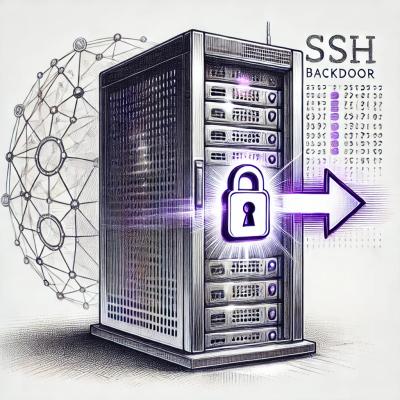
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
transmutant
Advanced tools
A powerful, type-safe TypeScript library for transmuting objects through flexible schema definitions.
npm install transmutant
import { Schema, transmute } from 'transmutant'
// Source type
interface User {
firstName: string;
lastName: string;
email: string;
}
// Target type
interface UserDTO {
fullName: string;
contactEmail: string;
}
// Define transmutation schema
const schema: Schema<User, UserDTO>[] = [
{
to: 'fullName',
from: ({ source }) => `${source.firstName} ${source.lastName}`
},
{
to: 'contactEmail',
from: 'email'
}
]
// Transmute the object
const user: User = {
firstName: 'John',
lastName: 'Doe',
email: 'john@example.com'
}
const userDTO = transmute(schema, user)
// Result: { fullName: 'John Doe', contactEmail: 'john@example.com' }
A schema is an array of transmutation rules that define how properties should be mapped from the source to the target type. Each rule specifies:
to
)from
)type Schema<Source, Target, Extra> = {
to: keyof Target,
from: keyof Source | Transmuter<Source, Target, Extra>
}
Map a property directly from source to target when types are compatible:
interface Source {
email: string;
}
interface Target {
contactEmail: string;
}
const schema: Schema<Source, Target>[] = [
{ to: 'contactEmail', from: 'email' }
]
Transmute properties using custom logic with full type safety:
interface Source {
age: number;
}
interface Target {
isAdult: boolean;
}
const schema: Schema<Source, Target>[] = [
{
to: 'isAdult',
from: ({ source }) => source.age >= 18
}
];
Include additional context in transmutations through the extra
parameter:
interface Source {
city: string;
country: string;
}
interface Target {
location: string;
}
interface ExtraData {
separator: string;
}
const schema: Schema<Source, Target, ExtraData>[] = [
{
to: 'location',
from: ({ source, extra }) =>
`${source.city}${extra.separator}${source.country}`
}
];
const result = transmute(schema,
{ city: 'New York', country: 'USA' },
{ separator: ', ' }
);
// Result: { location: 'New York, USA' }
// Arguments passed to a mutation function
type TransmuterArgs<Source, Extra> = { source: Source, extra?: Extra }
// Function that performs a custom transmutation
type Transmuter<Source, Target, Extra> = (args: TransmuterArgs<Source, Extra>) => Target[keyof Target]
// Defines how a property should be transmuted
type Schema<Source, Target, Extra> = {
to: keyof Target,
from: keyof Source | Transmuter<Source, Target, Extra>
}
Main function for performing object transmutations.
function transmute<Source, Target, Extra>(
schema: Schema<Source, Target, Extra>[],
source: Source,
extra?: Extra
): Target;
Parameter | Type | Description |
---|---|---|
schema | Schema<Source, Target, Extra>[] | Array of transmutation rules |
source | Source | Source object to transmute |
extra | Extra (optional) | Additional data for transmutations |
Returns an object of type Target
with all specified transmutations applied.
The library provides strong type checking for both direct mappings and custom transmutations:
interface Source {
firstName: string;
lastName: string;
age: number;
}
interface Target {
fullName: string;
isAdult: boolean;
}
// Correct usage - types match
const validSchema: Schema<Source, Target>[] = [
{
to: 'fullName',
from: ({ source }) => `${source.firstName} ${source.lastName}` // Returns string
},
{
to: 'isAdult',
from: ({ source }) => source.age >= 18 // Returns boolean
}
];
// Type error - incorrect return type
const invalidSchema: Schema<Source, Target>[] = [
{
to: 'isAdult',
from: ({ source }) => source.age // Error: number not assignable to boolean
}
];
Contributions are welcome! Feel free to submit a Pull Request.
MIT © Antoni Oriol
FAQs
Powerful type transmutations for TypeScript 🧬
The npm package transmutant receives a total of 2 weekly downloads. As such, transmutant popularity was classified as not popular.
We found that transmutant demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.