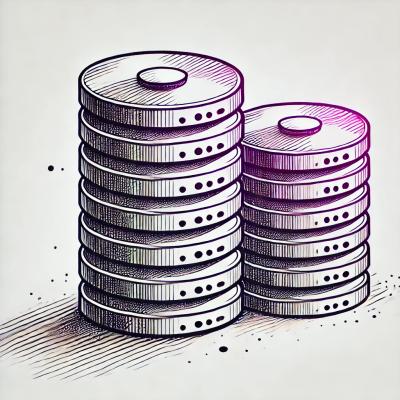
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
tus-js-client
Advanced tools
The tus-js-client is a JavaScript client for the tus resumable upload protocol. It allows you to upload large files to a server in a reliable and resumable manner, meaning that if the upload is interrupted, it can be resumed from where it left off.
Resumable File Upload
This feature allows you to upload a file to a server in a resumable manner. The upload can be paused and resumed, and it will automatically retry if it fails.
const tus = require('tus-js-client');
const file = document.querySelector('input[type="file"]').files[0];
const upload = new tus.Upload(file, {
endpoint: 'https://tusd.tusdemo.net/files/',
retryDelays: [0, 1000, 3000, 5000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log('Failed because: ' + error);
},
onProgress: function(bytesUploaded, bytesTotal) {
const percentage = (bytesUploaded / bytesTotal * 100).toFixed(2);
console.log(bytesUploaded, bytesTotal, percentage + '%');
},
onSuccess: function() {
console.log('Download %s from %s', upload.file.name, upload.url);
}
});
upload.start();
Custom Headers
This feature allows you to add custom headers to the upload request, such as an Authorization header for authentication purposes.
const tus = require('tus-js-client');
const file = document.querySelector('input[type="file"]').files[0];
const upload = new tus.Upload(file, {
endpoint: 'https://tusd.tusdemo.net/files/',
headers: {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
},
onError: function(error) {
console.log('Failed because: ' + error);
},
onSuccess: function() {
console.log('Download %s from %s', upload.file.name, upload.url);
}
});
upload.start();
Chunked Uploads
This feature allows you to upload files in chunks, which can be useful for large files. You can specify the chunk size, and the upload will be split into multiple smaller requests.
const tus = require('tus-js-client');
const file = document.querySelector('input[type="file"]').files[0];
const upload = new tus.Upload(file, {
endpoint: 'https://tusd.tusdemo.net/files/',
chunkSize: 5242880, // 5MB
onError: function(error) {
console.log('Failed because: ' + error);
},
onSuccess: function() {
console.log('Download %s from %s', upload.file.name, upload.url);
}
});
upload.start();
Uppy is a sleek, modular file uploader that integrates well with various backends. It supports resumable uploads using the tus protocol, just like tus-js-client, but also offers additional features like drag-and-drop, webcam support, and more.
Fine Uploader is a dependency-free JavaScript library for file uploading. It supports chunked and resumable uploads, similar to tus-js-client, but also provides a wide range of customization options and a comprehensive set of features for handling file uploads.
Resumable.js is a JavaScript library providing multiple simultaneous, stable, and resumable uploads via the HTML5 File API. It is similar to tus-js-client in that it supports resumable uploads, but it uses a different protocol and has a different set of features.
tus is a protocol based on HTTP for resumable file uploads. Resumable means that an upload can be interrupted at any moment and can be resumed without re-uploading the previous data again. An interruption may happen willingly, if the user wants to pause, or by accident in case of an network issue or server outage.
tus-js-client is a pure JavaScript client for the tus resumable upload protocol and can be used inside browsers, Node.js, React Native and Apache Cordova applications.
Protocol version: 1.0.0
This branch contains tus-js-client v4. If you are looking for the previous major release, after which breaking changes have been introduced, please look at the v3.1.3 tag.
input.addEventListener('change', function (e) {
// Get the selected file from the input element
var file = e.target.files[0]
// Create a new tus upload
var upload = new tus.Upload(file, {
endpoint: 'http://localhost:1080/files/',
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type,
},
onError: function (error) {
console.log('Failed because: ' + error)
},
onProgress: function (bytesUploaded, bytesTotal) {
var percentage = ((bytesUploaded / bytesTotal) * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + '%')
},
onSuccess: function () {
console.log('Download %s from %s', upload.file.name, upload.url)
},
})
// Check if there are any previous uploads to continue.
upload.findPreviousUploads().then(function (previousUploads) {
// Found previous uploads so we select the first one.
if (previousUploads.length) {
upload.resumeFromPreviousUpload(previousUploads[0])
}
// Start the upload
upload.start()
})
})
This project is licensed under the MIT license, see LICENSE
.
FAQs
A pure JavaScript client for the tus resumable upload protocol
The npm package tus-js-client receives a total of 156,496 weekly downloads. As such, tus-js-client popularity was classified as popular.
We found that tus-js-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.