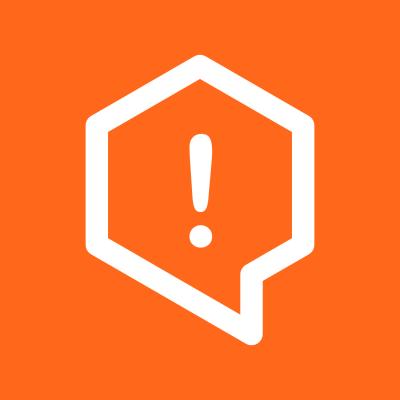
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The tarn.js package is a small, robust resource pool for JavaScript. It is primarily used for managing a pool of resources such as database connections, ensuring efficient resource utilization and scalability in applications. It provides features like acquiring and releasing resources, timeout handling, and error management.
Resource Pool Management
This code demonstrates how to create a resource pool with minimum and maximum limits, and manage resource acquisition and release. It handles timeouts and resource reaping intervals.
const { Pool } = require('tarn');
const pool = new Pool({
create: () => Promise.resolve(new Resource()),
destroy: resource => Promise.resolve(resource.dispose()),
min: 2,
max: 10,
acquireTimeoutMillis: 30000,
idleTimeoutMillis: 30000,
reapIntervalMillis: 1000
});
pool.acquire().promise.then(resource => {
console.log('Resource acquired');
pool.release(resource);
});
generic-pool is a robust resource pooling library similar to tarn. It supports priority queuing and more extensive configuration options for resource management. While tarn focuses on simplicity and specific use cases, generic-pool offers broader flexibility and customization.
pool2 is another resource pooling library that provides features like deferred resource creation and better error handling. It is designed to be a more modern and feature-rich alternative to older pooling libraries, offering a different API but similar core functionalities as tarn.
Tarn is focused on robustness and ability to recover from errors. Tarn has timeouts for all operations that can fail or timeout so that you should never end up with pool full of crap. Tarn has a comprehensive test suite and we are committed to adding tests and fixing all bugs that are found.
Tarn will always remain simple.
npm install tarn
const { Pool, TimeoutError } = require('tarn');
const pool = new Pool({
// Function that creates a resource. You can either pass the resource
// to the callback(error, resource) or return a promise that resolves the resource
// (but not both) Callback syntax will be deprecated at some point.
create: cb => {
cb(null, new SomeResource());
},
// Validates a connection before it is used. Return true or false
// from it. If false is returned, the resource is destroyed and
// another one is acquired. Should return a Promise if validate is
// an async function.
validate: resource => {
return true;
},
// Function that destroys a resource, should return a promise if
// destroying is an asynchronous operation.
destroy: someResource => {
someResource.cleanup();
},
// logger function, noop by default
log: (message, logLevel) => console.log(`${logLevel}: ${message}`)
// minimum size
min: 2,
// maximum size
max: 10,
// acquire promises are rejected after this many milliseconds
// if a resource cannot be acquired
acquireTimeoutMillis: 30000,
// create operations are cancelled after this many milliseconds
// if a resource cannot be acquired
createTimeoutMillis: 30000,
// destroy operations are awaited for at most this many milliseconds
// new resources will be created after this timeout
destroyTimeoutMillis: 5000,
// free resouces are destroyed after this many milliseconds
idleTimeoutMillis: 30000,
// how often to check for idle resources to destroy
reapIntervalMillis: 1000,
// how long to idle after failed create before trying again
createRetryIntervalMillis: 200,
// If true, when a create fails, the first pending acquire is
// rejected with the error. If this is false (the default) then
// create is retried until acquireTimeoutMillis milliseconds has
// passed.
propagateCreateError: false
});
// acquires a resource. The promise is rejected with `tarn.TimeoutError`
// after `acquireTimeoutMillis` if a resource could not be acquired.
const acquire = pool.acquire();
// acquire can be aborted using the abort method.
// If acquire had triggered creating a new resource in the pool
// creation will continue and it is not aborted.
acquire.abort();
// the acquire object has a promise property that gets resolved with
// the acquired resource
try {
const resource = await acquire.promise;
} catch (err) {
// if the acquire times out an error of class TimeoutError is thrown
if (err instanceof TimeoutError) {
console.log('timeout');
}
}
// releases the resource.
pool.release(resource);
// returns the number of non-free resources
pool.numUsed();
// returns the number of free resources
pool.numFree();
// how many acquires are waiting for a resource to be released
pool.numPendingAcquires();
// how many asynchronous create calls are running
pool.numPendingCreates();
// waits for all resources to be returned to the pool and destroys them.
// pool cannot be used after this.
await pool.destroy();
// The following examples add synchronous event handlers. For example, to
// allow externally collecting pool behaviour diagnostic data.
// If any of these hooks fail, all errors are caught and warnings are logged.
// resource is acquired from pool
pool.on('acquireRequest', eventId => {});
pool.on('acquireSuccess', (eventId, resource) => {});
pool.on('acquireFail', (eventId, err) => {});
// resource returned to pool
pool.on('release', resource => {});
// resource was created and added to the pool
pool.on('createRequest', eventId => {});
pool.on('createSuccess', (eventId, resource) => {});
pool.on('createFail', (eventId, err) => {});
// resource is destroyed and evicted from pool
// resource may or may not be invalid when destroySuccess / destroyFail is called
pool.on('destroyRequest', (eventId, resource) => {});
pool.on('destroySuccess', (eventId, resource) => {});
pool.on('destroyFail', (eventId, resource, err) => {});
// when internal reaping event clock is activated / deactivated
pool.on('startReaping', () => {});
pool.on('stopReaping', () => {});
// pool is destroyed (after poolDestroySuccess all event handlers are also cleared)
pool.on('poolDestroyRequest', eventId => {});
pool.on('poolDestroySuccess', eventId => {});
// remove single event listener
pool.removeListener(eventName, listener);
// remove all listeners from an event
pool.removeAllListeners(eventName);
Released as major version, because async validation support did require lots of internal changes, which may cause subtle difference in behavior.
FAQs
Simple and robust resource pool for node.js
The npm package tarn receives a total of 1,934,056 weekly downloads. As such, tarn popularity was classified as popular.
We found that tarn demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.