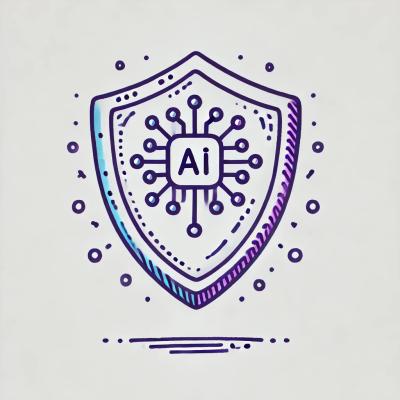
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
to-readable-stream
Advanced tools
The 'to-readable-stream' npm package is a simple utility that allows you to convert a string or buffer into a readable stream. This can be particularly useful when you need to work with stream-based APIs but only have static data to start with.
Convert string to stream
This feature allows you to convert a simple string into a readable stream, which can then be used with any API that accepts streams. The example demonstrates creating a stream from a string and reading from it.
const toReadableStream = require('to-readable-stream');
const stream = toReadableStream('Hello, world!');
stream.on('data', (chunk) => {
console.log(chunk.toString()); // Outputs: Hello, world!
});
Convert buffer to stream
This feature enables the conversion of a buffer into a readable stream. Similar to the string example, this allows buffers to be used wherever streams are required. The code sample shows how to convert a buffer to a stream and read its contents.
const toReadableStream = require('to-readable-stream');
const buffer = Buffer.from('Hello, buffer!');
const stream = toReadableStream(buffer);
stream.on('data', (chunk) => {
console.log(chunk.toString()); // Outputs: Hello, buffer!
});
The 'into-stream' package offers similar functionality to 'to-readable-stream' by converting strings, buffers, or arrays into readable streams. It provides a bit more flexibility compared to 'to-readable-stream' by supporting arrays of items, which can be particularly useful when you need to stream multiple items in sequence.
Streamifier is another package that can convert a buffer or a string to a stream. It is quite similar to 'to-readable-stream' but also supports creating streams from large objects, which might be useful for applications dealing with large datasets or files.
Convert a value to a
ReadableStream
Not to be confused with Node.js stream.Readable
, in which case, stream.Readable#from()
should be used instead.
npm install to-readable-stream
import toReadableStream from 'to-readable-stream';
toReadableStream('🦄🌈');
//=> ReadableStream<'🦄🌈'>
Returns a ReadableStream
.
The value to convert to a stream.
FAQs
Convert a value to a ReadableStream
The npm package to-readable-stream receives a total of 3,082,729 weekly downloads. As such, to-readable-stream popularity was classified as popular.
We found that to-readable-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.