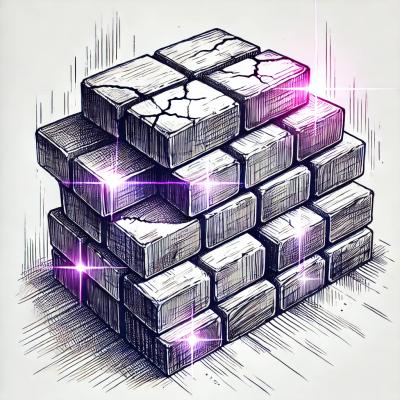
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Teabot allows you to create highly interactive Telegram bot for Node.js with some additional cool features.
npm install teabot
var TeaBot = require('teabot');
var token = 'YOUR_TELEGRAM_BOT_TOKEN';
var name = 'YOUR_TELEGRAM_BOT_NAME';
var Bot = new TeaBot(token, name);
Bot.defineCommand(function(dialog) {
var message = dialog.message;
dialog.sendMessage('Echo: ' + message.text);
});
Bot.startPooling();
Bot object stores all commands, actions, settings, and also all the dialogues bot.
var TeaBot = require('teabot');
var Bot = new TeaBot(token, name);
Commands always start with /.
var Bot = new TeaBot(token, name);
Bot
.defineCommand(['/start', '/help'], function(dialog) {
var message = dialog.message;
dialog.sendMessage('Hi there. This is a ' + message.getCommand() + ' command.');
})
.defineCommand(function(dialog) {
dialog.sendMessage('Send me /help for more information.');
});
Actions are singly linked list of unlimited length.
The first action is always set using defineAction
, and all others using the defineSubAction
in the previous action.
var Bot = new TeaBot(token, name);
Bot
.defineCommand('/start', function(dialog) {
dialog.startAction('/start').sendMessage('This is /start command');
})
.defineCommand(function(dialog) {
dialog.sendMessage('Send me /help for more information.');
});
Bot
.defineAction('/start', function(dialog) {
dialog.startAction('subaction 1').sendMessage('This is /start action');
}, function(action) {
action.defineSubAction('subaction 1', function(dialog) {
dialog.startAction('subaction 2').sendMessage('This is subaction 1');
}, function(action) {
action.defineSubAction('subaction 2', function(dialog) {
dialog.endAction().sendMessage('This is subaction 2');
})
})
});
Depending on what type of connection with Telegram is used (webhook or long pooling), there are 2 methods to start the bot.
To work with webhook.
To work with long pooling.
Dialog object stores bot current dialogue with the user, as well as commands for communication.
It can be obtained in defineCommand
, defineAction
, defineSubAction
callbacks, or directly from Bot object.
It is an object that contains a list of actions in the form of linked list.
Checks whether the user is in a state of action, and if so action will returned, otherwise false.
Start the action. Then all processes occur in defineAction
or defineSubAction
callbacks.
defineAction
or defineSubAction
for start.Ends the action and clears dialog.tempData
.
It is an object that can store user data (such as the bot settings).
Gets user data on the property name.
Sets the user data.
Deletes user data on the property name.
Clears the user data.
It is an object that can store temporary data to be transmitted between the actions.
Deleted automatically when calling endAction()
.
Gets temporary data on the property name.
Sets the temporary data.
Deletes temporary data on the property name.
Clears the temporary data.
All methods return a Promise
, unless otherwise indicated. All methods are sent to the current chat/user.
Send text message.
Forward messages of any kind.
Send photo.
file_id
. See InputFile object for more info.Send audio.
file_id
. See InputFile object for more info.Send document.
file_id
. See InputFile object for more info.Send .webp stickers.
file_id
. See InputFile object for more info.Send video.
file_id
. See InputFile object for more info.Send voice.
file_id
. See InputFile object for more info.Send location.
Send chat action.
typing
for text messages, upload_photo
for photos, record_video
or upload_video
for videos, record_audio
or upload_audio
for audio files, upload_document
for general files, find_location
for location data.
