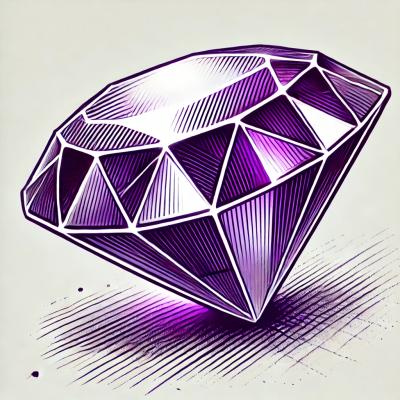
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
taskgroup
Advanced tools
Group together synchronous and asynchronous tasks and execute them with support for concurrency, naming, and nesting.
Group together synchronous and asynchronous tasks and execute them with support for concurrency, naming, and nesting.
npm install --save taskgroup
// Import
var TaskGroup = require('taskgroup').TaskGroup;
// Create our new group
var group = new TaskGroup();
// Define what should happen once the group has completed
group.once('complete', function(err,results){
// Log the error that has occured
console.log(err);
// => null
// Log the results that our group received from the executing items
console.log(JSON.stringify(results));
/* =>
[
[null, 'first', 'task'],
[null, 'second task'],
[null, [
[null, 'sub second task'],
[null, 'sub first', 'task']
]]
]
*/
});
// Add an asynchronous task that gives the result to the completion callback
group.addTask(function(complete){
setTimeout(function(){
complete(null, 'first', 'task');
},500);
});
// Add a synchronous task that returns the result
// Errors should be returned, though if an error is thrown we will catch it
group.addTask(function(){
return 'second task';
});
// Add a sub-group to our exiting group
group.addGroup(function(addGroup,addTask){
// Tell this sub-group to execute in parallel (all at once) by setting its concurrency to unlimited
// by default the concurrency for all groups is set to 1
// which means that they execute in serial fashion (one after the other, instead of all at once)
this.setConfig({concurrency:0});
// Add an asynchronous task that gives its result to the completion callback
addTask(function(complete){
setTimeout(function(){
complete(null, 'sub first', 'task');
},500);
});
// Add a synchronous task that returns its result
addTask(function(){
return 'sub second task';
});
});
// Execute our group
group.run();
new require('taskgroup').TaskGroup()
constructor(name?,fn?)
- create our new group, the arguments name
and fn
are optional, refer to their entries in configurationsetConfig(config)
- set the configuration for the group, returns chainaddTask(args...)
- create a new task item with the arguments and adds it to the group, returns the new task itemaddGroup(args...)
- create a new group item with the arguments and adds it to the group, returns the new group itemgetTotals()
- returns counts for the following {running,remaining,completed,total}
clear()
- remove the remaining items to be executedpause()
- pause the execution of the itemsstop()
- clear and pauseexit(err)
- stop and complete, err
if specified is sent to the completion event when firedcomplete()
- will fire the completion event if we are already complete, useful if you're binding your listeners after runrun()
- start/resume executing the items, returns chainname
, no default - allows us to assign a name to the group, useful for debuggingfn(addGroup,addTask,complete?)
, no default - allows us to use an inline and self-executing style for defining groups, useful for nestingconcurrency
, defaults to 1
- how many items shall we allow to be run at the same time, set to 0
to allow unlimitedpauseOnError
, defaults to true
- if an error occurs in one of our items, should we stop executing any remaining items?
false
will continue with execution with the other items even if an item experiences an errorrun()
- fired just before we execute the itemscomplete(err, results)
- fired when all our items have completedtask.run(task)
- fired just before a task item executestask.complete(task, err, args...)
- fired when a task item has completedgroup.run(group)
- fired just before a group item executesgroup.complete(group, err, results)
- fired when a group item has completeditem.run(item)
- fired just before an item executes (fired for both sub-tasks and sub-groups)item.complete(item, err, args...)
- fired when an item has completed (fired for both sub-task and sub-groups)new require('taskgroup').Task()
constructor(name?,fn?)
- create our new task, the arguments name
and fn
are optional though fn
must be set at some point, refer to their entries in configurationsetConfig(config)
- set the configuration for the group, returns chaincomplete()
- will fire the completion event if we are already complete, useful if you're binding your listeners after runrun()
- execute the taskname
, no default - allows us to assign a name to the group, useful for debuggingfn(complete?)
, no default - must be set at some point, it is the function to execute for the task, if it is asynchronous it should use the completion callback providedargs
, no default - an array of arguments that you would like to precede the completion callback when executing fn
run()
- fired just before we execute the taskcomplete(err, args...)
- fired when the task has completedYou can discover the history inside the History.md file
Licensed under the incredibly permissive MIT License
Copyright © 2013+ Bevry Pty Ltd
Copyright © 2011-2012 Benjamin Arthur Lupton
v3.1.2 2013 April 6
getTotals()
to TaskGroup
complete()
to Task
FAQs
Group together synchronous and asynchronous tasks and execute them with support for concurrency, naming, and nesting.
The npm package taskgroup receives a total of 55,294 weekly downloads. As such, taskgroup popularity was classified as popular.
We found that taskgroup demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.