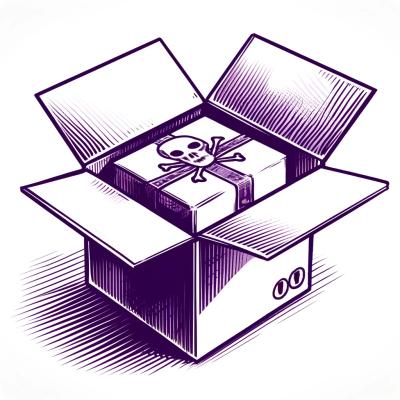
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
The ts-md5 npm package is a TypeScript implementation of the MD5 hashing algorithm. It allows you to generate MD5 hashes for strings, arrays, and other data types. This package is useful for creating checksums, verifying data integrity, and other cryptographic purposes.
Hashing a String
This feature allows you to generate an MD5 hash from a string. The `hashStr` method takes a string as input and returns its MD5 hash.
const { Md5 } = require('ts-md5');
const hash = Md5.hashStr('Hello World');
console.log(hash); // Outputs the MD5 hash of 'Hello World'
Hashing an Array
This feature allows you to generate an MD5 hash from an array of ASCII values. The `hashAsciiStr` method takes an array of ASCII values as input and returns its MD5 hash.
const { Md5 } = require('ts-md5');
const hash = Md5.hashAsciiStr([72, 101, 108, 108, 111]);
console.log(hash); // Outputs the MD5 hash of the ASCII values for 'Hello'
Incremental Hashing
This feature allows you to perform incremental hashing. You can append strings or arrays in parts and then call the `end` method to get the final MD5 hash.
const { Md5 } = require('ts-md5');
const md5 = new Md5();
md5.appendStr('Hello');
md5.appendStr(' World');
const hash = md5.end();
console.log(hash); // Outputs the MD5 hash of 'Hello World'
CryptoJS is a widely-used library that provides a variety of cryptographic algorithms, including MD5. It is more versatile than ts-md5 as it supports multiple hashing algorithms like SHA-1, SHA-256, and more.
The md5 package is a simple and straightforward implementation of the MD5 hashing algorithm. It is similar to ts-md5 but is written in plain JavaScript and does not provide TypeScript typings out of the box.
Hash.js is a library that provides a variety of hash functions, including MD5. It is more comprehensive than ts-md5 and supports other algorithms like SHA-1, SHA-256, and RIPEMD160.
A MD5 implementation for TypeScript
This library also includes tools for:
Based on work by
Install the node module with npm install ts-md5
import {Md5} from 'ts-md5';
Md5.hashStr('blah blah blah')
=> hex:stringMd5.hashStr('blah blah blah', true)
=> raw:Int32Array(4)Md5.hashAsciiStr('blah blah blah')
=> hex:stringMd5.hashAsciiStr('blah blah blah', true)
=> raw:Int32Array(4)For more complex uses:
md5 = new Md5();
// Append incrementally your file or other input
// Methods are chainable
md5.appendStr('somestring')
.appendAsciiStr('a different string')
.appendByteArray(blob);
// Generate the MD5 hex string
md5.end();
NOTE:: You have to make sure ts-md5/dist/md5_worker.js
is made available in your build so it can be accessed directly by a browser
It should always remain as a seperate file.
import {ParallelHasher} from 'ts-md5';
let hasher = new ParallelHasher('/path/to/ts-md5/dist/md5_worker.js');
hasher.hash(fileBlob).then(function(result) {
console.log('md5 of fileBlob is', result);
});
The project is written in typescript and transpiled into ES5.
npm install -g typescript
(if you haven't already)tsconfig.json
tsc
You can find more information here: https://github.com/Microsoft/TypeScript/wiki/tsconfig.json
npm run build
npm run test
package.json
https://docs.npmjs.com/files/package.jsonnpm publish
https://docs.npmjs.com/cli/publishMIT
FAQs
TypeScript MD5 implementation
We found that ts-md5 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.