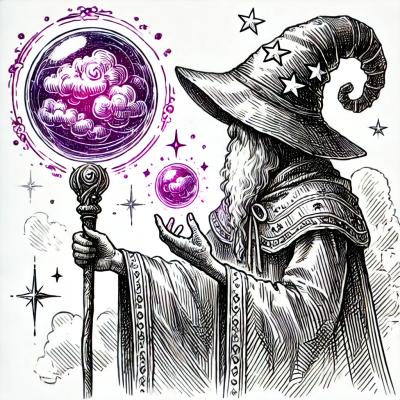
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
This module offers simple bindings for the C time.h APIs.
It also offers an extended regular Date
object with getTimezone()
and setTimezone()
functions, which aren't normally part of JavaScript.
var time = require('time');
// Create a new Date instance
var now = new time.Date();
now.setTimezone("America/Los_Angeles");
// `.getDate()`, `.getDay()`, `.getHours()`, etc.
// will return values according to UTC-8
now.setTimezone("America/New_York");
// `.getDate()`, `.getDay()`, `.getHours()`, etc.
// will return values according to UTC-5
A special Date
constructor that returns a "super" Date instance, that has
magic timezone capabilities!
var date = new time.Date();
Sets the timezone for the Date
instance. Calls to getHours()
, getDays()
,
getMinutes()
, etc. will be relative to the timezone specified. This will throw
an Error if information for the desired timezone could not be found.
date.setTimezone("America/Argentina/San_Juan");
Returns a String containing the currently configured timezone for the date instance.
date.getTimezone();
// "America/Argentina/San_Juan"
Returns the abbreviated timezone name, also taking daylight savings into consideration.
Useful for the presentation layer of a Date instance. This is a NON-STANDARD extension
to the Date object, and must be called after setTimezone()
.
date.getTimezoneAbbr();
// "ART"
Transforms a "regular" Date instance into one of node-time
's "extended" Date instances.
var d = new Date();
// `d.setTimezone()` does not exist...
time.extend(d);
d.setTimezone("UTC");
Binding for time()
. Returns the number of seconds since Jan 1, 1900 UTC.
These two are equivalent:
time.time();
// 1299827226
Math.floor(Date.now() / 1000);
// 1299827226
Binding for tzset()
. Sets up the timezone information that localtime()
will
use based on the specified timezone variable, or the current process.env.TZ
value if none is specified. Returns an Object containing information about the
newly set timezone, or throws an Error if no timezone information could be loaded
for the specified timezone.
time.tzset('US/Pacific');
// { tzname: [ 'PST', 'PDT' ],
// timezone: 28800,
// daylight: 1 }
Binding for localtime()
. Accepts a Number with the number of seconds since the
Epoch (i.e. the result of time()
), and returns a "broken-down" Object
representation of the timestamp, according the the currently configured timezone
(see tzset()
).
time.localtime(Date.now()/1000);
// { seconds: 38,
// minutes: 7,
// hours: 23,
// dayOfMonth: 10,
// month: 2,
// year: 111,
// dayOfWeek: 4,
// dayOfYear: 68,
// isDaylightSavings: false,
// gmtOffset: -28800,
// timezone: 'PST' }
FAQs
"time.h" bindings for Node.js
The npm package time receives a total of 639 weekly downloads. As such, time popularity was classified as not popular.
We found that time demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.