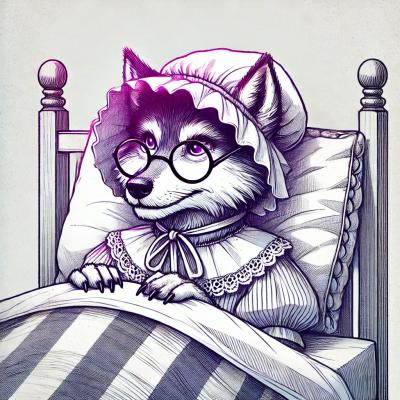
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
the-query-builder
Advanced tools
const sql = (new QueryBuilder())
.select('id', 'name', 'age')
.from('table')
.getSQL();
Generated SQL
SELECT id, name, age
FROM table
Column alias
const sql = (new QueryBuilder())
.select({
id: 'product_id',
title: 'product_title'
})
.from('table')
.getSQL();
Generated SQL:
SELECT id AS product_id, title AS product_title
FROM table
Distinct
const sql = (new QueryBuilder())
.distinct()
.select('category')
.from('table')
.getSQL();
Generated SQL:
SELECT DISTINCT category
FROM table
selectRaw
const sql = (new QueryBuilder())
.selectRaw('SELECT one, two, three')
.from('table')
.getSQL();
Generated SQL
SELECT one, two, three
FROM table
By default, all columns will be selected from table.
const sql = (new QueryBuilder())
.from('table')
.getSQL();
Generated SQL
SELECT *
FROM table
const sql = (new QueryBuilder())
.from('table')
.getSQL();
Set multiple FROM
const sql = (new QueryBuilder())
.from('table')
.from('another_table')
.getSQL();
Generated SQL
SELECT *
FROM table,
another_table
const sql = (new QueryBuilder())
.from('table')
.leftJoin('another_table', 'id', 'another_id')
.getSQL();
Generated SQL
SELECT *
FROM table
LEFT JOIN another_table ON id = another_id
const sql = (new QueryBuilder())
.from('table')
.rightJoin('another_table', 'id', 'another_id')
.getSQL();
Generated SQL
SELECT *
FROM table
RIGHT JOIN another_table ON id = another_id
const sql = (new QueryBuilder())
.from('table')
.innerJoin('another_table', 'id', 'another_id')
.getSQL();
Generated SQL
SELECT *
FROM table
INNER JOIN another_table ON id = another_id
const sql = (new QueryBuilder())
.from('table')
.joinRaw('LEFT JOIN another_table t ON id = another_id')
.getSQL();
Generated SQL
SELECT *
FROM table
LEFT JOIN another_table t ON id = another_id
const sql = (new QueryBuilder())
.from('table')
.join(qb => {
qb
.leftJoin('another_table')
.on('id', 'another_id')
.and('other_column', 'something')
.or('other_column', 'other_thing')
})
.getSQL();
Generated SQL
SELECT *
FROM table
LEFT JOIN another_table ON id = another_id AND other_column = 'something' OR other_column = 'other_thing'
const sql = (new QueryBuilder())
.where('id', 10)
.from('table')
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id = 10
Set multiple WHERE
const sql = (new QueryBuilder())
.where('id', 10)
.where('status', 'published')
.from('table')
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id = 10
AND status = 'published'
const sql = (new QueryBuilder())
.from('table')
.whereIn('id', [10, 100])
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id IN (10, 100)
const sql = (new QueryBuilder())
.from('table')
.whereBetween('id', 10, 100)
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id BETWEEN 10 AND 100
const sql = (new QueryBuilder())
.from('table')
.whereLike('status', 'something')
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE status LIKE '%something%'
const sql = (new QueryBuilder())
.from('table')
.whereIsNull('id')
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id IS NULL
const sql = (new QueryBuilder())
.from('table')
.whereIn('id', qb => {
qb
.select('another_id')
.from('another_table')
.where('category', 'something')
})
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE id IN (SELECT another_id FROM another_table WHERE category = 'something')
const sql = (new QueryBuilder())
.from('table')
.where('status', 'published')
.orWhere(qb => {
qb
.where('status', 'draft')
.where('writing', 'locked')
})
.getSQL();
Generated SQL
SELECT *
FROM table
WHERE status = 'published'
OR (status = 'draft' AND writing = 'locked')
const sql = (new QueryBuilder())
.from('table')
.orderBy('id')
.getSQL();
Generated SQL
SELECT *
FROM table
ORDER BY id ASC
Order by multiple columns
const sql = (new QueryBuilder())
.from('table')
.orderBy('id')
.orderBy('column_one', 'DESC')
.getSQL();
Generated SQL
SELECT *
FROM table
ORDER BY id ASC, column_one DESC
const sql = (new QueryBuilder())
.from('table')
.groupBy('id')
.getSQL();
Generated SQL
SELECT *
FROM table
GROUP BY id
Having
const sql = (new QueryBuilder())
.from('table')
.groupBy('id')
.having('id', '>', 10)
.getSQL();
Generated SQL
SELECT *
FROM table
GROUP BY id
HAVING id > 10
Limit
const sql = (new QueryBuilder())
.from('table')
.limit(10)
.getSQL();
Generated SQL
SELECT *
FROM table
LIMIT 10
Offset
const sql = (new QueryBuilder())
.from('table')
.limit(10)
.offset(10)
.getSQL();
Generated SQL
SELECT *
FROM table
LIMIT 10 OFFSET 10
Union
const builder1 = (new QueryBuilder())
.select('something')
.from('table');
const sql = (new QueryBuilder())
.select('something_else')
.from('another_table')
.union(builder1)
.getSQL();
Generated SQL
SELECT something_else
FROM another_table
UNION
SELECT something
FROM table
Union ALL
const builder1 = (new QueryBuilder())
.select('something')
.from('table');
const builder2 = (new QueryBuilder())
.select('another_thing')
.from('another_table');
const sql = (new QueryBuilder())
.select('something_else')
.from('some_table')
.unionAll(builder1, builder2)
.getSQL();
Generated SQL
SELECT something_else
FROM some_table
UNION ALL
SELECT something
FROM table
UNION ALL
SELECT another_thing
FROM another_table
FAQs
SQL Query Builder
The npm package the-query-builder receives a total of 1 weekly downloads. As such, the-query-builder popularity was classified as not popular.
We found that the-query-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.