Use this method to get a list of profile pictures for a user.
Custom keyboard.
Hide custom keyboard.
If you just want to hide the keyboard, then do this:
dialog.setKeyboard().sendMessage('Text');
//or
dialog.setKeyboard(true);
dialog.sendMessage('Text');
If you want to hide the keyboard to specific users only, then do this:
dialog.setKeyboard(true, true).sendMessage('Text');
//or
dialog.setKeyboard(true, true);
dialog.sendMessage('Text');
If buffer:
var inputFile = {
buffer: new Buffer(),
fileName: 'file.png'
};
If stream:
var inputFile = {
stream: fs.createReadStream('file.png'),
fileName: 'file.png'
};
If path:
var inputFile = 'file.png';
If file_id
:
var inputFile = 'file_id';
//or
var inputFile = {fileId: 'file_id'};
Message object stores processed incoming message, as well as its original copy.
It can be obtained from dialog.message
.
Returns the type of message: text
, audio
, document
, photo
, sticker
, video
, contact
, location
or other
.
If is message is command returns true
else false
.
Returns command.
It returns the rest of the message, if it contains a command or the entire message.
By default, all data is stored in memory, but for synchronization between servers or nodes, you may need a database.
By default key = 'app:teabot'
.
var redis = require('redis');
var client = redis.createClient();
var config = {
db: {
type: 'redis',
client: client,
key: 'bot:telegram'
}
};
var Bot = new TeaBot(token, name, config);
By default key = {ns: 'app', set: 'teabot'}
.
var aerospike = require('aerospike');
var client = aerospike.client({
hosts: [{
addr: '127.0.0.1',
port: 4000,
}]
}).connect(function(response) {
if (response.code == 0) {
console.log('Connection to Aerospike cluster succeeded!');
}
});
var config = {
db: {
type: 'aerospike',
client: client,
key: {
ns: 'bot', set: 'telegram'
}
}
};
var Bot = new TeaBot(token, name, config);
TeaBot has built-in support analytics from botan.io.
By default, all events are sent automatically at the defineCommand
, defineAction
, defineSubAction
.
And it looks like this:
But you can send they manually using the Bot.track, if you specify manualMode
property to true.
var config = {
analytics: {
key: 'KEY',
manualMode: true
}
};
var Bot = new TeaBot(token, name, config);
Bot
.defineCommand(['/start', '/help'], function(dialog) {
var message = dialog.message;
dialog.sendMessage('Hi there. This is a ' + message.getCommand() + ' command.');
Bot.track(dialog.userId, message, message.getCommand());
})
.defineCommand(function(dialog) {
dialog.sendMessage('Send me /help for more information.');
Bot.track(dialog.userId, message, 'Default');
});
It's weird but you can even let your users create their own command!
Note: Currently it'll correctly work only with default memory storage in single node environment.
Bot
.defineCommand('/add', function(dialog) {
dialog.startAction('/add').sendMessage('Send me new command');
})
.defineCommand('/cancel', function(dialog) {
dialog.endAction().sendMessage('Ok, now you can try something else.');
})
.defineCommand(function(dialog) {
dialog.sendMessage('Send me /help for more information.');
});
Bot
.defineAction('/add', function(dialog) {
dialog.setTempData('command', dialog.message.text);
dialog.startAction('message').sendMessage('Send me message for new command');
}, function(action) {
action.defineSubAction('message', function(dialog) {
var message = dialog.message.text;
Bot.defineCommand(dialog.getTempData('command'), function(dialog) {
dialog.sendMessage(message);
});
dialog.sendMessage('New command ' + dialog.getTempData('command') + ' was successfully added!');
dialog.endAction();
})
});
Coming soon.
The MIT License (MIT)
Copyright (c) 2015 Alexey Bystrov
FAQs
TeaBot - a way to build interactive Telegram bots.
The npm package teabot receives a total of 2 weekly downloads. As such, teabot popularity was classified as not popular.
We found that teabot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.