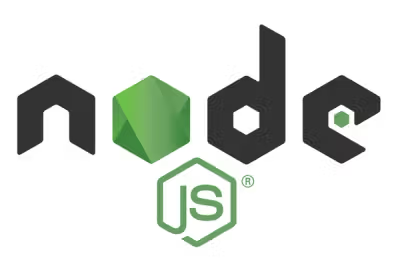
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
truedata-nodejs
Advanced tools
npm install truedata-nodejs
const { rtConnect, rtDisconnect, rtSubscribe, rtUnsubscribe, rtFeed, historical, formatTime, isSocketConnected } = require('truedata-nodejs')
const user = 'your username'
const pwd = 'your password'
const port = 8082
const symbols = ['NIFTY-I', 'BANKNIFTY-I', 'CRUDEOIL-I']; // symbols in array format
rtConnect(user, pwd, symbols, port, bidask = 1, heartbeat = 1, replay = 0, url = 'push');
rtFeed.on('touchline', touchlineHandler); // Receives Touchline Data
rtFeed.on('tick', tickHandler); // Receives Tick data
rtFeed.on('greeks', greeksHandler); // Receives Greeks data
rtFeed.on('bidask', bidaskHandler); // Receives Bid Ask data if enabled
rtFeed.on('bidaskL2', bidaskL2Handler); // Receives level 2 Bid Ask data only for BSE exchange
rtFeed.on('bar', barHandler); // Receives 1min and 5min bar data
rtFeed.on('marketstatus', marketStatusHandler); // Receives marketstatus messages
rtFeed.on('heartbeat', heartbeatHandler); // Receives heartbeat message and time
function touchlineHandler(touchline){
console.log(touchline)
}
function tickHandler(tick){
console.log(tick)
}
function greeksHandler(greeks){
console.log(greeks)
}
function bidaskHandler(bidask){
console.log(bidask)
}
function bidaskL2Handler(bidaskL2){
console.log(bidaskL2)
}
function barHandler(bar){
console.log(bar)
}
function marketStatusHandler(status) {
console.log(status);
}
function heartbeatHandler(heartbeat) {
console.log(heartbeat);
}
| Default values | Available values
| -------------- | ----------------
| bidask = 1 | 0, 1 // 1 = bidask active, 0 = bidask inactive
| heartbeat = 1 | 0, 1 // 1 = print heartbeat message, 0 = don't print heartbeat message
| replay = 0 | 0, 1 // 1 = Feed replay, 0 = Normal feed ( default - If parameter not entered then replay = 0 )
| url = 'push' | 'push'
isSocketConnected() // Check if socket is connected or not. Returns Boolean value
getMarketStatus() // Return the current market status
rtSubscribe( newSymbols ) // Dynamically subscribe to new symbols // newSymbols is array of symbol
rtUnsubscribe( oldSymbols ) // Dynamically unsubscribe from currently subscribed symbols // oldSymbols is array of symbol
rtDisconnect() // Disconnect live data feed and close socket connection
The library will check for internet connection and once steady will try to re-connect the Websocket.
historical.auth(user, pwd); // For authentication.
from = formatTime(2021, 3, 2, 9, 15) // (year, month, date, hour, minute) // hour in 24 hour format
to = formatTime(2021, 3, 5, 9, 15) // (year, month, date, hour, minute) // hour in 24 hour format
All API calls returns a promise. You can use .then(...)
and .catch(...)
