshub-rest-module demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
Product
We're excited to announce that Socket now supports the Java programming language.
Security News
Socket detected a malicious Python package impersonating a popular browser cookie library to steal passwords, screenshots, webcam images, and Discord tokens.