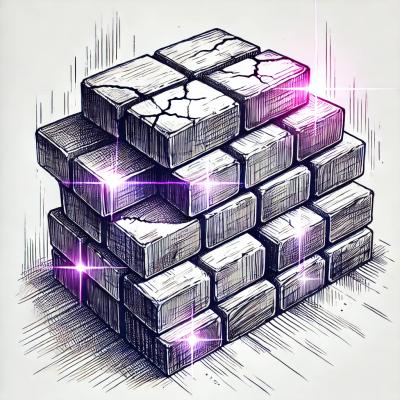
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
think-bayes
Advanced tools
An algorithm framework of probability and statistics for browser and Node.js environment.
An algorithm collection of probability and statistics for browser and Node.js environment.
In progress...
适用于 浏览器 和 Node.js 环境的概率统计算法集(非正式版本,功能亟待完善,努力 coding 中...)
yarn add think-bayes # OR npm i --save think-bayes
Let us resolve the cookie problem by using the class Suite
:
import { Suite } from 'think-bayes';
class Cookie extends Suite {
mixes = {
Bowl1: {
vanilla: 0.75,
chocolate: 0.25,
},
Bowl2: {
vanilla: 0.5,
chocolate: 0.5,
},
};
likelihood(data, hypo) {
const mix = this.mixes[hypo];
const like = mix[data];
return like;
}
}
const hypos = ['Bowl1', 'Bowl2'];
const pmf = new Cookie(hypos);
pmf.update('vanilla');
const result = pmf.render();
console.log(result); // [ [ 'Bowl1', 0.6 ], [ 'Bowl2', 0.4 ] ]
// You can also print the result as a table
pmf.print();
// | Value | Prob |
// |-------|------|
// | Bowl1 | 0.6 |
// | Bowl2 | 0.4 |
In addition, here are some simple demos you can refer directly to resolve some classic problems of probability and statistics.
This library provides some ES Classes following for calculations related to probability and statistics.
These classes can be imported by the same way following:
import { Pmf, Cdf, Pdf, Suite } from 'think-bayes';
An base class for generation an object contains a dictionary.
@Params:
param | type | description |
---|---|---|
values | string | array |
name | string | sequence of values |
@Methods:
Initializes with a sequence of equally-likely values.
@Params:
param | type | description |
---|---|---|
values | array | sequence of values |
Initializes with a map from value to probability.
@Params:
param | type | description |
---|---|---|
values | map | map from value to probability |
Initializes with a Pmf.
@Params:
param | type | description |
---|---|---|
values | pmf | Pmf object |
Throw an error.
Gets an unsorted sequence of values.
Note: One source of confusion is that the keys of this
dictionary are the values of the Hist/Pmf, and the
values of the dictionary are frequencies/probabilities.
Gets an unsorted sequence of (value, freq/prob) pairs.
Sets the freq/prob associated with the value x.
@Params:
param | type | description |
---|---|---|
value | any | number value or case name |
prob | number | number freq or prob |
Increments the freq/prob associated with the value x.
@Params:
param | type | description |
---|---|---|
x | any | number value or case name |
term | number | how much to increment by |
Scales the freq/prob associated with the value x.
@Params:
param | type | description |
---|---|---|
x | any | number value or case name |
factor | number | how much to multiply by |
Removes a value.
Throws an exception if the value is not there.
@Params:
param | type | description |
---|---|---|
value | any | value to remove |
Returns the total of the frequencies/probabilities in the map.
Returns the largest frequency/probability in the map.
Returns a copy.
Make a shallow copy of d. If you want a deep copy of d,
use one method to deep clone the whole object.
@Params:
param | type | description |
---|---|---|
name | string | string name for the new Hist |
@Returns: new object
Multiplies the values by a factor.
@Params:
param | type | description |
---|---|---|
factor | number | what to multiply by |
@Returns: new object
Log transforms the probabilities.
Removes values with probability 0.
Normalizes so that the largest logprob is 0.
@Params:
param | type | description |
---|---|---|
m | number | how much to shift the ps before exponentiating |
Exponentiates the probabilities.
If m is un-exist, normalizes so that the largest prob is 1.
@Params:
param | type | description |
---|---|---|
m | number | how much to shift the ps before exponentiating |
Gets the dictionary.
Sets the dictionary.
@Params:
param | type | description |
---|---|---|
d | map | object |
Generates a sequence of points suitable for plotting.
