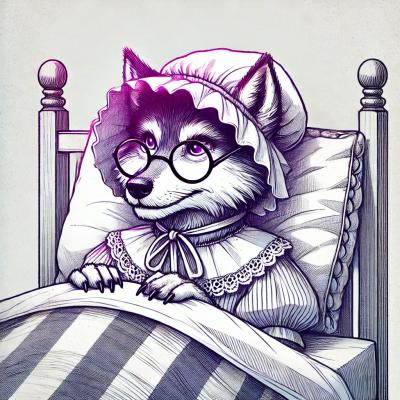
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
minimal fork of nanospy, with more features 🕵🏻♂️
Warning! Does not support mocking ES Modules. You can use it with
vitest
, who does additional transpormations, to mock ESM.
Simplest usage would be:
const fn = (n: string) => n + '!'
const spied = spy(fn)
fn('a')
console.log(method.called) // true
console.log(method.callCount) // 1
console.log(method.calls) // [['a']]
console.log(method.results) // [['ok', 'a!']
console.log(method.returns) // ['a!']
You can reassign mocked function:
const fn = (n: string) => n + '!'
const spied = spy(fn).willCall((n) => n + '.')
fn('a')
console.log(method.returns) // ['a.']
You can reset calls, returns, called and callCount with reset
function and restore mock to it's original implementation with restore
method:
const fn = (n: string) => n + '!'
const spied = spy(fn).willCall((n) => n + '.')
fn('a')
console.log(method.called) // true
console.log(method.callCount) // 1
console.log(method.calls) // [['a']]
console.log(method.returns) // ['a.']
fn.reset()
console.log(method.called) // false
console.log(method.callCount) // 0
console.log(method.calls) // []
console.log(method.returns) // []
fn.restore()
console.log(fn('a')).toBe('a!')
// still spied on!
console.log(method.callCount) // 1
All
spy
methods are available onspyOn
.
You can spy on an object's method or setter/getter with spyOn
function.
let apples = 0
const obj = {
getApples: () => 13,
}
const spy = spyOn(obj, 'getApples', () => apples)
apples = 1
console.log(obj.getApples()) // prints 1
console.log(spy.called) // true
console.log(spy.returns) // [1]
let apples = 0
let fakedApples = 0
const obj = {
get apples() {
return apples
},
set apples(count) {
apples = count
},
}
const spyGetter = spyOn(obj, { getter: 'apples' }, () => fakedApples)
const spySetter = spyOn(obj, { setter: 'apples' }, (count) => {
fakedApples = count
})
obj.apples = 1
console.log(spySetter.called) // true
console.log(spySetter.calls) // [[1]]
console.log(obj.apples) // 1
console.log(fakedApples) // 1
console.log(apples) // 0
console.log(spyGetter.called) // true
console.log(spyGetter.returns) // [1]
You can even make an attribute into a dynamic getter!
let apples = 0
const obj = {
apples: 13,
}
const spy = spyOn(obj, { getter: 'apples' }, () => apples)
apples = 1
console.log(obj.apples) // prints 1
You can restore spied function to it's original value with restore
method:
let apples = 0
const obj = {
getApples: () => 13,
}
const spy = spyOn(obj, 'getApples', () => apples)
console.log(obj.getApples()) // 0
obj.restore()
console.log(obj.getApples()) // 13
FAQs
A minimal fork of nanospy, with more features
The npm package tinyspy receives a total of 3,705,901 weekly downloads. As such, tinyspy popularity was classified as popular.
We found that tinyspy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.