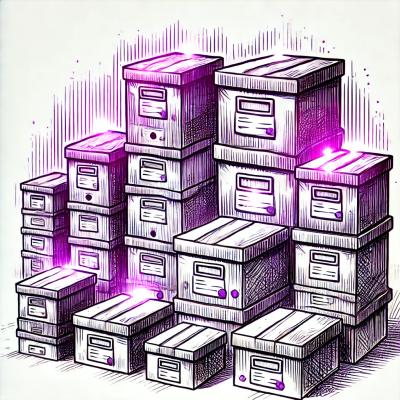
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
trtc-electron-plugin-xmagic
Advanced tools
This is a plugin used to integrate Tencent Effect SDK into Tencent TRTC Electron SDK.
This is a plugin used to integrate Tencent Effect SDK into Tencent TRTC Electron SDK.
npm install trtc-electron-sdk
npm install trtc-electron-plugin-xmagic
// beauty.js
import TRTCXmagicFactory, {
TRTCXmagicEffectConstant,
TRTCXmagicEffectValueType,
} from "trtc-electron-plugin-xmagic";
export {
TRTCXmagicEffectConstant,
TRTCXmagicEffectValueType,
TRTCXmagicEffectCategory,
default as TRTCXmagicFactory
} from "trtc-electron-plugin-xmagic";
// To do
function async getAppPath() {
// get app path from Electron main process use app.getAppPath()
}
async function init() {
const appPath = await getAppPath();
TRTCXmagicFactory.init(appPath);
}
init();
const { platform } = process;
const lutResourceList = [
{
label: "清晰",
label_en: "Refreshing",
resPath: "lut.bundle/moren_lf.png",
icon: "./assets/beauty_panel/panel_icon/lut_icon/moren_lf.png",
},
{
label: "自然",
label_en: "Natural",
resPath: "lut.bundle/n_ziran.png",
icon: "./assets/beauty_panel/panel_icon/lut_icon/ziran_lf.png",
},
{
label: "心动",
label_en: "Allure",
resPath: "lut.bundle/xindong_lf.png",
icon: "./assets/beauty_panel/panel_icon/lut_icon/xindong_lf.png",
},
]
// Lut(Filter)
TRTCXmagicEffectConstant["EFFECT_LUT"].options = TRTCXmagicEffectConstant["EFFECT_LUT"].options.concat(
lutResourceList.map(item => {
return {
...TRTCXmagicEffectConstant["EFFECT_LUT"].valueMeta.value,
...item,
}
})
);
// Makeup
TRTCXmagicEffectConstant["EFFECT_MAKEUP"].options = TRTCXmagicEffectConstant["EFFECT_MAKEUP"].options.concat([
{
label: "桃花",
label_en: "Peach",
effKey: platform === "win32" ? "makeup.strength" : "video_fenfenxia",
valueType: TRTCXmagicEffectValueType.ZERO_ONE,
resPath:
platform === "win32"
? "makeupMotionRes.bundle/video_fenfenxia/template.json"
: "makeupMotionRes.bundle/video_fenfenxia",
icon: "./assets/beauty_panel/panel_icon/makeup_icon/video_fenfenxia.png"
},
{
label: "晒伤",
label_en: "Sunburn",
effKey: platform === "win32" ? "makeup.strength" : "video_shaishangzhuang",
valueType: TRTCXmagicEffectValueType.ZERO_ONE,
resPath:
platform === "win32"
? "makeupMotionRes.bundle/video_shaishangzhuang/template.json"
: "makeupMotionRes.bundle/video_shaishangzhuang",
icon: "./assets/beauty_panel/panel_icon/makeup_icon/video_shaishangzhuang.png"
},
]);
// Motion
TRTCXmagicEffectConstant["EFFECT_MOTION"]["2dOptions"] = TRTCXmagicEffectConstant["EFFECT_MOTION"]["2dOptions"].concat([
{
label: "兔兔酱",
label_en: "Bunny"
effKey: "video_tutujiang",
resPath:
platform === "win32"
? "2dMotionRes.bundle/video_tutujiang/template.json"
: "2dMotionRes.bundle",
icon: "./assets/beauty_panel/panel_icon/motions_icon/video_tutujiang.png",
valueType: TRTCXmagicEffectValueType.NONE,
},
]);
TRTCXmagicEffectConstant["EFFECT_MOTION"]["3dOptions"] = TRTCXmagicEffectConstant["EFFECT_MOTION"]["3dOptions"].concat([
{
label: "知性玫瑰",
label_en: "Glasses",
effKey: "video_zhixingmeigui",
resPath:
platform === "win32"
? "3dMotionRes.bundle/video_zhixingmeigui/template.json"
: "3dMotionRes.bundle",
icon:"./assets/beauty_panel/panel_icon/motions_icon/video_zhixingmeigui.png",
valueType: TRTCXmagicEffectValueType.NONE,
},
]);
TRTCXmagicEffectConstant["EFFECT_MOTION"]["handOptions"] = TRTCXmagicEffectConstant["EFFECT_MOTION"]["handOptions"].concat([
{
label: "樱花女孩",
label_en: "Sakura",
effKey: "video_sakuragirl",
resPath:
platform === "win32"
? "handMotionRes.bundle/video_sakuragirl/template.json"
: "handMotionRes.bundle",
icon:"./assets/beauty_panel/panel_icon/motions_icon/video_sakuragirl.png",
valueType: TRTCXmagicEffectValueType.NONE,
},
