Comparing version 2.0.0 to 2.1.0
// Load modules | ||
var Hapi = require('hapi'); | ||
var Tv = require('../'); | ||
// Declare internals | ||
@@ -23,3 +23,3 @@ | ||
server.pack.require('../', function (err) { | ||
server.pack.register(Tv, function (err) { | ||
@@ -35,2 +35,2 @@ if (err) { | ||
}); | ||
}); | ||
}); |
@@ -35,3 +35,14 @@ // Load modules | ||
path: settings.endpoint + '/{file*2}', | ||
handler: { directory: { path: __dirname + '/../public', listing: false, index: false } } | ||
config: { | ||
handler: { | ||
directory: { | ||
path: __dirname + '/../public', | ||
listing: false, | ||
index: false | ||
} | ||
}, | ||
plugins: { | ||
lout: false | ||
} | ||
} | ||
}); | ||
@@ -55,3 +66,3 @@ | ||
endpoint: settings.endpoint, | ||
host: settings.host || server.info.host, | ||
host: settings.publicHost || settings.host || server.info.host, | ||
port: server.info.port | ||
@@ -68,2 +79,5 @@ }; | ||
reply.view(settings.template, context); | ||
}, | ||
plugins: { | ||
lout: false | ||
} | ||
@@ -70,0 +84,0 @@ } |
{ | ||
"name": "tv", | ||
"description": "Interactive debug console plugin for hapi", | ||
"version": "2.0.0", | ||
"author": "Eran Hammer <eran@hueniverse.com> (http://hueniverse.com)", | ||
"contributors":[ | ||
"version": "2.1.0", | ||
"author": "Eran Hammer <eran@hammer.io> (http://hueniverse.com)", | ||
"contributors": [ | ||
"Wyatt Preul <wpreul@gmail.com>", | ||
@@ -12,3 +12,3 @@ "Van Nguyen <the.gol.effect@gmail.com>", | ||
], | ||
"repository": "git://github.com/spumko/tv", | ||
"repository": "git://github.com/hapijs/tv", | ||
"main": "index", | ||
@@ -22,3 +22,3 @@ "keywords": [ | ||
"engines": { | ||
"node": ">=0.10.22" | ||
"node": ">=0.10.30" | ||
}, | ||
@@ -28,3 +28,3 @@ "dependencies": { | ||
"ws": "0.4.x", | ||
"handlebars": "1.3.x" | ||
"handlebars": "2.0.x" | ||
}, | ||
@@ -35,4 +35,5 @@ "peerDependencies": { | ||
"devDependencies": { | ||
"hapi": "6.x.x", | ||
"lab": "3.x.x" | ||
"code": "1.x.x", | ||
"hapi": "7.x.x", | ||
"lab": "5.x.x" | ||
}, | ||
@@ -43,7 +44,7 @@ "scripts": { | ||
"licenses": [ | ||
{ | ||
{ | ||
"type": "BSD", | ||
"url": "http://github.com/spumko/tv/raw/master/LICENSE" | ||
"url": "http://github.com/hapijs/tv/raw/master/LICENSE" | ||
} | ||
] | ||
} |
@@ -1,8 +0,9 @@ | ||
<a href="https://github.com/spumko"><img src="https://raw.github.com/spumko/spumko/master/images/from.png" align="right" /></a> | ||
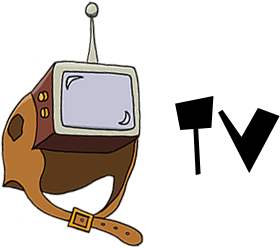 | ||
Interactive debug console plugin for [**hapi**](https://github.com/spumko/hapi) | ||
Interactive debug console plugin for [**hapi**](https://github.com/hapijs/hapi) | ||
[](http://travis-ci.org/spumko/tv) | ||
[](http://travis-ci.org/hapijs/tv) | ||
Lead Maintainer: [Wyatt Preul](https://github.com/wpreul) | ||
The debug console is a simple web page in which developers can subscribe to a debug id (or * for all), and then include that | ||
@@ -9,0 +10,0 @@ debug id as an extra query parameter with each request. The server will use WebSocket to stream the subscribed request logs to |
// Load modules | ||
var Code = require('code'); | ||
