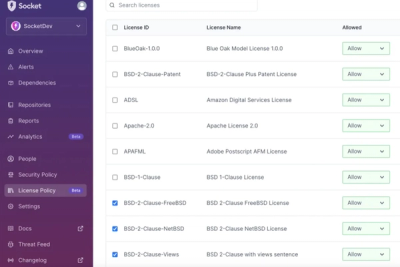
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
tree-changes
Advanced tools
The tree-changes npm package is a utility for detecting changes between two states of a JavaScript object. It helps in identifying added, updated, and removed properties, making it useful for state management and change detection in applications.
Detect Added Properties
This feature allows you to detect properties that have been added to the new state compared to the previous state.
const TreeChanges = require('tree-changes');
const prevState = { a: 1 };
const nextState = { a: 1, b: 2 };
const changes = new TreeChanges(prevState, nextState);
console.log(changes.added()); // { b: 2 }
Detect Updated Properties
This feature allows you to detect properties that have been updated in the new state compared to the previous state.
const TreeChanges = require('tree-changes');
const prevState = { a: 1 };
const nextState = { a: 2 };
const changes = new TreeChanges(prevState, nextState);
console.log(changes.updated()); // { a: 2 }
Detect Removed Properties
This feature allows you to detect properties that have been removed from the new state compared to the previous state.
const TreeChanges = require('tree-changes');
const prevState = { a: 1, b: 2 };
const nextState = { a: 1 };
const changes = new TreeChanges(prevState, nextState);
console.log(changes.removed()); // { b: 2 }
The deep-diff package provides a way to find the differences between two JavaScript objects. It supports nested objects and arrays, making it more versatile for complex data structures. Compared to tree-changes, deep-diff offers more granular control over the types of differences detected, such as additions, deletions, and modifications.
The diff package is a general-purpose text and object diffing library. It can be used to compare strings, arrays, and objects, and provides detailed information about the differences. While it is more comprehensive than tree-changes, it may be overkill for simple state change detection.
The immutability-helper package is designed to help with updating immutable data structures. It provides a set of commands to apply changes to an object without mutating it. While it doesn't directly compare states like tree-changes, it is useful for managing state updates in a predictable way.
Compare changes between two datasets.
npm install tree-changes
import treeChanges from 'tree-changes';
const previousData = {
hasData: false,
sort: {
data: [{ type: 'asc' }, { type: 'desc' }],
status: 'idle',
},
};
const newData = {
hasData: true,
sort: {
data: [{ type: 'desc' }, { type: 'asc' }],
status: 'success',
},
};
const { changed, changedFrom } = treeChanges(previousData, newData);
changed(); // true
changed('hasData'); // true
changed('hasData', true); // true
changed('hasData', true, false); // true
// support nested matches. with dot notation
changed('sort.data.0.type', 'desc'); // true
// works with array values too
changed('sort.status', ['done', 'success']); // true
// if you only need to know the previous value
changedFrom('sort.status', 'idle'); // true
import treeChanges from 'tree-changes';
const { changed } = treeChanges([0, { id: 2 }], [0, { id: 4 }]);
changed(); // true
changed(0); // false
changed(1); // true
changed('1.id', 4); // true
This library uses @gilbarbara/deep-equal to compare properties.
added(key: Key
, value?: Value
)
Check if something was added to the data.
Works with arrays and objects (using Object.keys).
import treeChanges from 'tree-changes';
const previousData = {
actions: {},
messages: [],
};
const newData = {
actions: { complete: true },
messages: ['New Message'],
sudo: true,
};
const { added } = treeChanges(previousData, newData);
added(); // true
added('actions'); // true
added('messages'); // true
added('sudo'); // true
changed(key?: Key
, actual?: Value
, previous?: Value
)
Check if the data has changed.
It also can compare to the actual
value or even with the previous
.
changedFrom(key: Key
, previous: Value
, actual?: Value
)
Check if the data has changed from previous
or from previous
to actual
.
decreased(key: Key
, actual?: Value
, previous?: Value
)
Check if both values are numbers and the value has decreased.
It also can compare to the actual
value or even with the previous
.
import treeChanges from 'tree-changes';
const previousData = {
ratio: 0.9,
retries: 0,
};
const newData = {
ratio: 0.5,
retries: 1,
};
const { decreased } = treeChanges(previousData, newData);
decreased('ratio'); // true
decreased('retries'); // false
emptied(key: Key
)
Check if the data was emptied. Works with arrays, objects and strings.
import treeChanges from 'tree-changes';
const previousData = {
data: { a: 1 },
items: [{ name: 'test' }],
missing: 'username',
};
const newData = {
data: {},
items: [],
missing: '',
};
const { emptied } = treeChanges(previousData, newData);
emptied('data'); // true
emptied('items'); // true
emptied('missing'); // true
filled(key: Key
)
Check if the data was filled (from a previous empty value). Works with arrays, objects and strings.
import treeChanges from 'tree-changes';
const previousData = {
actions: {},
messages: [],
username: '',
};
const newData = {
actions: { complete: true },
messages: ['New Message'],
username: 'John',
};
const { filled } = treeChanges(previousData, newData);
filled('actions'); // true
filled('messages'); // true
filled('username'); // true
increased(key: Key
, actual?: Value
, previous?: Value
)
Check if both values are numbers and the value has increased.
It also can compare to the actual
value or even with the previous
.
import treeChanges from 'tree-changes';
const previousData = {
ratio: 0.9,
retries: 0,
};
const newData = {
ratio: 0.5,
retries: 1,
};
const { increased } = treeChanges(previousData, newData);
increased('retries'); // true
increased('ratio'); // false
removed(key: Key
, value?: Value
)
Check if something was removed from the data.
Works with arrays and objects (using Object.keys).
import treeChanges from 'tree-changes';
const previousData = {
data: { a: 1 },
items: [{ name: 'test' }],
switch: false,
};
const newData = {
data: {},
items: [],
};
const { removed } = treeChanges(previousData, newData);
removed(); // true
removed('data'); // true
removed('items'); // true
removed('switch'); // true
Types
type Key = string | number;
type ValidTypes = string | boolean | number | Record<string, any> };
type Value = ValidTypes | ValidTypes[];
MIT
FAQs
Get changes between two versions of data with similar shape
The npm package tree-changes receives a total of 289,865 weekly downloads. As such, tree-changes popularity was classified as popular.
We found that tree-changes demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.