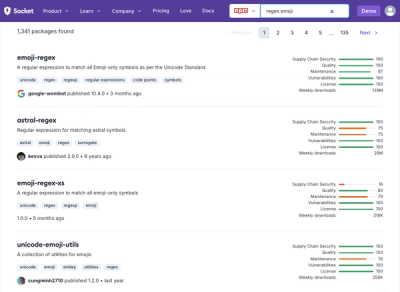
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
test-client
Advanced tools
test-client
is a small HTTP assertion library.
'use strict'
const http = require('http')
const Client = require('test-client')
async function test () {
const server = http.createServer((request, response) => {
response.setHeader('content-type', 'application/json')
response.end(JSON.stringify({x: 2}))
})
const client = new Client(server)
const response = await client
.get('/test')
.type('json')
.accept('json')
.set('user-agent', 'smith')
.send({x: 1})
response
.assert(200, {x: 2})
.assert('content-type', /json/)
}
npm install test-client
app
can be a koa/express app, an http.Server
, or anything
with a .listen()
method.
Returns a Request
with the corresponding path
and method
. method
can be
any of the values in http.METHODS
.
There is a convenience method proxying .request(…)
for each method in
http.METHODS
. Returns a Request
.
A CookieJar
instance for storing cookies.
Set a request header by key and value. Returns the request.
Set headers from an object containing header key/value pairs. Returns the request.
Set the accept
header from a content-type or extension via
mime-types
. Returns the request.
Set the content-type
header from a content-type or extension via
mime-types
. Returns the request.
Send the request with no body. Returns a promise that resolves as a Response
.
Send the request with the specified body
. If body
is an object, it's JSON
encoded and content-type
is set to application/json
. If body
is a stream,
it's piped to the request. Returns a promise that resolves as a Response
.
The numeric status code from the response.
An object containing header values from the response.
If the response is JSON as indicated by the content-type
, the decoded
response. Otherwise, the response string.
Assert the HTTP status value. Returns the response.
Assert the repsonse body value. Returns the response.
Assert that the response body matches a RegExp. Returns the response.
Assert the response body as a JSON object. Returns the response.
Assert the HTTP status and response body value. Returns the response.
Assert the HTTP status value and the response body matches a RegExp. Returns the response.
Assert an HTTP header value. Returns the response.
Assert an HTTP header matches a RegExp. Returns the response.
FAQs
HTTP assertions for node
The npm package test-client receives a total of 7 weekly downloads. As such, test-client popularity was classified as not popular.
We found that test-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.