@Returns: array of [sorted value sequence, freq/prob sequence]
Prints the values and freqs/probs in ascending order.
@Params:
param | type | description |
---|---|---|
indent |
Represents a probability mass function.
Values can be any hashable type; probabilities are floating-point.
Pmfs are not necessarily normalized.
@Params:
param | type | description |
---|---|---|
values | string | array |
name | string | sequence of values |
@Methods:
Important: This class inherits from DictWrapper, so you can use all methods of the parent class.
Gets the probability associated with the value x.
@Params:
param | type | description |
---|---|---|
x | any | number value |
probDefault | number | value to return if the key is not there |
@Returns: probability
Gets probabilities for a sequence of values.
@Params:
param | type | description |
---|---|---|
xs | array | a sequence of values |
@Returns: array of probabilities
Makes a cdf.
@Params:
param | type | description |
---|---|---|
name | string | the name for new cdf |
@Returns: one new cdf
Calculate the probability while the value is greater than x.
@Params:
param | type | description |
---|---|---|
x | number |
@Returns: probability
Calculate the probability while the value is less than x.
@Params:
param | type | description |
---|---|---|
x | number |
@Returns: probability
Normalizes this PMF so the sum of all probs is fraction.
@Params:
param | type | description |
---|---|---|
fraction | number | what the total should be after normalization |
@Returns: the total probability before normalizing
Chooses a random element from this PMF.
@Returns: float value from the pmf
Computes the mean of a PMF.
@Returns: float mean
Computes the variance of a PMF.
@Params:
param | type | description |
---|---|---|
miu | number | the point around which the variance is computed; if omitted, computes the mean |
@Returns: float variance
Returns the value with the highest probability.
@Returns: float probability
Computes the central credible interval.
If percentage=90, computes the 90% CI.
@Params:
param | type | description |
---|---|---|
percentage | number | float between 0 and 100 |
@Returns: sequence of two floats, low and high
Computes the Pmf of the sum of values drawn from self and other.
@Params:
param | type | description |
---|---|---|
other | number | pmf |
@Returns: new pmf
Computes the Pmf of the sum of values drawn from self and other.
@Params:
param | type | description |
---|---|---|
other | pmf | another pmf |
@Returns: new pmf
Computes the Pmf of the sum a constant and values from self.
@Params:
param | type | description |
---|---|---|
other | number | a number |
@Returns: new pmf
Computes the Pmf of the diff of values drawn from self and other.
@Params:
param | type | description |
---|---|---|
other | pmf | another pmf |
@Returns: new pmf
Computes the CDF of the maximum of k selections from this dist.
@Params:
param | type | description |
---|---|---|
k | number | int |
@Returns: new cdf
Represents a cumulative distribution function.
@Params:
param | type | description |
---|---|---|
xs | array | sequence of values |
ps | array | sequence of probabilities |
name | string | string used as a graph label |
@Methods:
Important: This class inherits from DictWrapper, so you can use all methods of the parent class.
Represents a cumulative distribution function.
@Params:
param | type | description |
---|---|---|
name | string | string name for the new cdf |
@Returns: new cdf
Makes a Pmf.
@Params:
param | type | description |
---|---|---|
name | string | string name for the new pmf |
@Returns: new pmf
Returns a sorted list of values.
@Returns: array of values
Returns a sorted sequence of [value, probability] pairs.
@Returns: array of [value, probability] pairs
Add an (x, p) pair to the end of this CDF.
Note: this us normally used to build a CDF from scratch, not
to modify existing CDFs. It is up to the caller to make sure
that the result is a legal CDF.
@Params:
param | type | description |
---|---|---|
x | any | number value or case name |
p | number | number freq or prob |
Adds a term to the xs.
@Params:
param | type | description |
---|---|---|
term | number | how much to add |
@Returns: another cdf
Multiplies the xs by a factor.
@Params:
param | type | description |
---|---|---|
factor | what to multiply by |
@Returns: another cdf
Returns CDF(x), the probability that corresponds to value x.
@Params:
param | type | description |
---|---|---|
x | number | number |
@Returns: float probability
Returns InverseCDF(p), the value that corresponds to probability p.
@Params:
param | type | description |
---|---|---|
p | number | number in the range [0, 1] |
@Returns: number value
Returns the value that corresponds to percentile p.
@Params:
param | type | description |
---|---|---|
p | number | number in the range [0, 100] |
@Returns: number value
Chooses a random value from this distribution.
@Returns: number value
Generates a random sample from this distribution.
