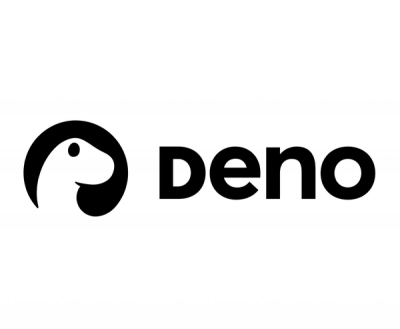
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
tools-for-instagram
Advanced tools
Automation scripts for Instagram
1. npm install tools-for-instagram
2. create a .env file on the main directory with this fields:
IG_USERNAME=myUsername
IG_PASSWORD=myPassword
# Uncomment next line if you want to be in offline chat status
# ONLINE_MODE=false
# Uncomment next line to use a proxy
# IG_PROXY=http://proxyuser:proxypassword@xxx.xxx.xxx.xxx:xxxxx
# Uncomment next line to use email verifications | sms by default
# IG_VERIFICATION=email
3. Copy the example.js on the root folder of your project from the repo
4. Execute'node example.js' to test the scripts.
1. Write your automation bots or copy the existent ones inside Tools-for-Instagram module
(Recommended to create a 'bots' folder in root of the project)
2. Tests the bot using 'node bots/yourBot.js'
1. Download the repo and install dependencies using this terminal commands:
git clone https://github.com/linkfy/Tools-for-Instagram.git
cd Tools-for-Instagram
npm install
2. Rename .env_example to .env and edit the configuration.
3. Execute 'node example.js' to test the scripts.
1. Write your automation bots inside bots folder or use the existent ones
2. Tests the bot using 'node bots/bot.js'
Download this - Replace the node_modules folder (1.8.0) on Windows
Download this - Replace the node_modules folder (1.8.2) on Linux
https://github.com/linkfy/Tools-for-Instagram/wiki
https://t.me/toolsforinstagram
You can follow the streams of the project on the Twitch channel
https://www.twitch.tv/mimi_twitchbot
Follow the development status to see what's the next upcoming idea
https://trello.com/b/ZlwRr6l0/tools-for-instagram
require("tools-for-instagram");
(async () => {
let ig = await login();
// .. Implement your code here
let info = await getUserInfo(ig, "Instagram");
console.log("User information, username: " + info.username);
// ..
})();
require('./src/tools-for-instagram');
(async () => {
let ig = await login();
// .. Implement your code here
let info = await getUserInfo(ig, "Instagram");
console.log("User information, username: " + info.username);
// ..
})();
Load a config file from the accounts folder
By default it will use the proxy from .env file if the proxy is not set on login().
let acc = loadConfig('exampleAccount');
let myAccount2 = await login(acc);
//same as await login("username", "password");
To avoid the default proxy from the .env file use false as inputProxy parameter on login file.
module.exports = {
inputLogin: 'accountName',
inputPassword: 'password',
inputProxy: false,
}
Currently Antiban is for likes/follow/unfollow. WARNING: Antiban IS NOT MAGIC, if you use simple code/examples Like_Easy or Like_With_Scanner (Super simple codes) it is possible to be banned anyway. A good Example is Like_Avoid_Bans, use it with caution.
let ig = await login();
await setAntiBanMode(ig);
Also it is possible to disable it:
let ig = await login();
await setAntiBanMode(ig, false);
Get the user information of the desired username
let info = await getUserInfo(ig, 'Instagram');
Get the last likers (max of 1000) of a post
let posts = await getUserRecentPosts(ig, username);
let likers = await getRecentPostLikers(ig, posts[0]);
Get the last likers (max of 1000) of the last post of the desired username
let likers = await getRecentPostLikersByUsername(ig,'instagram');
//Show the last liker of the array
console.log(likers[likers.length-1]);
It will save the followers inside the outputfolder with the format "acountName_followers.json"
await getFollowers(ig, 'Instagram');
Get your last 100 followers, and save it to database, when some follower is new you will a is_new_follower attirbute
let followers = await getMyLastFollowers(ig);
console.log(followers[0].is_new_follower);
It will save the following inside the outputfolder with the format "acountName_following.json"
await getFollowing(ig, 'Instagram');
It will return the followers inside the outputfolder with the format "acountName_followers.json"
let followers = await readFollowers(ig, 'Instagram');
It will return the following inside the outputfolder with the format "acountName_following.json"
let followers = await readFollowing(ig, 'Instagram');
like the desired instagram Url, the like will be saved inside database.
await likeUrl(ig, 'https://www.instagram.com/p/B1gzzVpA462/');
If 'force' is set to true, when the item was already liked before it will force to continue the operation.
await likeUrl(ig, 'https://www.instagram.com/p/B1gzzVpA462/', true);
View all the current stories of the given username
await viewStoriesFromUser(ig, 'instagram');
Get Stories that currently have polls
let user = await getUserInfo(ig, "its.crystinx");
let stories = await getStoriesFromId(ig, user.id);
let polls = await getPollsFromStories(ig, stories);
Vote a poll, the value can be 0 or 1 (left or right)
let stories = await getStoriesFromId(ig, 18839378120);
let polls = await getPollsFromStories(ig, stories);
for(p in polls) {
await voteStoryPoll(ig, polls[p]);//By default the value is always 1 if we don't set it
}
Returns True or False if the current time is inside the range
await isTimeInRange("10:00", "23:00");
It is also possible to calculate night ranges between the current day and tomorrow.
