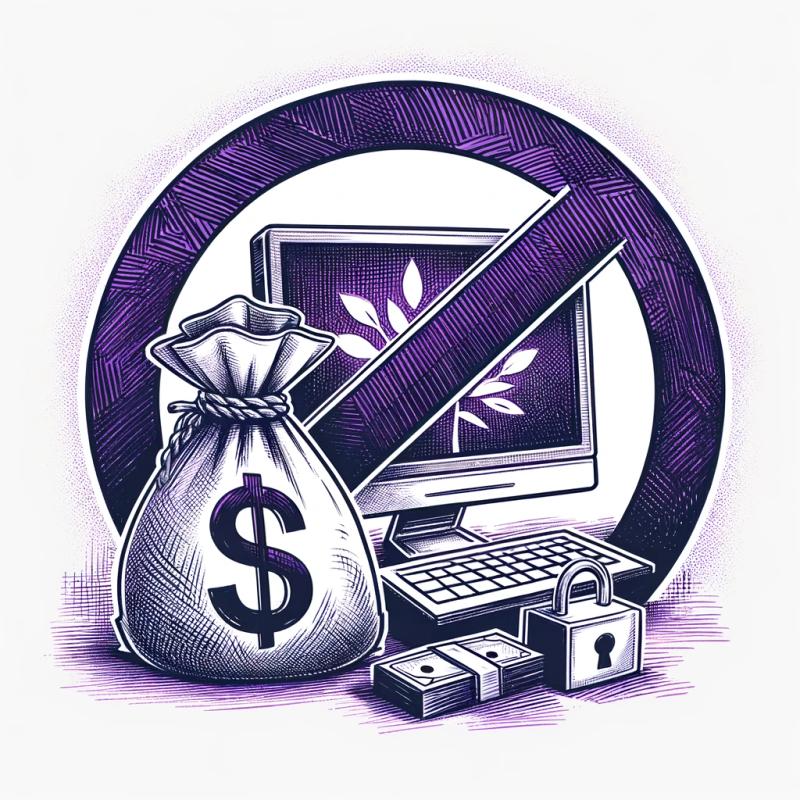
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
ts-map
Advanced tools
Readme
it is a Map structure like ES6 Map. Map is similar to the object, but also a set of key-value pairs, but the "key" range is not limited to strings, various types of values (including objects) can be used as a key.
npm install ts-map
use in typescript file
import TsMap from 'ts-map'
const map = new TsMap()
const k1: number = 1
const k2: number[] = [2]
const k3: boolean = true
map.set(1, "hello")
map.set(k2, "ts").set(k3, "map")
map.get(1) // "hello"
map.get(k2) // "world"
map.size // 3
map.keys() // [1, [2], true]
map.values() // ["hello", "ts", "map"]
map.forEach((value, key, map) => {
console.log(key, ':', value)
})
// 1 ':' 'hello'
// [ 2 ] ':' 'ts'
// true ':' 'map'
You can pass in the default parameters in the constructor:
const map = new TsMap([
[1, "ok"],
[2, "fail"]
])
console.log(map.get(1)) // ok
support define generic for ts-map
interface Coder {
name: string
}
const map = new TsMap<number, Coder>([
[1, {name: 'lavyun'}]
])
map.set(2, {name: "tom"}) // work
map.set(3, "jack") // sorry, error
If you do not define generics, but in the constructor passed in the parameters, you also need follow the generic rules.If you do not use generics, you can set any type of key-value pairs for the map.
return the Map's size
const map = new TsMap<number, Coder>([
[1, {name: 'lavyun'}]
])
map.set(2, {name: "tom"})
map.size // 2
set a key-value to Map, support chain called.
map.set(true, "1")
map.set(1, "hello").set(2, "world")
Notice: Only the reference to the same object, Map structure will be regarded as the same key.
const k = ["1"]
map.set(k, "hello")
map.get(k) // hello
map.get(["1"]) // undefind
If the same key is assigned multiple times, the following value will overwrite the previous value.
map.set(1, "111").set(1, "222")
map.get(1) // 222
Return the value of the corresponding key,if dosn't include, return undefind.
map.set(1, "111")
map.get(1) // 111
map.get(2) // undefind
Determine if a key is included.
map.set(1, "111")
map.has(1) // true
map.has(2) // false
Delete all the corresponding keys and its value, if detele success, return true. else return false.
map.set(1, "111")
map.set(2, "222")
map.delete(1)
map.has(1) //false
mao.size // 1
Delete all key-value from the Map.
map.set(1, "111")
map.set(2, "222")
map.size // 2
map.clear()
map.size // 0
return all Map's key.
map.set(1, 2)
map.set(true, false)
map.set(["1"], {name: 'lavyun'})
map.keys() // [1, true, ["1"]]
return all Map's value.
map.set(1, 2)
map.set(true, false)
map.set(["1"], {name: 'lavyun'})
map.values() // [2, false, 'lavyun']
return all Map's key-value.
map.set(1, 2)
map.set(true, false)
map.set(["1"], {name: 'lavyun'})
map.entries()
/*
[
[1, 2],
[true, false],
[["1"], {name: 'lavyun'}]
]
*/
Traversal the Map.Accept two parameters, first is a callback, second is a optional context.
callback function accepts 3 optional params,first is value, second is key, last is the map.
map.set(1, "111").set(2. "222")
map.forEach((value, key, map) => {
console.log(key, '-', value)
})
// 1 - '111'
// 2 - '222'
You can pass the second param to set the callback's context
const person = {
name: 'lavyun'
}
map.set(1, "111").set(2. "222")
map.forEach((value, key, map) => {
console.log(key, '-', value, '-', this.name)
}, person)
// 1 - '111' - 'lavyun'
// 2 - '222' - 'lavyun'
MIT LICENCE
FAQs
A typescript Map structure like ES6 Map
We found that ts-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).