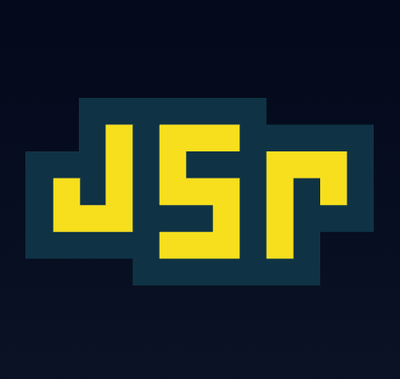
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The ts-poet npm package is a TypeScript code generation library that allows you to programmatically create TypeScript code. It is particularly useful for generating code based on some input data or schema, such as generating TypeScript types from a GraphQL schema or a protobuf definition.
Generating TypeScript Interfaces
This feature allows you to generate TypeScript interfaces programmatically. The code sample demonstrates how to create a 'User' interface with properties 'id', 'name', and 'email'.
const { Code, InterfaceSpec } = require('ts-poet');
const userInterface = InterfaceSpec.create('User')
.addProperty('id', 'number')
.addProperty('name', 'string')
.addProperty('email', 'string');
const code = Code.create(userInterface);
console.log(code.toString());
Generating TypeScript Classes
This feature allows you to generate TypeScript classes programmatically. The code sample demonstrates how to create a 'User' class with properties 'id', 'name', and 'email', and a constructor method.
const { Code, ClassSpec } = require('ts-poet');
const userClass = ClassSpec.create('User')
.addProperty('id', 'number')
.addProperty('name', 'string')
.addProperty('email', 'string')
.addMethod('constructor', 'constructor(id: number, name: string, email: string) { this.id = id; this.name = name; this.email = email; }');
const code = Code.create(userClass);
console.log(code.toString());
Generating TypeScript Functions
This feature allows you to generate TypeScript functions programmatically. The code sample demonstrates how to create a 'greet' function that takes a 'name' parameter and returns a greeting string.
const { Code, FunctionSpec } = require('ts-poet');
const greetFunction = FunctionSpec.create('greet')
.addParameter('name', 'string')
.setReturnType('string')
.setBody('return `Hello, ${name}!`;');
const code = Code.create(greetFunction);
console.log(code.toString());
The 'typescript' package is the official TypeScript compiler and language service. While it does not focus on code generation, it provides the necessary tools to parse, analyze, and transform TypeScript code, which can be used for code generation tasks.
The 'ts-morph' package is a TypeScript compiler API wrapper that simplifies working with the TypeScript AST (Abstract Syntax Tree). It provides a higher-level API for creating, navigating, and manipulating TypeScript code, making it a powerful tool for code generation and transformation tasks.
The 'codegen' package is a general-purpose code generation library that supports multiple languages, including TypeScript. It provides a flexible API for generating code structures, making it a versatile alternative to ts-poet for various code generation needs.
ts-poet is a TypeScript code generator that is really just a fancy wrapper around template literals.
Here's some example HelloWorld
output generated by ts-poet:
import { Observable } from 'rxjs/Observable';
class Greeter {
private name: string;
constructor(private name: string) {
}
greet(): Observable<string> {
return Observable.from(`Hello $name`);
}
}
And this is the code to generate it with ts-poet:
import { code, imp } from "ts-poet";
const Observable = imp("@rxjs/Observable");
const greet = code`
greet(): ${Observable}<string> {
return ${Observable}.from(\`Hello $name\`)};
}
`;
const greeter = code`
export class Greeter {
private name: string;
constructor(private name: string) {
}
${greet}
}
`;
const output = greeter.toStringWithImports("Greeter");
I.e. really all ts-poet does is:
"Auto organize imports" symbols, i.e. if you use imp
to define the modules/imports you need in your generated code, ts-poet will create the import stanza at the top of the file.
This can seem fairly minor, but it facilitates decomposition of your code generation code, so that you can have multiple levels of helper methods/etc. that can return code
template literals that embed both the code itself as well as the import types, so that when the final file is generated, ts-poet can collect and emit all of the imports.
Formats the output with prettier (using your local .prettierrc
if it exists)
Given the primary goal of ts-poet is to manage imports for you, there are several ways of specifying imports to the imp
function:
imp("Observable@rxjs")
--> import { Observable } from "rxjs"
imp("Observable@./Api")
--> import { Observable } from "./Api"
imp("Observable*./Api")
--> import * as Observable from "./Api"
imp("Observable=./Api")
--> import Observable from "./Api"
imp("@rxjs/Observable")
--> import { Observable } from "rxjs/Observable"
imp("*rxjs/Observable")
--> import * as Observable from "rxjs/Observable"
imp("@Api")
--> import { Api } from "Api"
imp("describe+mocha")
--> import "mocha"
ts-poet was originally inspired by Square's JavaPoet code generation DSL, which has a very "Java-esque" builder API of addFunction
/addProperty
/etc. that ts-poet copied in it's original v1/v2 releases.
JavaPoet's approach worked very well for the Java ecosystem, as it was providing three features:
appendLine(...).appendLine(...)
style methods.However, in the JavaScript/TypeScript world we have prettier for formatting, and nice multi-line string support via template literals, so really the only value add that ts-poet needs to provide is the "auto organize imports", which is what the post-v2/3.0 API has been rewritten (and dramatically simplified as a result) to provide.
FAQs
code generation DSL for TypeScript
The npm package ts-poet receives a total of 268,416 weekly downloads. As such, ts-poet popularity was classified as popular.
We found that ts-poet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.