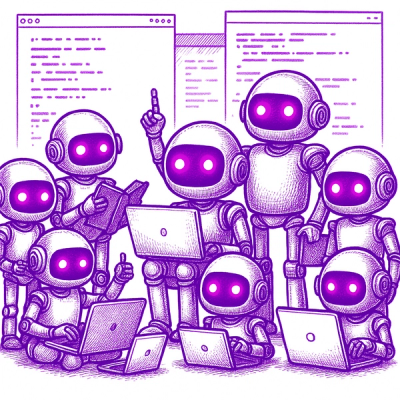
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
timer-node
Advanced tools
A timestamp-based timer that enables recording elapsed time and formatting the result.
A lightweight JavaScript library for measuring elapsed time in Node.js or the browser without using setInterval
or setTimeout
. It also has type definitions for TypeScript.
npm install timer-node
// JS
const { Timer } = require('timer-node');
// TS
import { Timer, TimerOptions, Time } from 'timer-node';
A timestamp-based timer that can be started, paused, resumed, and stopped. It calculates durations based on system time.
new Timer(options?: TimerOptions)
options
: An optional configuration object with:
label?: string
– A label for this timer.startTimestamp?: number
– Start time (if you want to initialize from the past).endTimestamp?: number
– End time (if already stopped).currentStartTimestamp?: number
– Most recent resume time.pauseCount?: number
– Number of times paused.accumulatedMs?: number
– Accumulated milliseconds from previous runs.d
, h
, m
, s
, ms
).ms()
/ time()
, but for paused duration.%label
, %d
, %h
, %m
, %s
, %ms
).Timer.deserialize(serializedTimer: string): Timer
Re-creates a timer from a serialized string generated by .serialize()
.
Timer.benchmark(fn: () => any): Timer
Measures the synchronous execution time of fn
. Returns a stopped Timer
.
Any method returning an object breakdown of time uses the following shape:
interface Time {
d: number; // days
h: number; // hours
m: number; // minutes
s: number; // seconds
ms: number; // milliseconds
}
const { Timer } = require('timer-node');
const timer = new Timer({ label: 'demo' });
timer.start();
// ... some operations ...
timer.pause();
console.log('Paused at', timer.ms(), 'ms');
timer.resume();
// ... more operations ...
timer.stop();
console.log('Total elapsed:', timer.time());
// e.g. { d: 0, h: 0, m: 1, s: 12, ms: 345 }
This library is licensed under the MIT License. See LICENSE for details.
[5.0.9] - 2025-01-26
FAQs
A timestamp-based timer that enables recording elapsed time and formatting the result.
We found that timer-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.