TypeScript Standard Template Library
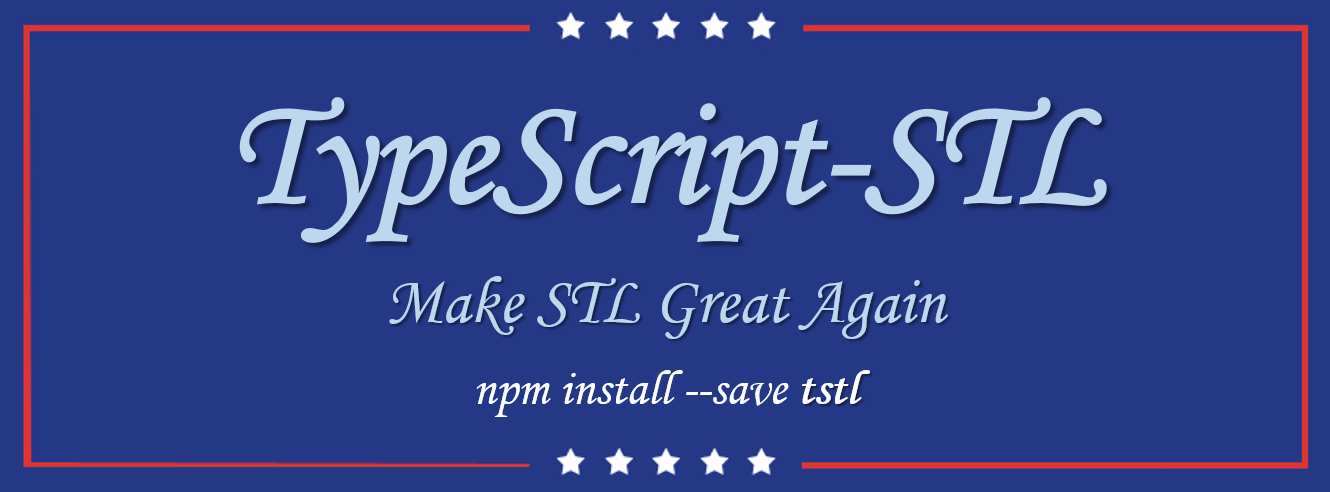
npm install --save tstl

Implementation of STL (Standard Template Library) in TypeScript.
- Containers
- Iterators
- Algorithms
- Functors
TSTL is an open-source project providing features of STL, migrated from C++ to TypeScript. You can enjoy the STL's own specific containers, algorithms and functors in the JavaScript. If TypeScript, you also can take advantage of type restrictions and generic programming with the TypeScript.
Below components are list of provided objects in the TSTL. If you want to know more about the TSTL, then please read the Guide Documents.
Features
Containers
- Linear Containers
- Associative Containers
- Tree-structured Containers
- Hash-buckets based Container
- Adaptor Containers
- Linear Adaptors
- Associative Adaptors
Algorithms
Functors
Installation
NPM Module
Installing TSTL in NodeJS is very easy. Just install with the npm
npm install --save tstl
Usage
import std from "tstl";
function main(): void
{
const map: std.TreeMap<number, string> = new std.TreeMap();
map.emplace(1, "First");
map.emplace(4, "Fourth");
map.emplace(5, "Fifth");
map.set(9, "Nineth");
for (const it of map)
console.log(it.first, it.second);
const it: std.TreeMap.Iterator<number, string> = map.lower_bound(3);
console.log(`lower bound of 3 is: ${it.first}, ${it.second}`);
}
main();
Appendix
- Repositories
- Documents
- Extensions
- ASTL - C++ STL for AssemblyScript
- ECol - Collections dispatching events
- TGrid - Network & Thread extension
- Mutex-Server - Critical sections in the network level