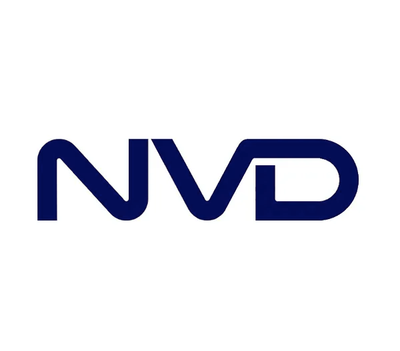
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
The tty-table npm package is used to create and display tables in the terminal. It provides a simple and flexible way to format and present tabular data in a visually appealing manner.
Basic Table Creation
This feature allows you to create a basic table with specified headers and rows. The table is then rendered and displayed in the terminal.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan' },
{ value: 'Age', width: 10, headerColor: 'cyan' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const table = ttyTable(header, rows);
console.log(table.render());
Customizing Table Appearance
This feature allows you to customize the appearance of the table, including border style, border color, padding, alignment, and text color.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan', color: 'white', align: 'left' },
{ value: 'Age', width: 10, headerColor: 'cyan', color: 'white', align: 'right' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const options = {
borderStyle: 'solid',
borderColor: 'blue',
paddingBottom: 0,
headerAlign: 'center',
align: 'center',
color: 'white'
};
const table = ttyTable(header, rows, options);
console.log(table.render());
Adding Footers
This feature allows you to add footers to the table, which can be used to display summary information or totals.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan' },
{ value: 'Age', width: 10, headerColor: 'cyan' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const footer = [
{ value: 'Total', colspan: 1, align: 'right' },
{ value: '2', align: 'right' }
];
const table = ttyTable(header, rows, { footer });
console.log(table.render());
cli-table is another npm package for creating tables in the terminal. It offers similar functionality to tty-table but with a different API. cli-table is known for its simplicity and ease of use, making it a popular choice for basic table creation.
The table package provides a more advanced and flexible way to create tables in the terminal. It supports a wide range of customization options and is suitable for more complex table layouts. Compared to tty-table, the table package offers more features but may have a steeper learning curve.
easy-table is a lightweight package for creating tables in the terminal. It focuses on simplicity and ease of use, making it a good choice for quick and straightforward table creation. While it may not offer as many customization options as tty-table, it is very easy to get started with.
Display your data in a table using a terminal, browser, or browser console.
使用终端,浏览器或浏览器控制台在表中显示您的数据
$ sudo apt-get install nodejs
$ npm install tty-table -g
$ npm install tty-table
<script src="tty-table.bundle.min.js"></script>
<script>
let Table = require('tty-table');
...
</script>
var Table = require('tty-table')('automattic-cli-table');
//now runs with same syntax as Automattic/cli-table
...
examples/styles-and-formatting.js
$ node examples/data/fake-stream.js | tty-table --format=json
$ tty-table -h
Note that neither ASCI colors nor default borders are rendered in the browser. An alternative border style, as shown below, should be used by setting the following option:
borderStyle : 2
Kind: global class
Note:
Default border character sets:
[
[
{v: " ", l: " ", j: " ", h: " ", r: " "},
{v: " ", l: " ", j: " ", h: " ", r: " "},
{v: " ", l: " ", j: " ", h: " ", r: " "}
],
[
{v: "│", l: "┌", j: "┬", h: "─", r: "┐"},
{v: "│", l: "├", j: "┼", h: "─", r: "┤"},
{v: "│", l: "└", j: "┴", h: "─", r: "┘"}
],
[
{v: "|", l: "+", j: "+", h: "-", r: "+"},
{v: "|", l: "+", j: "+", h: "-", r: "+"},
{v: "|", l: "+", j: "+", h: "-", r: "+"}
]
]
Param | Type | Description |
---|---|---|
header | array | See example |
header.column | object | Column options |
header.column.alias | string | Alternate header column name |
header.column.align | string | default: "center" |
header.column.color | string | default: terminal default color |
header.column.footerAlign | string | default: "center" |
header.column.footerColor | string | default: terminal default color |
header.column.formatter | function | Runs a callback on each cell value in the parent column |
header.column.headerAlign | string | default: "center" |
header.column.headerColor | string | default: terminal's default color |
header.column.marginLeft | number | default: 0 |
header.column.marginTop | number | default: 0 |
header.column.width | string | number | default: "auto" |
header.column.paddingBottom | number | default: 0 |
header.column.paddingLeft | number | default: 1 |
header.column.paddingRight | number | default: 1 |
header.column.paddingTop | number | default: 0 |
rows | array | See example |
options | object | Table options |
options.borderStyle | number | default: 1 (0 = no border) Refers to the index of the desired character set. |
options.borderCharacters | array | See @note |
options.borderColor | string | default: terminal's default color |
options.compact | boolean | default: false Removes horizontal lines when true. |
options.defaultErrorValue | mixed | default: 'ERROR!' |
options.defaultValue | mixed | default: '?' |
options.errorOnNull | boolean | default: false |
options.truncate | mixed | default: false When this property is set to a string, cell contents will be truncated by that string instead of wrapped when they extend beyond of the width of the cell. For example if: "truncate":"..." the cell will be truncated with "..." |
Example
let Table = require('tty-table');
let t1 = Table(header,rows,options);
console.log(t1.render());
String
Renders a table to a string
Kind: static method of Table
Example
let str = t1.render();
console.log(str); //outputs table
$ grunt test
$ grunt st
node script.js --color=always
$ grunt tags
$ grunt watch
Copyright 2015-2018, Tecfu.
FAQs
Node cli table
The npm package tty-table receives a total of 0 weekly downloads. As such, tty-table popularity was classified as not popular.
We found that tty-table demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.