]);
const virtualBackgroundImageList = [
{
label: "黑板",
label_en: "Blackboard",
icon: "./virtual-bg/黑板.jpg",
}
]
// Virtual background
TRTCXmagicEffectConstant["EFFECT_SEGMENTATION"].options = TRTCXmagicEffectConstant["EFFECT_SEGMENTATION"].options.concat(
virtualBackgroundImageList.map(item => {
return {
...TRTCXmagicEffectConstant["EFFECT_SEGMENTATION"].valueMeta.background,
...item,
}
})
);
export const customEffectConstant = TRTCXmagicEffectConstant;
// Room.vue script
import TRTCCloud, { TRTCPluginType, TRTCVideoPixelFormat } from 'trtc-electron-sdk';
import { customEffectConstant, TRTCXmagicFactory } from './beauty.js';
const trtcCloud = TRTCCloud.getTRTCShareInstance();
const startBeauty = () {
// Enable beauty effect
trtcCloud.setPluginParams(TRTCPluginType.TRTCPluginTypeVideoProcess, {
enable: true,
pixelFormat: TRTCVideoPixelFormat.TRTCVideoPixelFormat_I420
});
// Regist beauty plugin callback
trtcCloud.setPluginCallback((pluginId, errorCode, msg) => {
console.log(`plugin info: ${pluginId}, errorCode: ${errorCode}, msg: ${msg}`);
});
const currentCamera = trtcCloud.getCurrentCameraDevice(); // Make sure your PC has a camera
if (currentCamera) {
const libPath = await TRTCXmagicFactory.getEffectPluginLibPath();
beautyPlugin = trtcCloud.addPlugin({
id: `${currentCamera.deviceId}-${new Date().getTime()}`, // ID shoud be unique globally
path: libPath,
type: TRTCPluginType.TRTCPluginTypeVideoProcess,
});
const initParam = await TRTCXmagicFactory.buildEffectInitParam({
licenseURL: "", // --- IMPORTANT --- To do: input you license URL
icenseKey: "", // --- IMPORTANT --- To do: input you license Key
});
beautyPlugin.setParameter(JSON.stringify(initParam));
beautyPlugin.enable();
const beautyEffectConstants = TRTCXmagicFactory.getEffectConstant(customEffectConstant);
const properties1 = TRTCXmagicFactory.buildEffectProperty({
...beautyEffectConstants.beauty.details[0],
effValue: 89,
});
const properties2 = TRTCXmagicFactory.buildEffectProperty({
...beautyEffectConstants.makeup.details[0],
effValue: 0,
});
const properties3 = TRTCXmagicFactory.buildMakeupEffectProperty({
...beautyEffectConstants.makeup.details[0],
effValue: 70,
});
const beautySetting = {
beautySetting: [...properties1, ...properties2, ...properties3]
};
const jsonParam = JSON.stringify(beautySetting);
beautyPlugin.setParameter(jsonParam);
}
}
// electron builder configuration related
"build": {
"win": {
"target": ["nsis","zip"],
"extraFiles": [
{
"from": "node_modules/trtc-electron-sdk/build/Release/",
"to": "./resources",
"filter": [
"**/*"
]
},
{
"from": "node_modules/trtc-electron-plugin-xmagic/plugin/XMagic/win/${arch}/platforms/",
"to": "./platforms",
"filter": [
"**/*"
]
},
{
"from": "node_modules/trtc-electron-plugin-xmagic/plugin/XMagic/win/${arch}/",
"to": "./resources/app/plugin/XMagic/win/${arch}/",
"filter": [
"**/*"
]
},
{
"from": "node_modules/trtc-electron-plugin-xmagic/plugin/XMagic/win/res/",
"to": "./resources/app/plugin/XMagic/win/res/",
"filter": [
"**/*"
]
}
]
},
"mac": {
"target": ["dmg"],
"extraFiles": [
{
"from": "node_modules/trtc-electron-sdk/build/Release/${arch}/trtc_electron_sdk.node",
"to": "./Resources"
},
{
"from": "node_modules/trtc-electron-sdk/build/mac-framework/${arch}/",
"to": "./Frameworks"
},
{
"from": "node_modules/trtc-electron-plugin-xmagic/plugin/XMagic/mac/",
"to": "./Resources/app/plugin/XMagic/mac/"
}
]
},
}
FAQs
This is a plugin used to integrate Tencent Effect SDK into Tencent TRTC Electron SDK.
The npm package trtc-electron-plugin-xmagic receives a total of 4 weekly downloads. As such, trtc-electron-plugin-xmagic popularity was classified as not popular.
We found that trtc-electron-plugin-xmagic demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.