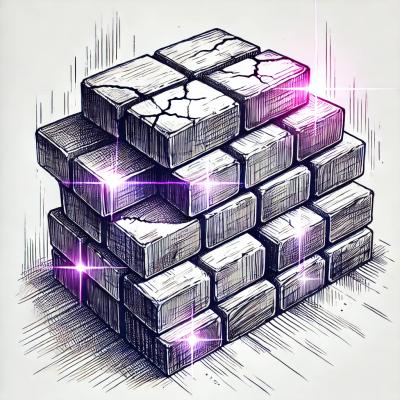
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
transformalizer
Advanced tools
a bare bones node module for building JSON API v1.0 compliant payloads
a bare bones node module for transforming raw data into JSON API v1.0 compliant payloads.
this module:
$ npm install --save transformalizer
Create a new transformalizer and register schemas
import createTransformalizer from 'transformalizer';
// create a new transformalizer
const transformalizer = createTransformalizer();
// register a schema
transformalizer.register({
name: 'article',
schema: { /* see below for schema details and examples */ },
});
// transform raw data into a valid JSON API v1.0 document
const document = transformalizer.transform({ name: 'article', source });
console.log(JSON.stringify(document));
See examples in the examples folder of this repository.
Create a new transformalizer object
Name | Type | Description |
---|---|---|
[options={}] | Object | global options shared between all schemas |
const createTransformalizer = require('transformalizer')
const transformalizer = createTransformalizer()
Register a new document schema.
Name | Type | Description |
---|---|---|
params | Object | |
params.name | String | schema name |
params.schema | Object | mappings for type, see Schema for more details |
transformalizer.register({
name: 'blog-post',
schema: {
// ..
}
})
Build a json api document using the schema with specified name and with the given source data.
Name | Type | Description |
---|---|---|
params | Object | |
params.name | String | the name of the schema to use |
params.source | Object | Object[] |
[params.options={}] | Object | additional data to be passed to transform functions, this will be merged with the global options |
const blogPost = { title: 'Hello, World!', body: 'To be continued...', createdAt: new Date() }
const document = transformalizer.transform({ name: 'blog-post', source: blogPost })
Reconstruct data objects from a json api document.
Name | Type | Description |
---|---|---|
params | Object | |
params.document | Object | json api document |
params.options={} | Object | additional data to be passed to untransform functions, this will be merged with the global options |
const payload = { data: { id: '1', type: 'blog-post', attributes: { title: 'Hello, World!', body: 'To be continued...', createdAt: '2017-09-19T13:10:00' } } }
const data = transformalizer.untransform({ document: payload })
Global options are passed in when creating a new transformalizer object. Options can also be passed in when transforming source objects to the json-api format or untransforming a json-api document back to data objects.
Name | Type | Description |
---|---|---|
untransformIncluded | Boolean | A value indicating whether included resources are untransformed back to data objects |
nestIncluded | Boolean | A value indicating whether the full object hierarchy is recreated using the included data objects. Caveat: This can lead to circular references. |
removeCircularDependencies | Boolean | A value indicating whether circular dependencies in the full object hierarchy should be removed. Caveat: This may lead to an unexpected object hierarchy depending on how the data relationships are structured. |
A schema object defines a set of functions used to transform your raw data into a valid JSON API document. It has the following basic structure (that closely resembles a json api document), which is described in more detail below
{
links({ source, options, data, included }) {
return { /* top level links */ };
},
meta({ source, options, data, included }) {
return { /* top level meta */ };
},
data: {
dataSchema({ source, options, data }) {
return 'other-schema-name';
},
untransformDataSchema({ type, resource, document, options }) {
return 'my-type';
},
type({ source, options, data, state }) {
return 'my-type';
},
id({ source, options, data, type, state }) {
return data.id.toString();
},
untransformId({ id, type, options }) {
return parseInt(id, 10);
},
attributes({ source, options, data, type, id, state }) {
return { /* resource attributes */ }
},
untransformAttributes({ id, type, attributes, resource, options }) {
return { /* resource attributes */ }
},
relationships: {
// ..
[key]({ source, options, data, type, id, attributes, state }) {
return {
data: {
name: 'related-schema',
data: { /* relationship data to be passed to other schema */ },
included: true,
},
links: { /* relationship links if available */ },
meta: { /* relationship meta if available */ }
}
},
// ..
