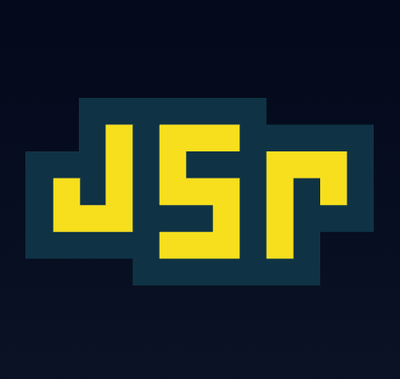
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The tcomb npm package is a library for Node.js and the browser which allows you to check the types of JavaScript values at runtime with a simple and concise syntax. It is useful for enforcing type safety, defining domain models, and creating composable and reusable type checkers.
Type Checking
This feature allows you to define a structure with specific types and create instances that adhere to those types.
const t = require('tcomb');
const Person = t.struct({ name: t.String, age: t.Number });
const person = Person({ name: 'Giulio', age: 43 });
Function Argument Validation
This feature enables you to validate function arguments to ensure they are of the expected type.
const t = require('tcomb');
function sum(a, b) {
t.Number(a);
t.Number(b);
return a + b;
}
sum(1, 2);
Subtyping
This feature allows you to create subtypes based on existing types with additional constraints.
const t = require('tcomb');
const Positive = t.subtype(t.Number, n => n >= 0);
const positiveNumber = Positive(10);
Refinement
This feature is similar to subtyping but is specifically for refining types to a more specific subset.
const t = require('tcomb');
const Integer = t.refinement(t.Number, n => n % 1 === 0);
const integerNumber = Integer(42);
Enums
This feature allows you to define a set of constants and ensure values match one of those constants.
const t = require('tcomb');
const Role = t.enums({ Admin: 'Admin', User: 'User' });
const userRole = Role('User');
Prop-types is a library for React that is used to document the intended types of properties passed to components. It is similar to tcomb in that it provides runtime type checking, but it is specifically tailored for React's component props.
io-ts is a TypeScript runtime type system for IO decoding/encoding. It is similar to tcomb in providing runtime type checking and validation, but it leverages TypeScript's type system for added compile-time safety.
Joi is an object schema description language and validator for JavaScript objects. It provides a powerful and expressive way to define and validate data structures. It is similar to tcomb in terms of validation capabilities but has a different API and is often used for validating API input.
Ajv is a JSON schema validator. It validates data against JSON Schema standards and is similar to tcomb in terms of validation. However, it uses a JSON-based schema definition rather than a programmatic API.
tcomb is a library for Node.js and the browser which allows you to check the types of JavaScript values at runtime with a simple and concise syntax. It's great for Domain Driven Design and for adding safety to your internal code.
"Si vis pacem, para bellum" - (Vegetius 5th century)
var t = require('tcomb');
// a user defined type
var Integer = t.refinement(t.Number, function (n) { return n % 1 === 0; }, 'Integer'); // <= give a name for better debug messages
// a struct
var Person = t.struct({
name: t.String, // required string
surname: t.maybe(t.String), // optional string
age: Integer, // required integer
tags: t.list(t.String) // a list of strings
}, 'Person'); // <= give a name for better debug messages
// methods are defined as usual
Person.prototype.getFullName = function () {
return `${this.name} ${this.surname}`;
};
// an instance of Person (the keyword new is optional)
var person = new Person({
name: 'Giulio',
surname: 'Canti',
age: 41,
tags: ['js developer', 'rock climber']
});
3KB gzipped.
Write complex domain models in a breeze and with a small code footprint. Supported types:
Blog posts:
All models are type checked:
var person = new Person({
name: 'Giulio',
// missing required field "age"
tags: ['js developer', 'rock climber']
});
Output to console:
[tcomb] Invalid value undefined supplied to Person/age: Number
See "Debugging with Chrome DevTools" section for details.
Instances are immutables using Object.freeze
. This means you can use standard JavaScript objects and arrays. You don't have to change how you normally code. You can update an immutable instance with the provided update(instance, spec)
function:
var person2 = Person.update(person, {
name: {$set: 'Guido'}
});
where spec
is an object contaning commands. The following commands are compatible with the Facebook Immutability Helpers:
$push
$unshift
$splice
$set
$apply
$merge
See Updating immutable instances in the docs for details.
Object.freeze
calls and asserts are executed only in development and stripped out in production (using process.env.NODE_ENV = 'production'
tests).
You can customize the behavior when an assert fails leveraging the power of Chrome DevTools.
// use the default...
t.fail = function fail(message) {
throw new TypeError('[tcomb] ' + message); // set "Pause on exceptions" on the "Sources" panel
};
// .. or define your own behavior
t.fail = function fail(message) {
debugger; // starts the Chrome DevTools debugger
throw new TypeError(message);
};
All models are inspectable at runtime. You can read and reuse the informations stored in your types (in a meta
static property). See The meta object in the docs for details.
Libraries exploiting tcomb's RTI:
Encodes / decodes your domain models to / from JSON for free.
// this example uses ES6 syntax
const result = t.match(1,
t.String, () => 'a string',
t.Number, () => 'a number'
);
console.log(result); // => 'a number'
func
combinator ideas and documentation.The MIT License (MIT)
FAQs
Type checking and DDD for JavaScript
The npm package tcomb receives a total of 872,977 weekly downloads. As such, tcomb popularity was classified as popular.
We found that tcomb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.