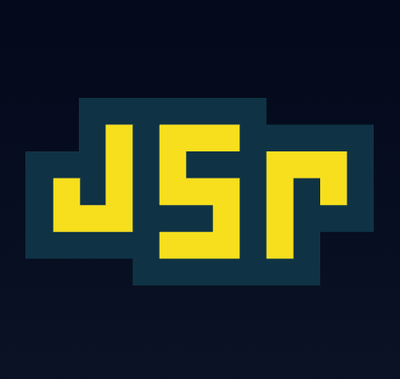
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The table npm package is a utility for generating text-based tables for Node.js applications. It provides a simple yet flexible way to create and manipulate tables in the console or other text-based outputs. It supports custom borders, padding, alignment, and more, making it a versatile tool for displaying tabular data in a structured format.
Basic table creation
This feature allows you to create a simple table by providing an array of arrays. Each sub-array represents a row in the table. The first sub-array can be used for headers.
"use strict";\nconst {table} = require('table');\nlet data = [\n ['Name', 'Age'],\n ['Alice', 24],\n ['Bob', 19]\n];\nconsole.log(table(data));
Custom border styles
This feature allows customization of the table's border by using predefined border styles or creating custom ones. It enhances the visual appearance of the table.
"use strict";\nconst {table, getBorderCharacters} = require('table');\nlet config = {\n border: getBorderCharacters('ramac')\n};\nlet data = [\n ['Name', 'Age'],\n ['Alice', 24],\n ['Bob', 19]\n];\nconsole.log(table(data, config));
Column width and alignment
This feature allows you to specify the width and alignment of columns, providing control over the layout and presentation of data within the table.
"use strict";\nconst {table} = require('table');\nlet config = {\n columns: {\n 0: {\n width: 10,\n alignment: 'left'\n },\n 1: {\n width: 5,\n alignment: 'right'\n }\n }\n};\nlet data = [\n ['Name', 'Age'],\n ['Alice', 24],\n ['Bob', 19]\n];\nconsole.log(table(data, config));
cli-table is a similar package that provides functionalities for rendering unicode-aided tables on the command line. Compared to table, cli-table offers a different API and customization options but serves a similar purpose of displaying tabular data in CLI applications.
ascii-table is another package for creating ASCII tables in Node.js. It is simpler and less feature-rich compared to table, focusing on basic table creation without the extensive customization options that table provides.
Produces a string that represents array data in a text table.
minWidth
.maxWidth
.Table data is described using an array (rows) of array (cells).
import table from 'table';
let data,
output;
data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
/**
* @typedef {String} table~cell
*/
/**
* @typedef {table~cell[]} table~row
*/
/**
* @typedef {Object} table~configColumn
* @property {String} alignment Cell content alignment (enum: left, center, right) (default: left).
* @property {Number} minWidth Minimum column width (default: 0).
* @property {Number} maxWidth Maximum column width (default: Infinity).
* @property {Number} paddingLeft Cell content padding width left (default: 0).
* @property {Number} paddingRight Cell content padding width right (default: 0).
*/
/**
* @typedef {Object} table~configBorder
* @property {String} topBody
* @property {String} topJoin
* @property {String} topLeft
* @property {String} topRight
* @property {String} bottomBody
* @property {String} bottomJoin
* @property {String} bottomLeft
* @property {String} bottomRight
* @property {String} bodyLeft
* @property {String} bodyRight
* @property {String} bodyJoin
* @property {String} joinBody
* @property {String} joinLeft
* @property {String} joinRight
* @property {String} joinJoin
*/
/**
* @typedef {Object} table~config
* @property {table~configBorder}
* @property {table~configColumn[]} column Column specific configuration.
*/
/**
* Generates a text table.