@Params:
param | type | description |
---|---|---|
n | number | int length of the sample |
@Returns: array of random values
Computes the mean of a CDF.
@Returns: float mean
Computes the central credible interval.
If percentage=90, computes the 90% CI.
@Params:
param | type | description |
---|---|---|
percentage | number | float between 0 and 100 |
@Returns: sequence of two floats, low and high
Generates a sequence of points suitable for plotting.
An empirical CDF is a step function; linear interpolation can be misleading.
@Returns: array of points
Computes the CDF of the maximum of k selections from this dist.
@Params:
param | type | description |
---|---|---|
k | number | int |
@Returns: new Cdf
Represents a probability density function (PDF).
@Methods:
Evaluates this pdf at x.
This method needs implement by children class, if not there is an UnimplementedMethodException
would be throw when the method is called
@Params:
param | type | description |
---|---|---|
x | number | number |
@Returns: float probability density
Makes a discrete version of this pdf, evaluated at xs.
@Params:
param | type | description |
---|---|---|
xs | string | array |
@Returns: new pmf
Represents a suite of hypotheses and their probabilities.
@Params:
param | type | description |
---|---|---|
values | string | array |
name | string | sequence of values |
@Methods:
Important: This class inherits from Pmf, so you can use all methods of the parent class.
Updates each hypothesis based on the data.
@Params:
param | type | description |
---|---|---|
data | any | any representation of the data |
@Returns: the normalizing constant
Updates a suite of hypotheses based on new data.
Modifies the suite directly; if you want to keep the original, make a copy.
Note: unlike Update, LogUpdate does not normalize.
@Params:
param | type | description |
---|---|---|
any | any | representation of the data |
Updates each hypothesis based on the dataset.
This is more efficient than calling Update repeatedly because
it waits until the end to Normalize.
Modifies the suite directly; if you want to keep the original, make a copy.
@Params:
param | type | description |
---|---|---|
dataset | array | set |
@Returns: the normalizing constant
Updates each hypothesis based on the dataset.
Modifies the suite directly; if you want to keep the original, make a copy.
@Params:
param | type | description |
---|---|---|
dataset | array | set |
Computes the likelihood of the data under the hypothesis.
This method needs implement by children class
if not there is an UnimplementedMethodException
would be throw
@Params:
param | type | description |
---|---|---|
data | any | some representation of the data |
hypo | any | some representation of the hypothesis |
@Returns: likelihood
Computes the log likelihood of the data under the hypothesis.
This method needs implement by children class
if not there is an UnimplementedMethodException
would be throw
@Params:
param | type | description |
---|---|---|
data | any | some representation of the data |
hypo | any | some representation of the hypothesis |
@Returns: likelihood
Transforms from probabilities to odds.
Values with prob=0 are removed.
Transforms from odds to probabilities.
Represents a histogram, which is a map from values to frequencies.
Values can be any hashable type; frequencies are integer counters.
@Params:
param | type | description |
---|---|---|
values | string | array |
name | string | sequence of values |
@Methods:
Important: This class inherits from DictWrapper, so you can use all methods of the parent class.
Gets the frequency associated with the value x.
@Params:
param | type | description |
---|---|---|
x | any | number value |
@Returns: int frequency
Gets frequencies for a sequence of values.
Checks whether the values in this histogram are a subset of
the values in the given histogram.
Subtracts the values in the given histogram from this histogram.
Represents a mapping between sorted sequences; performs linear interp.
@Params:
param | type | description |
---|---|---|
xs | array | sorted list |
ys | array | sorted list |
@Methods:
Looks up x and returns the corresponding value of y.
Looks up y and returns the corresponding value of x.
Represents a joint distribution.
The values are sequences (usually tuples)
@Params:
param | type | description |
---|---|---|
values | string | array |
name | string | sequence of values |
@Methods:
Important: This class inherits from Pmf, so you can use all methods of the parent class.
Gets the marginal distribution of the indicated variable.
@Params:
param | type | description |
---|---|---|
i | number | index of the variable we want |
@Returns: Pmf
Gets the conditional distribution of the indicated variable.
Distribution of vs[i], conditioned on vs[j] = val.
@Params:
param | type | description |
---|---|---|
i | number | index of the variable we want |
j | number | which variable is conditioned on |
val | the value the jth variable has to have |
@Returns: Pmf
Returns the maximum-likelihood credible interval.
If percentage=90, computes a 90% CI containing the values
with the highest likelihoods.