},
links({ source, options, data, type, id, attributes, relationships, state }) {
return { /* resource links if available */ }
},
meta({ source, options, data, type, id, attributes, relationships, state }) {
return { /* resource meta if available */ }
}
}
}
A function that should return the top level links object.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function, merged with the global options object |
params.data | Object | the json api document data after transform |
params.included | Object[] | the json api document included data after transform |
A function that should return the top level meta object.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function, merged with the global options object |
params.data | Object | the json api document data after transform |
params.included | Object[] | the json api document included data after transform |
A function that should return the type of the resource being processed. If this is not provided, the name of the schema will be used as the resource type.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the id of the data object being processed. If this is not provided, it is assumed that the "id" of the resource is simply the "id" property of the source object.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.type | String | the resource type determined in the data.type step |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the id of the resource being processed. If this is not provided, it is assumed that the "id" of the data object is simply the "id" property of the resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.id | String | the resource id |
params.type | String | the resource type |
params.options | Object | any options passed to the #untransform function |
A function that should return the attributes portion of the resource being processed. If a null or undefined value is returned, no attributes will be included on the resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.type | String | the resource type determined in the data.type step |
params.id | String | the id of the current resource, determined in the data.id step |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the attributes portion of the data object being processed. If this is not provided, it is assumed that the attributes of the data object are simply the "attributes" property of the resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.id | Object | the id of the current data object, determined in the data.untransformId step |
params.type | String | the resource type |
params.attributes | Object | the json-api resource object attributes |
params.resource | Object | the full json-api resource object |
params.options | Object | any options passed to the #untransform function |
A map of relationship keys to functions that should return a valid relationship object with one caveat outlined below. If a null or undefined value is returned, that relationship will be excluded from the relationships object.
Caveat: The data property of the relationship object should either be a single object or an array of objects in the form shown below
{
name: 'schemaName', // the name of the related schema to use to transform the related item
data: { /* the "data" param to be passed to the related schema's functions */ },
included: true, // optional, required if the related item should be included
meta: { /* a meta object to be included on the resource identifier object */ }
}
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.type | String | the resource type determined in the data.type step |
params.id | String | the id of the current resource, determined in the data.id step |
params.attributes | Object | the attributes object of the current resource, determined in the data.attributes step |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the links object for the current resource. If a null or undefined value is returned, no links will be included on the resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.type | String | the resource type determined in the data.type step |
params.id | String | the id of the current resource, determined in the data.id step |
params.attributes | Object | the attributes object of the current resource, determined in the data.attributes step |
params.relationships | Object | the relationships object of the current resource, determined in the data.relationships step |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the meta object for the current resource. If a null or undefined value is returned, no attributes will be included on the resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
params.type | String | the resource type determined in the data.type step |
params.id | String | the id of the current resource, determined in the data.id step |
params.attributes | Object | the attributes object of the current resource, determined in the data.attributes step |
params.relationships | Object | the relationships object of the current resource, determined in the data.relationships step |
params.links | Object | the links object of the current resource, determined in the data.links step |
params.state | Object | the recommended namespace for passing information between data level methods, useful for storing calculated data that is needed in multiple places |
A function that should return the name of a schema to use to transform the current source object. Useful for building documents who's primary data is a collection of multiple types.
Name | Type | Description |
---|---|---|
params | Object | |
params.source | Object[],Object | the source data passed to the #transform function |
params.options | Object | any options passed to the #transform function |
params.data | Object | the current item being processed when source is an array, or the source itself if not an array |
A function that should return the name of a schema to use to untransform the current resource.
Name | Type | Description |
---|---|---|
params | Object | |
params.type | String | the resource type |
params.resource | Object | the full json-api resource object |
params.document | Object | the full json-api document |
params.options | Object | any options passed to the #untransform function |
Run the test suite
$ npm test
Run coverage
$ npm run coverage
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)Copyright (c) 2017 Gaia. Licensed under the MIT license.
FAQs
a bare bones node module for building JSON API v1.0 compliant payloads
The npm package transformalizer receives a total of 7 weekly downloads. As such, transformalizer popularity was classified as not popular.
We found that transformalizer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.