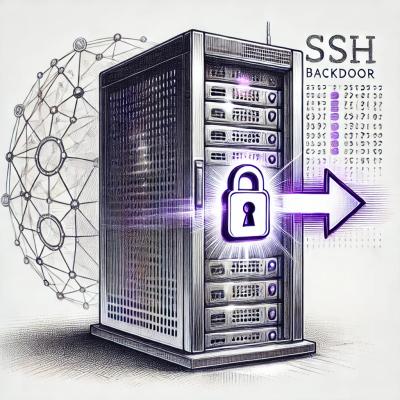
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
text-processor
Advanced tools
A javascript library combining profanity filtering and sentiment analysis
TextProcessor is a JavaScript library devveloped for text analysis. It integrates profanity/badwords filtering, sentiment analysis, and toxicity detection capabilities. By leveraging the AFINN-165 wordlist, Emoji Sentiment Ranking, and the Perspective API, TextProcessor offers a robust toolkit for enhancing content moderation and fostering healthier online interactions.
English and French for Profanity and Sentiment Analysis, can be extended. Toxicity Detection works for any language.
npm install text-processor --save
Censor or identify profanity within text inputs automatically.
const TextProcessor = require('text-processor');
const textProcessor = new TextProcessor();
console.log(textProcessor.clean("Don't be an ash0le"));
// Output: "Don't be an ******"
var customTextProcessor = new TextProcessor({ placeHolder: 'x'});
customTextProcessor.clean("Don't be an ash0le"); // Don't be an xxxxxx
textProcessor.addWords('some', 'bad', 'word');
textProcessor.clean("some bad word!") // **** *** ****!
textProcessor.removeWords('hells', 'sadist');
textProcessor.clean("some hells word!"); //some hells word!
This function helps maintain respectful communication by replacing recognized profane words with placeholders.
Evaluate textual sentiment, identifying whether the content is positive, neutral, or negative.
const result = textProcessor.analyzeSentiment('Cats are amazing.');
console.dir(result);
Example output:
{
"score": 3,
"comparative": 1,
"calculation": [{"amazing": 3}],
"tokens": ["cats", "are", "amazing"],
"words": ["amazing"],
"positive": ["amazing"],
"negative": []
}
The output demonstrates a positive sentiment score, reflecting the text's overall positive tone.
var frLanguage = {
labels: { 'stupide': -2 }
};
textProcessor.registerLanguage('fr', frLanguage);
var result = textProcessor.analyzeSentiment('Le chat est stupide.', { language: 'fr' });
console.dir(result); // Score: -2, Comparative: -0.5
Analyze text for toxicity with the Perspective API to maintain constructive discourse. This is developed
const API_KEY = 'your_api_key_here'; // Replace with your Persective API key from Google API Services
textProcessor.analyzeToxicity("Your text to analyzeSentiment", API_KEY)
.then(result => console.log(JSON.stringify(result)))
.catch(err => console.error(err));
Sample output:
{
"attributeScores": {
"TOXICITY": {
"summaryScore": {
"value": 0.021196328,
"type": "PROBABILITY"
}
}
},
"languages": ["en"],
"detectedLanguages": ["en"]
}
This provides a toxicity score, indicating how likely the text is perceived as toxic, aiding in moderating content effectively.
### API
<!-- Generated by documentation.js. Update this documentation by updating the source code. -->
##### Table of Contents
- [constructor](#constructor)
- [isProfane](#isprofane)
- [replaceWord](#replaceword)
- [clean](#clean)
- [addWords](#addwords)
- [removeWords](#removewords)
- [registerLanguage](#registerlanguage)
- [analyzeSentiment](#analyzeSentiment)
- [analyzeToxicity](#analyzetoxicity)
- [tokenize](#tokenize)
- [addLanguage](#addlanguage)
#### constructor
TextProcessor constructor.
Combines functionalities of word filtering and sentiment analysis.
**Parameters**
- `options` **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** TextProcessor instance options. (optional, default `{}`)
- `options.emptyList` **[boolean](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean)** Instantiate filter with no blacklist. (optional, default `false`)
- `options.list` **[array](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)** Instantiate filter with custom list. (optional, default `[]`)
- `options.placeHolder` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Character used to replace profane words. (optional, default `'*'`)
- `options.regex` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Regular expression used to sanitize words before comparing them to blacklist. (optional, default `/[^a-zA-Z0-9|\$|\@]|\^/g`)
- `options.replaceRegex` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Regular expression used to replace profane words with placeHolder. (optional, default `/\w/g`)
- `options.splitRegex` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Regular expression used to split a string into words. (optional, default `/\b/`)
- `options.sentimentOptions` **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** Options for sentiment analysis. (optional, default `{}`)
#### isProfane
Determine if a string contains profane language.
