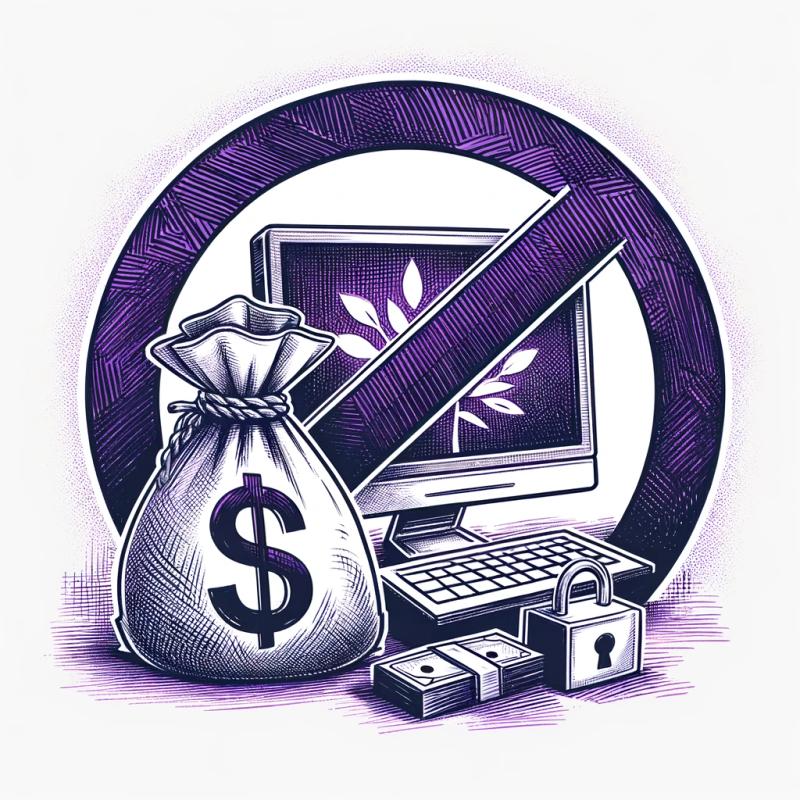
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
truffle-assertions
Advanced tools
Readme
This package adds additional assertions that can be used to test Ethereum smart contracts inside Truffle tests.
truffle-assertions can be installed through npm:
npm install truffle-assertions
To use this package, import it at the top of the Truffle test file:
const truffleAssert = require('truffle-assertions');
The eventEmitted
assertion checks that an event with type eventType has been emitted by the transaction with result result. A filter function can be passed along to further specify requirements for the event arguments:
truffleAssert.eventEmitted(result, 'TestEvent', (ev) => {
return ev.param1 === 10 && ev.param2 === ev.param3;
});
When the filter parameter is set to null, the assertion checks just for event type:
truffleAssert.eventEmitted(result, 'TestEvent', null);
The eventNotEmitted
assertion checks that an event with type eventType has not been emitted by the transaction with result result. A filter function can be passed along to further specify requirements for the event arguments:
truffleAssert.eventNotEmitted(result, 'TestEvent', (ev) => {
return ev.param1 === 10 && ev.param2 === ev.param3;
});
When the filter parameter is set to null, the assertion checks just for event type:
truffleAssert.eventNotEmitted(result, 'TestEvent', null);
FAQs
Additional assertions and utilities for testing Ethereum smart contracts in Truffle unit tests
We found that truffle-assertions demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).