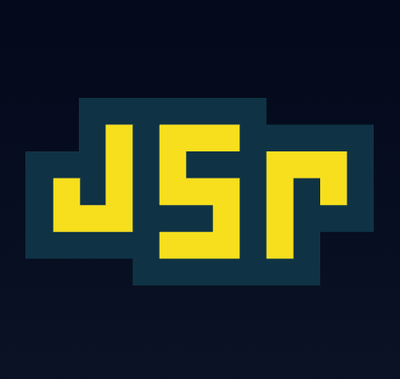
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The 'to-vfile' npm package is a utility for working with virtual files in Node.js. It allows you to create, read, write, and manipulate file objects in memory, which can be particularly useful for processing files in a pipeline without needing to interact with the filesystem directly.
Creating a virtual file
This feature allows you to create a virtual file object with specified path and contents. The file object can then be manipulated in memory.
const vfile = require('to-vfile');
const file = vfile({ path: 'example.txt', contents: 'Hello, world!' });
console.log(file);
Reading a file from the filesystem
This feature allows you to read a file from the filesystem into a virtual file object. The contents of the file are loaded into memory, and you can manipulate the file object as needed.
const vfile = require('to-vfile');
vfile.read('example.txt', 'utf8', (err, file) => {
if (err) throw err;
console.log(file);
});
Writing a virtual file to the filesystem
This feature allows you to write a virtual file object to the filesystem. The contents of the virtual file are saved to the specified path.
const vfile = require('to-vfile');
const file = vfile({ path: 'example.txt', contents: 'Hello, world!' });
vfile.write(file, err => {
if (err) throw err;
console.log('File written!');
});
Vinyl is a similar package that provides a virtual file format. It is commonly used in the Gulp ecosystem for handling file transformations in memory. Compared to 'to-vfile', Vinyl is more focused on stream-based workflows and integrates tightly with Gulp.
Memfs is a package that provides an in-memory filesystem. It allows you to perform filesystem operations in memory, which can be useful for testing and mocking. While 'to-vfile' focuses on individual file objects, Memfs provides a broader filesystem abstraction.
Create a vfile from a file-path.
npm:
npm install to-vfile
to-vfile is also available for bower, component, and duo, and as an AMD, CommonJS, and globals module, uncompressed and compressed.
Note: browser-builds do not include
read
andreadSync
.
var toVFile = require('to-vfile');
var file = toVFile('./readme.md');
/*
* { contents: '',
* filename: 'readme',
* directory: '.',
* extension: 'md',
* messages: [],
* __proto__: [VFile] }
*/
toVFile.read('.git/HEAD', function (err, file) {
if (err) throw err;
console.log(file);
/*
* { contents: 'ref: refs/heads/master\n',
* filename: 'HEAD',
* directory: '.git',
* extension: '',
* messages: [],
* __proto__: [VFile] }
*/
});
var file = toVFile.readSync('.gitignore')
/*
* { contents: '.DS_Store\n*.log\nbower_components/\nbuild/\ncomponents/\nnode_modules/\ncoverage/\nbuild.js\n',
* filename: '.gitignore',
* directory: '.',
* extension: '',
* messages: [],
* __proto__: [VFile] }
*/
Create a virtual file from filePath
.
Signatures
file = toVFile(filePath)
.Parameters
filePath
(string
) — Path to a (possibly non-existent) file;Returns
vfile
— Instance.
Create a virtual file from filePath
and fill it with the actual contents
at filePath
.
Signatures
toVFile.read(filePath, callback)
.Parameters
filePath
(string
) — Path to a (possibly non-existent) file;callback
— See function done(err, vfile)
.Returns
vfile
— Instance.
Callback.
Signatures
function done(Error, null)
;function done(null, VFile)
.Parameters
err
(Error
) — Error from reading filePath
;vfile
(VFile
) — Virtual file.Create a virtual file from filePath
and fill it with the actual contents at
filePath
(synchroneously).
Signatures
toVFile.read(filePath, callback)
.Parameters
filePath
(string
) — Path to a (possibly non-existent) file;Returns
vfile
— Instance.
Throw
Error
— When reading filePath
fails.
FAQs
vfile utility to read and write to the file system
The npm package to-vfile receives a total of 252,136 weekly downloads. As such, to-vfile popularity was classified as popular.
We found that to-vfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.