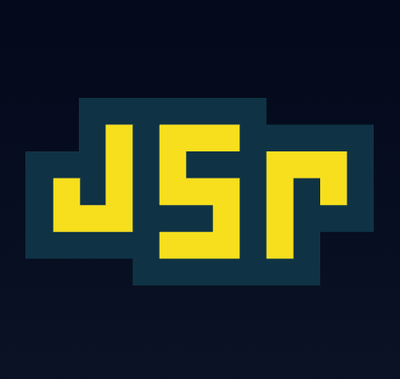
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The 'tap' npm package is a Test Anything Protocol (TAP) producer for Node.js. It is used for writing tests in JavaScript and provides a simple way to create and run tests, generate reports, and handle assertions.
Basic Test Creation
This feature allows you to create basic tests using the 'tap' module. The example demonstrates a simple test that checks if 1 + 1 equals 2.
const tap = require('tap');
tap.test('basic test', t => {
t.equal(1 + 1, 2, '1 + 1 should equal 2');
t.end();
});
Asynchronous Testing
This feature allows you to write asynchronous tests. The example shows how to use async/await to handle asynchronous operations within a test.
const tap = require('tap');
tap.test('async test', async t => {
const result = await new Promise(resolve => setTimeout(() => resolve(42), 100));
t.equal(result, 42, 'result should be 42');
t.end();
});
Nested Tests
This feature allows you to create nested tests. The example demonstrates a parent test containing a child test, which helps in organizing tests hierarchically.
const tap = require('tap');
tap.test('parent test', t => {
t.test('child test', t => {
t.equal(2 * 2, 4, '2 * 2 should equal 4');
t.end();
});
t.end();
});
Assertions
This feature provides various assertion methods to validate test conditions. The example shows different types of assertions like ok, notOk, equal, and notEqual.
const tap = require('tap');
tap.test('assertions test', t => {
t.ok(true, 'this should be true');
t.notOk(false, 'this should be false');
t.equal(3, 3, '3 should equal 3');
t.notEqual(3, 4, '3 should not equal 4');
t.end();
});
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple and fun. It provides a variety of interfaces (BDD, TDD, etc.) and supports a wide range of reporters. Compared to 'tap', Mocha is more flexible and has a larger community.
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works out of the box for most JavaScript projects and provides a rich API for assertions, mocking, and more. Jest is more opinionated and comes with built-in features like snapshot testing, which 'tap' does not offer.
AVA is a test runner for Node.js with a concise API, detailed error output, and process isolation. It runs tests concurrently, which can lead to faster test execution. AVA's approach to concurrency and simplicity makes it different from 'tap', which runs tests sequentially by default.
This is a mix-and-match set of utilities that you can use to write test harnesses and frameworks that communicate with one another using the Test Anything Protocol.
If you don't yet know what TAP is, you better ask somebody.
Default Usage:
npm install tap
tap ./tests
The output will be TAP-compliant.
For extra special bonus points, you can do something like this:
var test = require("tap").test
test("make sure the thingie is a thing", function (t) {
t.equal(thingie, "thing", "thingie should be thing")
t.type(thingie, "string", "type of thingie is string")
t.ok(true, "this is always true")
t.notOk(false, "this is never true")
t.test("a child test", function (t) {
t.equal(this, superEasy, "right!?")
t.similar(7, 2, "ever notice 7 is kinda like 2?", {todo: true})
t.test("so skippable", {skip: true}, function (t) {
t.plan(1) // only one test in this block
t.ok(true, "but when the flag changes, it'll pass")
// no need to end, since we had a plan.
})
t.end()
})
t.ok(99, "can also skip individual assertions", {skip: true})
// end lets it know it's over.
t.end()
})
test("another one", function (t) {
t.plan(1)
t.ok(true, "It's ok to plan, and also end. Watch.")
t.end() // but it must match the plan!
})
Node-tap is actually a collection of several packages, any of which may be mixed and matched however you please.
If you don't like this test framework, and think you can do much much
better, I strongly encourage you to do so! If you use this library,
however, at least to output TAP-compliant results when process.env.TAP
is set, then the data coming out of your framework will be much more
consumable by machines.
That matters. Or rather, it will, very soon.
You can also use this to build programs that consume the TAP data, so this is very useful for CI systems and such.
More docs coming soon, hopefully.
FAQs
A Test-Anything-Protocol library for JavaScript
The npm package tap receives a total of 244,177 weekly downloads. As such, tap popularity was classified as popular.
We found that tap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.