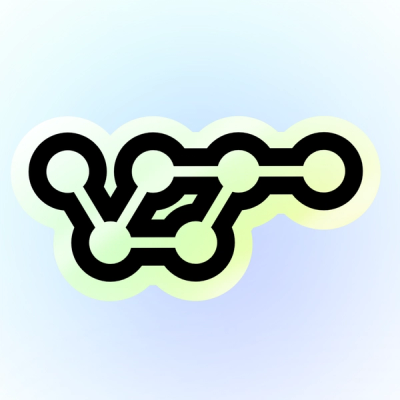
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
ts-jest is an npm package that allows users to run tests written in TypeScript directly, without having to precompile them to JavaScript. It is a Jest transformer with source map support that lets you use Jest to test projects written in TypeScript.
TypeScript testing
This feature allows you to write Jest tests in TypeScript. The code sample demonstrates a simple test for a sum function.
import sum from './sum';
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Source map support
Source map support for accurate stack traces in error messages, which is useful for debugging tests.
/* Source maps are automatically handled by ts-jest, so there's no specific code sample for this feature. It works under the hood to provide accurate stack traces in your tests. */
TypeScript configuration
Allows you to use your project's TypeScript configuration or specify a custom one for testing purposes.
/* ts-jest uses the tsconfig.json file in your project to understand how to compile your TypeScript code. You can also specify a different configuration file for ts-jest if needed. */
Coverage reports
Integrates with Jest's coverage reporting to include TypeScript files in coverage statistics.
/* To collect coverage, you can use Jest's built-in coverage collection feature with ts-jest. */
jest --coverage
babel-jest is a Jest plugin that allows you to use Babel to transform your JavaScript code. It is similar to ts-jest but is focused on JavaScript with Babel transformations rather than TypeScript.
Jest itself is a testing framework that can work with TypeScript when configured with the appropriate preprocessor (like ts-jest). It provides the overall testing framework that ts-jest plugs into.
Mocha is another testing framework that can be used with TypeScript when combined with a TypeScript compiler like ts-node. It is an alternative to Jest and thus to ts-jest, but it requires additional setup for working with TypeScript.
karma-typescript is a Karma plugin that compiles and bundles TypeScript on the fly. It is similar to ts-jest in that it allows for testing TypeScript code, but it is designed to work within the Karma test runner ecosystem.
Note: This is currently just a hack and might not be suitable for all setups.
Note: This repo uses code from the source-map-support package to show errors with mapped locations.
To use this in your project, run:
npm install --save-dev ts-jest
Modify your project's package.json
so that the jest
section looks something like:
{
"jest": {
"scriptPreprocessor": "<rootDir>/node_modules/ts-jest/preprocessor.js",
"testRegex": "(/__tests__/.*|\\.(test|spec))\\.(ts|tsx|js)$",
"moduleFileExtensions": [
"ts",
"tsx",
"js"
]
}
}
This setup should allow you to write Jest tests in Typescript and be able to locate errors without any additional gymnastics.
By default jest
does not provide code coverage remapping for transpiled codes, so if you'd like to have code coverage it needs additional coverage remapping. This can be done via writing custom processing script, or configure testResultsProcessor
to use built-in coverage remapping in ts-jest
.
{
"jest": {
"scriptPreprocessor": "<rootDir>/node_modules/ts-jest/preprocessor.js",
"testResultsProcessor": "<rootDir>/node_modules/ts-jest/coverageprocessor.js"
}
}
Note: If you're experiencing remapping failure with source lookup, it may due to pre-created cache from
jest
. It can be manually deleted, or execute with--no-cache
to not use those.
By default this package will try to locate tsconfig.json
and use its compiler options for your .ts
and .tsx
files.
But you are able to override this behaviour and provide another path to your config for TypeScript by using __TS_CONFIG__
option in globals
for jest
:
{
"jest": {
"globals": {
"__TS_CONFIG__": "my-tsconfig.json"
}
}
}
Or even declare options for tsc
instead of using separate config, like this:
{
"jest": {
"globals": {
"__TS_CONFIG__": {
"module": "commonjs",
"jsx": "react"
}
}
}
}
For all available options see TypeScript docs.
If you have any suggestions/pull requests to turn this into a useful package, just open an issue and I'll be happy to work with you to improve this.
git clone https://github.com/kulshekhar/ts-jest
cd ts-jest
npm install
npm test
Copyright (c) Authors.
This source code is licensed under the MIT license.
0.1.9 (2016-10-24)
<a name="0.1.8"></a>
FAQs
A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript
The npm package ts-jest receives a total of 6,293,917 weekly downloads. As such, ts-jest popularity was classified as popular.
We found that ts-jest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.