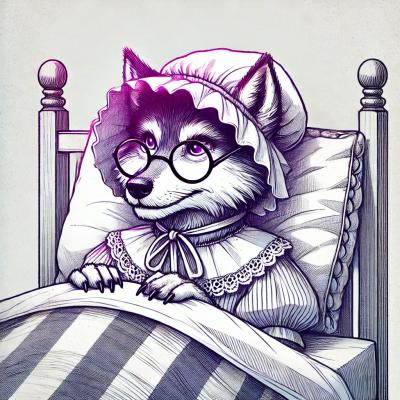
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Compose custom types containing mutually exclusive keys, using this generic Typescript helper type.
Compose custom types containing mutually exclusive keys, using this generic Typescript helper type.
Typescript's union operator (|
) allows combining two object types A
and B
, into a superset type C which can contain all the members of both A
and B
.
But sometimes the requirements dictate that we combine two types with mutually exclusive members. So take the members A.a
and B.b
. Given type C = A | B
then we want to impose the restriction that we can set either C.a
or C.b
but never both AND always at least one of the two!
Typescript does not have this feature built-in.
The package ts-xor
introduces the new custom type XOR
. You can use XOR to compose your own custom types with mutually exclusive members.
The XOR type effectively implements the well-known XOR logical operator from boolean algebra as defined by the following truth table:
A | B | Result | Note |
---|---|---|---|
0 | 0 | 0 | achievable with union operator (| ) and XOR |
0 | 1 | 1 | achievable with union operator (| ) and XOR |
1 | 0 | 1 | achievable with union operator (| ) and XOR |
1 | 1 | 0 | achievable only with XOR |
If we use the union operator
type A_OR_B = A | B
then the derived type is shown in VS Code like so:
Whereas if we use the XOR mapped type
type A_XOR_B = XOR<A, B>
then the derived type is shown quite differently in VS Code:
Notice that when using XOR each "variant" of the resulting type contains all keys of one source type plus all keys of the other, with those of the second type defined as optional and at the same time typed as undefined.
This trick will not only forbid defining keys of both source types at the same time (since the type of each key is explicitly undefined
), but also allow us to not need to define all keys all of the time since each set of keys is optional on each variant.
In your typescript powered, npm project, run:
npm install -D ts-xor # yarn add -D ts-xor
// example1.ts
import { XOR } from 'ts-xor'
interface A {
a: string
}
interface B {
b: string
}
let test: XOR<A, B>
test = { a: '' } // OK
test = { b: '' } // OK
test = { a: '', b: '' } // rejected
test = {} // rejected
Let's assume that we have the following spec for a weather forecast API's response:
id
and station
membersrain
or a member snow
, but never both at the same time.1h
or a member 3h
with a number value, but never both keys at the same time.// example2.ts
import { XOR } from 'ts-xor'
type ForecastAccuracy = XOR<{ '1h': number }, { '3h': number }>
interface WeatherForecastBase {
id: number
station: string
}
interface WeatherForecastWithRain extends WeatherForecastBase {
rain: ForecastAccuracy
}
interface WeatherForecastWithSnow extends WeatherForecastBase {
snow: ForecastAccuracy
}
type WeatherForecast = XOR<WeatherForecastWithRain, WeatherForecastWithSnow>
const test: WeatherForecast = {
id: 1,
station: 'Acropolis',
// rain: { '1h': 1 }, // OK
// rain: { '2h': 1 }, // rejected
// rain: { '3h': 1 }, // OK
// rain: {}, // rejected
// rain: { '1h': 1 , '3h': 3 }, // rejected
// lel: { '3h': 1 }, // rejected
// rain: { '3h': 1, lel: 1 }, // rejected
// snow: { '3h': 1 }, // OK
// rejected when BOTH `rain` AND `snow` keys are defined at the same time
}
If you want to create a type as the product of the logical XOR operation between multiple types (more than two), then nest the generic params.
// example1.ts
import { XOR } from 'ts-xor'
interface A {
a: string
}
interface B {
b: string
}
interface C {
c: string
}
let test: XOR<A, XOR<B, C>>
test = { a: '' } // OK
test = { b: '' } // OK
test = { c: '' } // OK
test = { a: '', c: '' } // rejected
test = {} // rejected
The library ts-xor
is fully covered with smoke, acceptance and mutation tests against the typescript compiler itself. The tests can be found inside the test
folder.
To run all tests locally, execute the following command inside your git-cloned ts-xor
folder:
npm run test
This library is published on NPM.
Distributed under the MIT license. See LICENSE.md
for more information.
This project's commits comply with the Conventional Commits guidelines.
git checkout -b feat/foobar
)git commit -am 'feat(foobar): add support for foobar tricks'
)git push origin feat/fooBar
)FAQs
Compose custom types containing mutually exclusive keys, using this generic Typescript helper type.
We found that ts-xor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.