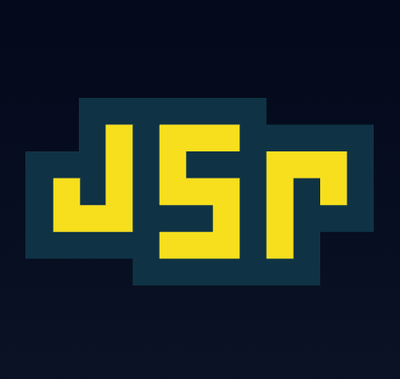
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
A "thin ODM" which is less opinionated and tries to give you query flexibility whilst still providing helpful document handling.
Features:
This package requires Node 4 or above
$ npm install thinodium
This package provides the core infrastructure. To actually access a particular database you will need to additionally install one of the following adapters:
NOTE: Please raise a PR if you want me to add your adapter to the above list.
Let's first create a database connection:
// thinodium instance
const thinodium = require('thinodium');
// create the connection
const db = yield thinodium.connect('rethinkdb', {
db: 'mydb',
});
Thinodium will try to load an NPM module called thinodium-rethinkdb
(which
is our intention in this example). If not
available it will try to load a module called rethinkdb
. Once loaded it will
instantiate a database connection through that module, passing in the second
parameter to connect()
.
If we wished to add a custom adapter but not as an NPM module we could simply
provide its path to connect()
:
// connect using custom adatper
const db = yield thinodium.connect('path/to/custom/adapter', {
db: 'mydb',
});
Models
Once we have our database connection setup we can access models (i.e. tables) within the database:
// get the model
const model = yield db.model('User');
// insert a new user
let user = yield model.insert({
name: 'john'
});
// change the name
user.name = 'mark';
yield user.save();
// load user with id
let user2 = yield model.get(user.id);
console.log(user2.name); /* mark */
Both get()
and insert()
return Thinodium.Document
instances.
These internally call through to the methods prefixed with raw
- methods
which you can also use directly if you do not wish to deal with Document
s.
These are documented in the API docs.
Document customization
Model documents (each representing a row in the table) can be customized. We can add virtual fields and additional methods:
// create the model
const model = yield db.model('User', {
docMethods: {
padName: function(str) {
this.name += str;
}
},
docVirtuals: {
fullName: {
get: function() {
return this.name + ' smith';
}
}
}
});
// insert a new user
let user = yield model.insert({
name: 'john'
});
console.log(user2.fullName); /* mark smith */
user.padName('test');
console.log(user2.fullName); /* marktest smith */
Schema validation
Schema validation is performed by simple-nosql-schema, and is used if a schema is provided in the initial model config:
// create the model
const model = yield db.model('User', {
// tell Thinodium to validate all updates and inserts against the given schema
schema: {
age: {
type: Number,
required: true,
},
}
});
// insert a new user
let user1 = yield model.insert({ name: 'john', }); /* throws Error since age is missing */
let user2 = yield model.insert({
name: 'john',
age: 23,
});
user2.age = 'test';
yield user2.save(); /* throws Error since age must be a number */
Note that schema validation is partial. If the insert or update contains a key that is not mentioned within the schema then that key gets passed through without any checks. This allows for flexibility - you only need to vaildate the parts of a model's schema you're interested in.
Check out the API docs for information on supported methods.
An adapter has to extend the base Database
and Model
classes and
override the necessary internal methods:
"use strict";
const thinodium = require('thinodium');
class Database extends thinodium.Database {
_connect (options) {
return new Promise((resolve, reject) => {
// do what's needed for connection here and save into "connection" var
resolve(connection);
});
}
_disconnect (connection) {
return new Promise((resolve, reject) => {
// disconnect connection
resolve();
});
}
_model (connection, name, config) {
return new Model(connection, name, config);
}
}
class Model extends thinodium.Model {
rawQry() {
// return object for doing raw querying
}
rawGet (id) {
return new Promise((resolve, reject) => {
// fetch doc with given id
resolve(doc);
});
}
rawInsert (attrs) {
return new Promise((resolve, reject) => {
// insert doc
resolve(doc);
});
}
rawUpdate (id, changes, document) {
return new Promise((resolve, reject) => {
// update doc
resolve();
});
}
rawRemove (id) {
return new Promise((resolve, reject) => {
// remove doc with id
resolve();
});
}
}
module.exports = Database;
To run the tests:
$ npm install
$ npm test
Contributions are welcome! Please see CONTRIBUTING.md.
MIT - see LICENSE.md
FAQs
Light-weight, pluggable ODM for Node.js. Supports RethinkDB, etc
We found that thinodium demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.