await isTimeInRange("23:00", "3:00");
Automate like actions on given array of recent hashtags feed
let likesPerInterval = 15;
let waitMinutesBetweenLikes = 3;
let intervals = [
["7:00", "8:00"],
["10:00", "11:00"],
["22:00", "23:00"],
];
let hashtagArray = [
"cats",
"dogs",
"music"
];
let likeInterval = likeRecentHashtagsByIntervals(
ig,
hashtagArray,
intervals,
likesPerInterval,
waitMinutesBetweenLikes);
It is also possible to stop the interval clearing it
clearInterval(likeInterval);
Upload a picture from computer to Instagram account
const path = require('path');
let myPicturePath = path.join(__dirname, '/images');
await uploadPicture(ig, "My picture", myPicturePath);
This example is not tested yet, if anyone can verify, please send a message to Issues section: Set tagged accounts to it
const path = require('path');
let myPicture = path.join(__dirname, '/images/image.jpg');
let album = [];
album.push(myPicture);
await uploadAlbum(ig, "My picture", "caption", album, namesToTag = ['linkfytester', 'instagram']);
let pictureUrl = "https://i.ytimg.com/vi/w6-1O0WCdGM/maxresdefault.jpg";
let caption = "This is a test image that I'll delete in a few seconds";
let image = await uploadPictureByUrl(ig, caption, pictureUrl);
Post a comment on the desired post giving the media Id
let posts = await recentHashtagList(ig, "dogs");
await commentMediaId(ig, posts[0].pk, "Amazing!");
let posts = await recentHashtagList(ig, "dogs");
await commentPost(ig, posts[0], "Lovely!");
By default you will get 20 comments:
let posts = await topHashtagList(ig, "dogs");
//Each post have a pk (private key) that is used as Id.
let comments = await getPostCommentsById(ig, posts[0].pk);
comments.forEach(comment => {
console.log(comment.text);
});
You can specify a max for the comments:
let posts = await topHashtagList(ig, "dogs");
//Get 100 comments
let comments = await getPostCommentsById(ig, posts[0].pk, 100);
comments.forEach(comment => {
console.log(comment.text);
});
Send message by user id
let user = await getUserInfo(ig, "Instagram");
let sendDm = await replyDirectMessage(ig,{ userId : user.pk }, "yay!");
Send message by Inbox thread Id
let inbox = await getInbox(ig);
let sendDm = await replyDirectMessage(ig,{ threadId : inbox[0].threadId }, "yay!");
Send Story as a Message to userId or ThreadId
let ig = await login();
let instagram = await getUserInfo(ig, 'instagram');
let stories = await getStoriesFromId(ig, instagram.id);
let linkfy = await getUserInfo(ig, 'linkfytester');
//Share normal message
await replyDirectMessage(ig, {userId: linkfy.id}, 'I will send you a story');
//Share story test
await replyDirectMessage(ig, {userId: linkfy.id, story:stories[0]});
Get the basic information of some user, also the last posts
(async () => {
let info = await spider.getUserInfo("linkfytester");
console.log(info);
})();
Get true or false if the user has stories
(async () => {
let info = await spider.userHasStories("linkfytester");
console.log(info);
})();
Get user likers of some post, it is useful when trying to scrap more than 10k users
(async () => {
let users = await spider.getUserLikers("linkfytester");
console.log(info);
})();
(async () => {
let users = await spider.getUserLikers("linkfytester", maxUsers=50);
console.log(info);
})();
We will be able to use "workers". Multiple executions of bots that work as "threads" and report the execution to a Boss or Controller. Example Uses:
node bots/bosses/exampleBoss
There is also possible to execute advanced workers with the function executeAdvancedWorker, instead of sending an 'accountLogin' parameter, use the 'variables' parameter to send all the related parameters and information to the bot.
For example the advanced boss scriptLoaderBoss.js sends an script name to the scriptLoader.js worker.
let worker1 = executeAdvancedWorker({
workerName: 'scriptLoader',
variables: {scriptName: 'Message_New_Followers'},
timeout: Infinity // Set to Infinity to avoid Timeout seconds on subroutines
});
The advanced worker read the variables like that:
module.exports = function (variables, callback) {
(async () => {
console.log(variables.scriptName +'.js')
///...
Recursive intervals (setInterval) are only executed once when normal scripts are called from a worker, so consider to set the intervals inside the worker code and not inside the script code. In this example (scriptLoader.js) you can see how Message_New_Followers is only executed once for that reason.
The CLI is currently under construction so it will change constantly
Inside the root folder on git / the node_modules folder on npm
cd cli
npm link
cd ..
Well done! Now you can use tfi on your current session
Use tfi from the root working folder to see expected results.
Get the current available commands:
tfi help
Create a new bot:
tfi new bot
Create a new bot called myBot:
tfi new bot myBot
Execute an existing bot [Proxy is optional]:
tfi start -b bots/botname.js -u username -p password -y proxy
When the cookie exists you can use it without password
tfi start -b bots/botname.js -u username
FAQs
Tools for instagram
The npm package tools-for-instagram receives a total of 37 weekly downloads. As such, tools-for-instagram popularity was classified as not popular.
We found that tools-for-instagram demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.