OR async
and await
method
You can use “duration” instead of using “from and to” in both the above functions. If you are using “duration” then, the function will be like:
| Default values | Available values
| -------------- | ----------------
| response = 'json' | response : 'json', 'csv'
| bidask = 1 | bidask: 1, 0 // 1 – bidask feed active , 0 – bidask feed inactive
| interval = '1min' | interval:'1min', '2min', '3min', '5min', '10min', '15min', '30min', '60min', 'EOD'
| nticks = 2000 | nticks: Any number // Max upto last 5 days of ticks
| nbars = 200 | nbars: Any number
| getSymbolId = 0 | getSymbolId : 1, 0 // 1 - symbolId active , 0 - symbolId inactive
| segment = 'FO' | segment: 'FO', 'EQ', 'MCX', 'BSEFO', 'BSEEQ'
| topsegment = 'NSEFUT' | topsegment: 'NSEFUT', 'NSEEQ', 'NSEOPT', 'CDS', 'MCX'
| top = 50 | top: Any number
*symbol in historical api is symbol name. For eg: 'NIFTY-I' or 'RELIANCE' etc.
*In historical.getBhavCopy, current date is default. Date format is 'yyyy-mm-dd'
historical
.getBarData('NIFTY-I', '210302T09:00:00', '210302T15:30:00', interval = '1min', response ='json', getSymbolId = 0 )
.then((res) => console.log(res))
.catch((err) => console.log(err));
historical
.getBarData('NIFTY-I', duration = '3W', interval = '1min', response ='json', getSymbolId = 0)
.then((res) => console.log(res))
.catch((err) => console.log(err));
const { rtConnect, rtSubscribe, rtUnsubscribe, rtFeed, historical, formatTime } = require('truedata-nodejs');
const user = 'your username';
const pwd = 'your password';
const port = 8082;
const symbols = ['NIFTY-I', 'BANKNIFTY-I', 'CRUDEOIL-I']; // symbols in array format
rtConnect(user, pwd, symbols, port, (bidask = 1), (heartbeat = 1));
rtFeed.on('touchline', touchlineHandler);
rtFeed.on('tick', tickHandler);
rtFeed.on('greeks', greeksHandler);
rtFeed.on('bidask', bidaskHandler);
rtFeed.on('bidaskL2', bidaskL2Handler);
rtFeed.on('bar', barHandler);
rtFeed.on('marketstatus', marketStatusHandler)
rtFeed.on('heartbeat', heartbeatHandler);
function touchlineHandler(touchline) {
console.log(touchline);
}
function tickHandler(tick) {
console.log(tick);
}
function greeksHandler(greeks){
console.log(greeks)
}
function bidaskHandler(bidask) {
console.log(bidask);
}
function bidaskL2Handler(bidaskL2){
console.log(bidaskL2)
}
function barHandler(bar) {
console.log(bar);
}
function marketStatusHandler(status) {
console.log(status);
}
function heartbeatHandler(heartbeat) {
console.log(heartbeat);
}
historical.auth(user, pwd); // For authentication
from = formatTime(2021, 3, 2, 9, 15); // (year, month, date, hour, minute) // hour in 24 hour format
to = formatTime(2021, 3, 5, 9, 15); // (year, month, date, hour, minute) // hour in 24 hour format
historical
.getBarData('NIFTY-I', '210302T09:00:00', '210302T15:30:00', (interval = '1min'), (response = 'json'), (getSymbolId = 0))
.then((res) => console.log(res))
.catch((err) => console.log(err));
historical
.getBarData('RELIANCE', from, to, (interval = '1min'), (response = 'json'), (getSymbolId = 0))
.then((res) => console.log(res))
.catch((err) => console.log(err));
historical
.getBarData('NIFTY 50', (duration = '1W'), (interval = '60min'), (response = 'json'), (getSymbolId = 0))
.then((res) => console.log(res))
.catch((err) => console.log(err));
historical
.getTickData('SBIN', '1D', (bidask = 1), (response = 'csv'), (getSymbolId = 0))
.then((res) => console.log(res))
.catch((err) => console.log(err));
historical
.getLTP('L&TFH')
.then((res) => console.log(res))
.catch((err) => console.log(err));
FAQs
Truedata's Official Nodejs Package
The npm package truedata-nodejs receives a total of 0 weekly downloads. As such, truedata-nodejs popularity was classified as not popular.
We found that truedata-nodejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.