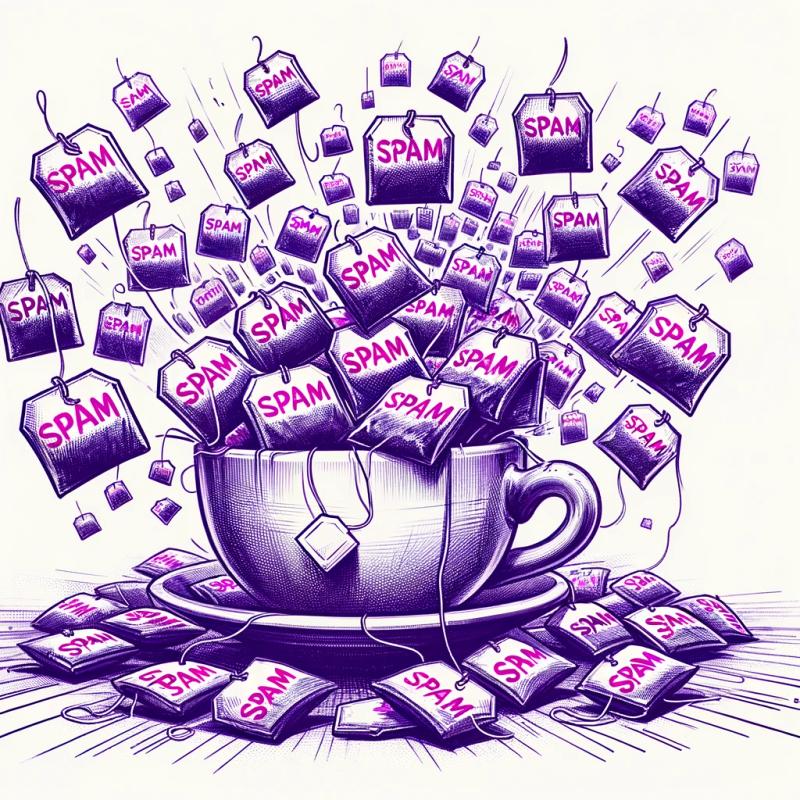
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ts-auto-guard-err
Advanced tools
Readme
Generate type guard functions from TypeScript interfaces
A tool for automatically generating TypeScript type guards for interfaces in your code base.
This tool aims to allow developers to verify data from untyped sources to ensure it conforms to TypeScript types. For example when initializing a data store or receiving structured data in an AJAX response.
$ yarn add -D ts-auto-guard
$ npm install --save-dev ts-auto-guard
Specify which types to process (see below) and run the CLI tool in the same folder as your project's tsconfig.json
(optionally passing in paths to the files you'd like it to parse).
$ ts-auto-guard ./my-project/Person.ts
See generated files alongside your annotated files:
// my-project/Person.guard.ts
import { Person } from './Person'
export function isPerson(obj: any): obj is Person {
return (
typeof obj === 'object' &&
typeof obj.name === 'string' &&
(typeof obj.age === 'undefined' || typeof obj.age === 'number') &&
Array.isArray(obj.children) &&
obj.children.every(e => isPerson(e))
)
}
Now use in your project:
// index.ts
import { Person } from './Person'
import { isPerson } from './Person.guard'
// Loading up an (untyped) JSON file
const person = require('./person.json')
if (isPerson(person)) {
// Can trust the type system here because the object has been verified.
console.log(`${person.name} has ${person.children.length} child(ren)`)
} else {
console.error('Invalid person.json')
}
Annotate interfaces in your project or pass. ts-auto-guard will generate guards only for interfaces with a @see {name} ts-auto-guard:type-guard
JSDoc tag.
// my-project/Person.ts
/** @see {isPerson} ts-auto-guard:type-guard */
export interface Person {
// !do not forget to export - only exported types are processed
name: string
age?: number
children: Person[]
}
Use --export-all
parameter to process all exported types:
$ ts-auto-guard --export-all
Use debug mode to help work out why your type guards are failing in development. This will change the output type guards to log the path, expected type and value of failing guards.
$ ts-auto-guard --debug
isPerson({ name: 20, age: 20 })
// stderr: "person.name type mismatch, expected: string, found: 20"
ts-auto-guard also supports a shortcircuit
flag that will cause all guards
to always return true
.
$ ts-auto-guard --shortcircuit="process.env.NODE_ENV === 'production'"
This will result in the following:
// my-project/Person.guard.ts
import { Person } from './Person'
export function isPerson(obj: any): obj is Person {
if (process.env.NODE_ENV === 'production') {
return true
}
return (
typeof obj === 'object' &&
// ...normal conditions
)
}
Using the shortcircuit
option in combination with uglify-js's dead_code
and global_defs
options will let you omit the long and complicated checks from your production code.
Use --return-errors flag to create an extra function that will not acts as typesafe guards, but output an array of appropriate errors (the same errors as given in the debug mode). Intended use is for example integrity testing non typesafe database outputs, that can then be printed to the screen.
$ ts-auto-guard --return-errors
// Generic usage
isObjErrorOut(obj): errors: Error[]
const returnVar = isPersonErrorOut({ name: "John", age: 20 })
// returnVar.length: 0
const returnVar = isPersonErrorOut({ name: 20, age: 20 })
// returnVar[0].message: "person.name type mismatch, expected: string, found: 20"
FAQs
Generate type guard functions from TypeScript interfaces
The npm package ts-auto-guard-err receives a total of 0 weekly downloads. As such, ts-auto-guard-err popularity was classified as not popular.
We found that ts-auto-guard-err demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.