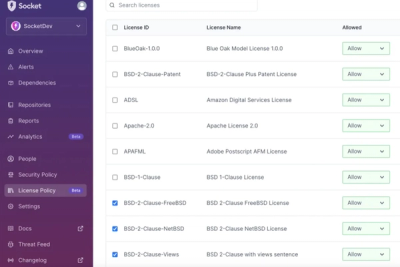
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
ted-crushinator-helpers
Advanced tools
JavaScript methods to produce Crushinator-optimized image URLs.
crushinator.crush('http://images.ted.com/image.jpg', { width: 320 })
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=320'
The Crushinator helpers are distributed in UMD and have no other dependencies, so they can be imported as an AMD or as a CommonJS (Node) module. If no module system is available, the library will occupy the crushinator
namespace in your app's global (e.g. window.crushinator
).
npm install ted-crushinator-helpers
bower install ted-crushinator-helpers
Code in dist/crushinator.umd.min.js
can be copied to your application.
This library provides the following methods:
crush(url, options)
whose options can include:
uncrush(url)
crushable(url)
Create a Crushinator-optimized image URL.
Method signature:
crushinator.crush ( url , [ options = {} ] )
Parameters:
url
(string, required) - URL of the image you would like to be crushed.options
(object, optional) - Crushinator image options as a Plain Old JavaScript Object. Available options:
width
(number) - Target image width in pixels.height
(number) - Target image height in pixels.quality
(number) - Image quality as a percentage (0-100).crop
(object) - Crop configuration. (See details below.)Example use:
crushinator.crush('http://images.ted.com/image.jpg', { width: 320 })
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=320'
Crushinator only operates on images hosted on whiteslisted domains. If you use the crush
method on an image outside of that whitelist, it will simply return the original URL:
crushinator.crush('http://celly.xxx/waffles.jpg', { width: 320 })
// => 'http://celly.xxx/waffles.jpg'
This is helpful for dealing with dynamic image URLs.
Some more detail and examples for individual Crushinator helper options:
Number; target image width in pixels.
If the original image is wider than this value, Crushinator's version will be resized to match it.
Width and height are treated as a maximum. If width and height are both specified, Crushinator will resize the image to fit into a space of those dimensions while respecting its original aspect ratio.
If neither the width nor the height are specified, the original image dimensions will be used.
If the height is specified but not the width, the width of the resized image will respect the original aspect ratio as the image is resized by height.
Example:
crushinator.crush('http://images.ted.com/image.jpg', { width: 320 })
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=320'
Number; target image height in pixels.
If the original image is taller than this value, Crushinator's version will be resized to match it.
Height and width are treated as a maximum. If height and width are both specified, Crushinator will resize the image to fit into a space of those dimensions while respecting its original aspect ratio.
If neither the height nor the width are specified, the original image dimensions will be used.
If the width is specified but not the height, the height of the resized image will respect the original aspect ratio as the image is resized by width.
Example:
crushinator.crush('http://images.ted.com/image.jpg', { height: 240 })
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?h=240'
Number; image quality as a percentage (0
-100
).
If this value is omitted, a default image quality of 75% is used.
Example:
crushinator.crush('http://images.ted.com/image.jpg', { quality: 93 })
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?quality=93'
Crop configuration options are passed in as an object with the following properties:
width
(number) - Width of cropped section (in pixels).height
(number) - Height of cropped section (in pixels).x
(number) - Coordinate at which to start crop (pixels from left).y
(number) - Coordinate at which to start crop (pixels from top).afterResize
(boolean) - true
if you want to crop the resized image rather than the original, false
or omitted otherwise.By default, crop configuration will be applied to the original image, which will then be resized in accord with the height
and width
options. The afterResize
option allows you to configure Crushinator to instead apply the crop after resizing the image.
Example:
crushinator.crush('http://images.ted.com/image.jpg', {
width: 640,
height: 480,
crop: {
width: 200, height: 100,
x: 50, y: 25,
afterResize: true
}
})
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=640&h=480&c=200%2C100%2C50%2C25'
The above example would resize the original image to 640x480 and then take a 200x100 crop of the resized image, starting at 50x25.
Crop configuration options can also be sent in hyphenated form:
crushinator.crush('http://images.ted.com/image.jpg', {
width: 640,
height: 480,
'crop-width': 200, 'crop-height': 100,
'crop-x': 50, 'crop-y': 25,
'crop-afterResize': true
})
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=640&h=480&c=200%2C100%2C50%2C25'
The query
option can be used to append custom query parameters to the Crushinator URL:
crushinator.crush('http://images.ted.com/image.jpg', {
width: 200,
query: { c: '100,100' }
})
// => 'https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=200&c=100%2C100'
This allows you to directly apply any of Crushinator's query parameters instead of using this helper's wrapper API.
Restore a previously crushed URL to its original form.
Method signature:
crushinator.uncrush ( url )
Parameters:
url
(string, required) - URL of previously crushed image.Example:
crushinator.uncrush('https://tedcdnpi-a.akamaihd.net/r/images.ted.com/image.jpg?w=320')
// => 'https://images.ted.com/image.jpg'
Test to see if an image URL is allowed by Crushinator's whitelist.
Method signature:
crushinator.crushable ( url )
Parameters:
url
(string, required) - URL of image to check.Returns true
if the image's host is in Crushinator's whitelist, false
otherwise.
Examples:
crushinator.crushable('http://images.ted.com/image.jpg')
// => true
crushinator.crushable('http://celly.xxx/waffles.jpg')
// => false
Use NVM to make sure you have the correct Node version installed for local development.
git clone git@github.com:tedconf/js-crushinator-helpers.git
npm install
npm test
will lint and test the library.
npm run build
to produce new JS in the dist
directorypackage.json
and commitgit tag
the new semvernpm publish
the updated node moduleFAQs
JS methods to produce crushinator'd image URLs.
The npm package ted-crushinator-helpers receives a total of 1 weekly downloads. As such, ted-crushinator-helpers popularity was classified as not popular.
We found that ted-crushinator-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.