**Parameters**
- `string` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** String to evaluate for profanity.
#### replaceWord
Replace a word with placeHolder characters.
**Parameters**
- `string` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** String to replace.
#### clean
Evaluate a string for profanity and return an edited version.
**Parameters**
- `string` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Sentence to filter.
#### addWords
Add word(s) to blacklist filter / remove words from whitelist filter.
**Parameters**
- `words` **...any**
- `word` **...[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Word(s) to add to blacklist.
#### removeWords
Add words to whitelist filter.
**Parameters**
- `words` **...any**
- `word` **...[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Word(s) to add to whitelist.
#### registerLanguage
Registers the specified language.
**Parameters**
- `languageCode` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Two-digit code for the language to register.
- `language` **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** The language module to register.
#### analyzeSentiment
Performs sentiment analysis on the provided input 'phrase'.
**Parameters**
- `phrase` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Input phrase.
- `opts` **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** Options. (optional, default `{}`)
- `callback` **[function](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function)** Optional callback.
Returns **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
#### analyzeToxicity
Analyzes the toxicity of a given text using the Perspective API.
**Parameters**
- `text` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Text to analyze.
- `apiKey` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** API key for the Perspective API.
Returns **[Promise](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise)** A promise that resolves with the analysis result.
#### tokenize
Remove special characters and return an array of tokens (words).
**Parameters**
- `input` **[string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Input string
Returns **[array](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)** Array of tokens
#### addLanguage
Registers the specified language
**Parameters**
- `languageCode` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Two-digit code for the language to register
- `language` **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)** The language module to register
#### getLanguage
Retrieves a language object from the cache,
or tries to load it from the set of supported languages
**Parameters**
- `languageCode` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Two-digit code for the language to fetch
#### getLabels
Returns AFINN-165 weighted labels for the specified language
**Parameters**
- `languageCode` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Two-digit language code
Returns **[Object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)**
#### applyScoringStrategy
Applies a scoring strategy for the current token
**Parameters**
- `languageCode` **[String](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String)** Two-digit language code
- `tokens` **[Array](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array)** Tokens of the phrase to analyze
- `cursor` **int** Cursor of the current token being analyzed
- `tokenScore` **int** The score of the current token being analyzed
## How it works
### AFINN
AFINN is a list of words rated for valence with an integer between minus five (negative) and plus five (positive). Sentiment analysis is performed by cross-checking the string tokens (words, emojis) with the AFINN list and getting their respective scores. The comparative score is simply: `sum of each token / number of tokens`. So for example let's take the following:
`I love cats, but I am allergic to them.`
That string results in the following:
```javascript
{
score: 1,
comparative: 0.1111111111111111,
calculation: [ { allergic: -2 }, { love: 3 } ],
tokens: [
'i',
'love',
'cats',
'but',
'i',
'am',
'allergic',
'to',
'them'
],
words: [
'allergic',
'love'
],
positive: [
'love'
],
negative: [
'allergic'
]
}
In this case, love has a value of 3, allergic has a value of -2, and the remaining tokens are neutral with a value of 0. Because the string has 9 tokens the resulting comparative score looks like:
(3 + -2) / 9 = 0.111111111
This approach leaves you with a mid-point of 0 and the upper and lower bounds are constrained to positive and negative 5 respectively (the same as each token! 😸). For example, let's imagine an incredibly "positive" string with 200 tokens and where each token has an AFINN score of 5. Our resulting comparative score would look like this:
(max positive score * number of tokens) / number of tokens
(5 * 200) / 200 = 5
Tokenization works by splitting the lines of input string, then removing the special characters, and finally splitting it using spaces. This is used to get list of words in the string.
The MIT License (MIT)
Copyright (c) 2013 Michael Price
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
A javascript library combining profanity filtering and sentiment analysis
The npm package text-processor receives a total of 0 weekly downloads. As such, text-processor popularity was classified as not popular.
We found that text-processor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.