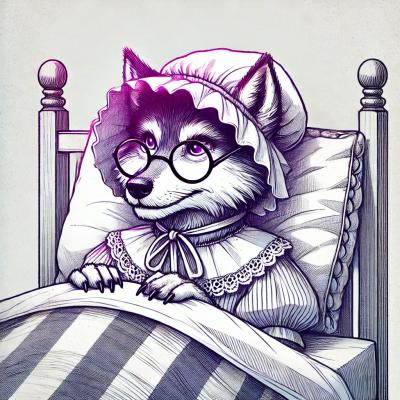
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
to-regex-range
Advanced tools
Pass two numbers, get a regex-compatible source string for matching ranges. Validated against more than 2.87 million test assertions.
The to-regex-range npm package is designed to generate regular expressions for matching numeric ranges. It is useful for creating regex patterns that can match against specific ranges of numbers, allowing for precise control over numeric input validation, among other applications.
Generating regex for simple numeric ranges
This feature allows you to generate a regex pattern for a simple numeric range, such as from 5 to 10. The resulting regex will match any number within this range.
const toRegexRange = require('to-regex-range');
const regex = toRegexRange('5', '10');
console.log(regex); // => '5|6|7|8|9|10'
Creating regex with zero-padding
This feature enables the generation of regex patterns that account for zero-padded numbers, useful for matching numbers within a range where the number of digits is consistent.
const toRegexRange = require('to-regex-range');
const regex = toRegexRange('001', '100', { capture: true, pad: true });
console.log(regex); // Example output: '(0[0-9]{2}|1[0-9]{2})'
Generating regex for complex ranges with options
This feature allows for the creation of regex patterns for more complex numeric ranges with additional options, such as disabling the relaxation of leading zeros, providing more precise control over the matching behavior.
const toRegexRange = require('to-regex-range');
const regex = toRegexRange('10', '299', { relaxZeros: false });
console.log(regex); // Example output: '1[0-9]|2[0-9]{2}'
Pass two numbers, get a regex-compatible source string for matching ranges. Validated against more than 2.87 million test assertions.
Install with npm:
$ npm install --save to-regex-range
Install with yarn:
$ yarn add to-regex-range
This libary generates the source
string to be passed to new RegExp()
for matching a range of numbers.
Example
var toRegexRange = require('to-regex-range');
var regex = new RegExp(toRegexRange('15', '95'));
A string is returned so that you can do whatever you need with it before passing it to new RegExp()
(like adding ^
or $
boundaries, defining flags, or combining it another string).
Creating regular expressions for matching numbers gets deceptively complicated pretty fast.
For example, let's say you need a validation regex for matching part of a user-id, postal code, social security number, tax id, etc:
1
=> /1/
(easy enough)1
through 5
=> /[1-5]/
(not bad...)1
or 5
=> /(1|5)/
(still easy...)1
through 50
=> /([1-9]|[1-4][0-9]|50)/
(uh-oh...)1
through 55
=> /([1-9]|[1-4][0-9]|5[0-5])/
(no prob, I can do this...)1
through 555
=> /([1-9]|[1-9][0-9]|[1-4][0-9]{2}|5[0-4][0-9]|55[0-5])/
(maybe not...)0001
through 5555
=> /(0{3}[1-9]|0{2}[1-9][0-9]|0[1-9][0-9]{2}|[1-4][0-9]{3}|5[0-4][0-9]{2}|55[0-4][0-9]|555[0-5])/
(okay, I get the point!)The numbers are contrived, but they're also really basic. In the real world you might need to generate a regex on-the-fly for validation.
Learn more
If you're interested in learning more about character classes and other regex features, I personally have always found regular-expressions.info to be pretty useful.
As of April 22, 2017, this library runs 2,783,483 test assertions against generated regex-ranges to provide brute-force verification that results are indeed correct.
Tests run in ~870ms on my MacBook Pro, 2.5 GHz Intel Core i7.
Generated regular expressions are highly optimized:
?
conditionals when number(s) or range(s) can be positive or negativeAdd this library to your javascript application with the following line of code
var toRegexRange = require('to-regex-range');
The main export is a function that takes two integers: the min
value and max
value (formatted as strings or numbers).
var source = toRegexRange('15', '95');
//=> 1[5-9]|[2-8][0-9]|9[0-5]
var re = new RegExp('^' + source + '$');
console.log(re.test('14')); //=> false
console.log(re.test('50')); //=> true
console.log(re.test('94')); //=> true
console.log(re.test('96')); //=> false
Range | Result | Compile time |
---|---|---|
toRegexRange('5, 5') | 5 | 26μs |
toRegexRange('5, 6') | 5|6 | 47μs |
toRegexRange('29, 51') | 29|[3-4][0-9]|5[0-1] | 452μs |
toRegexRange('31, 877') | 3[1-9]|[4-9][0-9]|[1-7][0-9]{2}|8[0-6][0-9]|87[0-7] | 756μs |
toRegexRange('111, 555') | 11[1-9]|1[2-9][0-9]|[2-4][0-9]{2}|5[0-4][0-9]|55[0-5] | 61μs |
toRegexRange('-10, 10') | -[1-9]|-?10|[0-9] | 69μs |
toRegexRange('-100, -10') | -1[0-9]|-[2-9][0-9]|-100 | 42μs |
toRegexRange('-100, 100') | -[1-9]|-?[1-9][0-9]|-?100|[0-9] | 42μs |
toRegexRange('001, 100') | 0{2}[1-9]|0[1-9][0-9]|100 | 135μs |
toRegexRange('0010, 1000') | 0{2}1[0-9]|0{2}[2-9][0-9]|0[1-9][0-9]{2}|1000 | 53μs |
toRegexRange('1, 2') | 1|2 | 18μs |
toRegexRange('1, 5') | [1-5] | 23μs |
toRegexRange('1, 10') | [1-9]|10 | 22μs |
toRegexRange('1, 100') | [1-9]|[1-9][0-9]|100 | 23μs |
toRegexRange('1, 1000') | [1-9]|[1-9][0-9]{1,2}|1000 | 60μs |
toRegexRange('1, 10000') | [1-9]|[1-9][0-9]{1,3}|10000 | 117μs |
toRegexRange('1, 100000') | [1-9]|[1-9][0-9]{1,4}|100000 | 42μs |
toRegexRange('1, 1000000') | [1-9]|[1-9][0-9]{1,5}|1000000 | 46μs |
toRegexRange('1, 10000000') | [1-9]|[1-9][0-9]{1,6}|10000000 | 60μs |
Order of arguments
When the min
is larger than the max
, values will be flipped to create a valid range:
toRegexRange('51', '29');
Is effectively flipped to:
toRegexRange('29', '51');
//=> 29|[3-4][0-9]|5[0-1]
Steps / increments
This library does not support steps (increments). A pr to add support would be welcome.
New features
Adds support for zero-padding!
Optimizations
Repeating ranges are now grouped using quantifiers. rocessing time is roughly the same, but the generated regex is much smaller, which should result in faster matching.
Inspired by the python library range-regex.
step
to… more | homepagePull requests and stars are always welcome. For bugs and feature requests, please create an issue.
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
Jon Schlinkert
Copyright © 2017, Jon Schlinkert. Released under the MIT License.
This file was generated by verb-generate-readme, v0.5.0, on April 22, 2017.
FAQs
Pass two numbers, get a regex-compatible source string for matching ranges. Validated against more than 2.78 million test assertions.
The npm package to-regex-range receives a total of 59,041,401 weekly downloads. As such, to-regex-range popularity was classified as popular.
We found that to-regex-range demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.