@Params:
param | type | description |
---|---|---|
percentage | number | float between 0 and 100 |
@Returns: list of values from the suite
Represents the PDF of a Gaussian distribution.
@Methods:
Important: This class inherits from Pdf, so you can use all methods of the parent class.
Constructs a Gaussian Pdf with given mu and sigma.
@Params:
param | type | description |
---|---|---|
mu | number | mean |
sigma | number | standard deviation |
Evaluates this Pdf at x.
@Returns: float probability density
TODO: implemente this class.
Represents a PDF estimated by KDE.
Estimates the density function based on a sample.
@Params:
param | type | description |
---|---|---|
sample | array | sequence of data |
@Methods:
Important: This class inherits from Pdf, so you can use all methods of the parent class.
Evaluates this Pdf at x.
@Returns: float probability density
This library provides some Utility Functions following for calculations related to probability and statistics.
These functions can be imported by the same way following:
import { odds, probability, percentile } from 'think-bayes/helpers';
Computes odds for a given probability.
Example: p=0.75 means 75 for and 25 against, or 3:1 odds in favor.
Note: when p=1, the formula for odds divides by zero, which is
normally undefined. But I think it is reasonable to define Odds(1)
to be infinity, so that's what this function does.
@Params:
param | type | description |
---|---|---|
p | number | float 0~1 |
@Returns: float odds
Computes the probability corresponding to given odds.
Example: o=2 means 2:1 odds in favor, or 2/3 probability
@Params:
param | type | description |
---|---|---|
o | number | float odds, strictly positive |
@Returns: float probability
Computes the probability corresponding to given odds.
Example: yes=2, no=1 means 2:1 odds in favor, or 2/3 probability.
@Params:
param | type | description |
---|---|---|
yes | number | int or float odds in favor |
no | number | int or float odds in favor |
Computes a percentile of a given Pmf.
@Params:
param | type | description |
---|---|---|
pmf | pmf | |
percentage | number | float 0-100 |
Computes a credible interval for a given distribution.
If percentage=90, computes the 90% CI.
@Params:
param | type | description |
---|---|---|
pmf | pmf | Pmf object representing a posterior distribution |
percentage | number | float between 0 and 100 |
@Returns: sequence of two floats, low and high
Probability that a value from pmf1 is less than a value from pmf2.
@Params:
param | type | description |
---|---|---|
pmf1 | pmf | Pmf object |
pmf2 | pmf | Pmf object |
@Returns: float probability
Probability that a value from pmf1 is greater than a value from pmf2.
@Params:
param | type | description |
---|---|---|
pmf1 | pmf | Pmf object |
pmf2 | pmf | Pmf object |
@Returns: float probability
Probability that a value from pmf1 equals a value from pmf2.
@Params:
param | type | description |
---|---|---|
pmf1 | pmf | Pmf object |
pmf2 | pmf | Pmf object |
@Returns: float probability
Chooses a random value from each dist and returns the sum.
@Params:
param | type | description |
---|---|---|
dists | array | sequence of Pmf or Cdf objects |
@Returns: numerical sum
Draws a sample of sums from a list of distributions.
@Params:
param | type | description |
---|---|---|
dists | array | sequence of Pmf or Cdf objects |
n | number | sample size |
@Returns: new Pmf of sums
Computes the unnormalized PDF of the normal distribution.
@Params:
param | type | description |
---|---|---|
x | number | value |
mu | number | mean |
sigma | number | standard deviation |
@Returns: float probability density
Makes a PMF discrete approx to a Gaussian distribution.
@Params:
param | type | description |
---|---|---|
mu | number | float mean |
sigma | number | float standard deviation |
numSigmas | number | how many sigmas to extend in each direction |
n | number | number of values in the Pmf |
@Returns: normalized Pmf
Evaluates the binomial pmf.
@Returns: the probabily of k successes in n trials with probability p.
Computes the Poisson PMF.
@Params:
param | type | description |
---|---|---|
k | number | number of events |
lam | number | parameter lambda in events per unit time |
@Returns: float probability
This library use decimal.js to handle the problem what calculation of float point number, in the same way, you can use it in this library:
import { Decimal } from 'think-bayes';
Decimal.add(0.1, 0.2).toNumber() === 0.3; // true
FAQs
An algorithm framework of probability and statistics for browser and Node.js environment.
The npm package think-bayes receives a total of 14 weekly downloads. As such, think-bayes popularity was classified as not popular.
We found that think-bayes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.