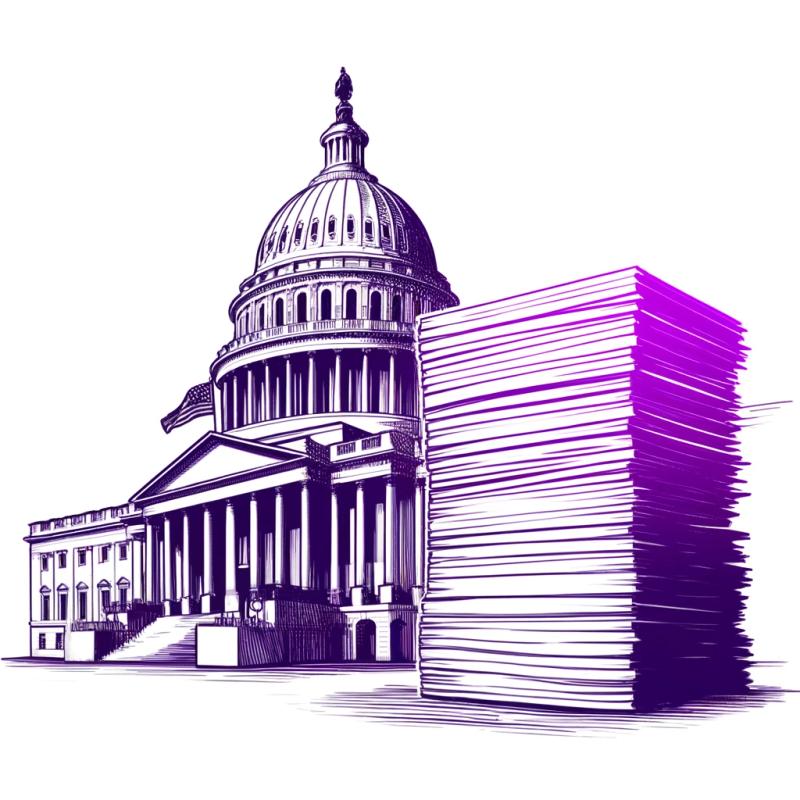
Application Security
SEC Cracks Down on Unreported Data Breaches with New 30-Day Disclosure Requirement for Financial Institutions
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.