*
* @param {table~row[]} rows
* @param {table~config} config
* @return {String}
*/
output = table(data);
console.log(output);
╔══╤══╤══╗
║0A│0B│0C║
╟──┼──┼──╢
║1A│1B│1C║
╟──┼──┼──╢
║2A│2B│2C║
╚══╧══╧══╝
border
property describes the characters used to draw the table borders.
let data,
output,
options;
data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
options = {
border: {
topBody: `─`,
topJoin: `┬`,
topLeft: `┌`,
topRight: `┐`,
bottomBody: `─`,
bottomJoin: `┴`,
bottomLeft: `└`,
bottomRight: `┘`,
bodyLeft: `│`,
bodyRight: `│`,
bodyJoin: `│`,
joinBody: `─`,
joinLeft: `├`,
joinRight: `┤`,
joinJoin: `┼`
}
};
output = table(data, options);
console.log(output);
┌──┬──┬──┐
│0A│0B│0C│
├──┼──┼──┤
│1A│1B│1C│
├──┼──┼──┤
│2A│2B│2C│
└──┴──┴──┘
You can load one of the predefined border templates using border
function.
import table from 'table';
import {
border
} from 'table';
let data;
data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
table(data, {
border: border(`name of the template`)
});
# honeywell
╔══╤══╤══╗
║0A│0B│0C║
╟──┼──┼──╢
║1A│1B│1C║
╟──┼──┼──╢
║2A│2B│2C║
╚══╧══╧══╝
# norc
┌──┬──┬──┐
│0A│0B│0C│
├──┼──┼──┤
│1A│1B│1C│
├──┼──┼──┤
│2A│2B│2C│
└──┴──┴──┘
# ramac (ASCII; for use in terminals that do not support Unicode characters)
+--+--+--+
|0A|0B|0C|
|--|--|--|
|1A|1B|1C|
|--|--|--|
|2A|2B|2C|
+--+--+--+
Raise an issue if you'd like to contribute a new border template.
minWidth
property pads the string to fill the cell.
let data,
output,
options;
data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
options = {
column: {
1: {
minWidth: 10
}
}
};
output = table(data, options);
console.log(output);
╔══╤══════════╤══╗
║0A│0B │0C║
╟──┼──────────┼──╢
║1A│1B │1C║
╟──┼──────────┼──╢
║2A│2B │2C║
╚══╧══════════╧══╝
maxWidth
property makes the overflowing text break into multiple lines.
let data,
output,
options;
data = [
['0A', 'AAABBBCCC', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
options = {
column: {
1: {
maxWidth: 3
}
}
};
output = table(data, options);
console.log(output);
╔══╤═══╤══╗
║0A│AAA│0C║
║ │BBB│ ║
║ │CCC│ ║
╟──┼───┼──╢
║1A│1B │1C║
╟──┼───┼──╢
║2A│2B │2C║
╚══╧═══╧══╝
alignment
property controls content horizontal alignment within a cell.
Valid values are: "left", "right" and "center".
let data,
output,
options;
data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
options = {
column: {
0: {
alignment: 'left',
minWidth: 10
},
1: {
alignment: 'center',
minWidth: 10
},
2: {
alignment: 'right',
minWidth: 10
}
}
};
output = table(data, options);
console.log(output);
╔══════════╤══════════╤══════════╗
║0A │ 0B │ 0C║
╟──────────┼──────────┼──────────╢
║1A │ 1B │ 1C║
╟──────────┼──────────┼──────────╢
║2A │ 2B │ 2C║
╚══════════╧══════════╧══════════╝
paddingLeft
and paddingRight
properties control content padding within a cell. Property value represents a number of whitespaces using to pad the content.
let data,
output,
options;
data = [
['0A', 'AABBCC', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
options = {
column: {
0: {
paddingLeft: 3
},
1: {
maxWidth: 2,
paddingRight: 3
}
}
};
output = table(data, options);
console.log(output);
╔═════╤═════╤══╗
║ 0A│AA │0C║
║ │BB │ ║
║ │CC │ ║
╟─────┼─────┼──╢
║ 1A│1B │1C║
╟─────┼─────┼──╢
║ 2A│2B │2C║
╚═════╧═════╧══╝
FAQs
Formats data into a string table.
The npm package table receives a total of 10,821,642 weekly downloads. As such, table popularity was classified as popular.
We found that table demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.