var Hapi = require('hapi'); | ||
var Lab = require('lab'); | ||
var Hapi = require('hapi'); | ||
var Ws = require('ws'); | ||
@@ -16,41 +17,91 @@ var Tv = require('../'); | ||
var expect = Lab.expect; | ||
var before = Lab.before; | ||
var after = Lab.after; | ||
var describe = Lab.experiment; | ||
var it = Lab.test; | ||
var lab = exports.lab = Lab.script(); | ||
var describe = lab.describe; | ||
var it = lab.it; | ||
var expect = Code.expect; | ||
describe('Tv', function () { | ||
it('reports a request event', function (done) { | ||
it('reports a request event', function (done) { | ||
var server = new Hapi.Server(0); | ||
var server = new Hapi.Server(0); | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
reply('1'); | ||
} | ||
}); | ||
reply('1'); | ||
} | ||
server.pack.register({ plugin: Tv, options: { port: 0 } }, function (err) { | ||
expect(err).to.not.exist(); | ||
server.inject('/debug/console', function (res) { | ||
expect(res.statusCode).to.equal(200); | ||
expect(res.result).to.contain('Debug Console'); | ||
var host = res.result.match(/var host = '([^']+)'/)[1]; | ||
var port = res.result.match(/var port = (\d+)/)[1]; | ||
var ws = new Ws('ws://' + host + ':' + port); | ||
ws.once('open', function () { | ||
ws.send('*'); | ||
setTimeout(function () { | ||
server.inject('/?debug=123', function (res) { | ||
expect(res.result).to.equal('1'); | ||
}); | ||
}, 100); | ||
}); | ||
ws.once('message', function (data, flags) { | ||
expect(JSON.parse(data).data.agent).to.equal('shot'); | ||
done(); | ||
}); | ||
}); | ||
}); | ||
}); | ||
server.pack.register({ plugin: Tv, options: { port: 0 } }, function (err) { | ||
it('handles reconnects gracefully', function (done) { | ||
expect(err).to.not.exist; | ||
var server = new Hapi.Server(0); | ||
server.inject('/debug/console', function (res) { | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
expect(res.statusCode).to.equal(200); | ||
expect(res.result).to.contain('Debug Console'); | ||
reply('1'); | ||
} | ||
}); | ||
var host = res.result.match(/var host = '([^']+)'/)[1]; | ||
var port = res.result.match(/var port = (\d+)/)[1]; | ||
var ws = new Ws('ws://' + host + ':' + port); | ||
server.pack.register({ plugin: Tv, options: { port: 0, host: 'localhost' }}, function (err) { | ||
ws.once('open', function () { | ||
expect(err).to.not.exist(); | ||
ws.send('*'); | ||
server.inject('/debug/console', function (res) { | ||
expect(res.statusCode).to.equal(200); | ||
expect(res.result).to.contain('Debug Console'); | ||
var host = res.result.match(/var host = '([^']+)'/)[1]; | ||
var port = res.result.match(/var port = (\d+)/)[1]; | ||
var ws1 = new Ws('ws://' + host + ':' + port); | ||
ws1.once('open', function () { | ||
ws1.send('*'); | ||
ws1.close(); | ||
var ws2 = new Ws('ws://' + host + ':' + port); | ||
ws2.once('open', function () { | ||
ws2.send('*'); | ||
setTimeout(function () { | ||
@@ -65,3 +116,4 @@ | ||
ws.once('message', function (data, flags) { | ||
// Shouldn't get called | ||
ws2.once('message', function (data, flags) { | ||
@@ -74,79 +126,53 @@ expect(JSON.parse(data).data.agent).to.equal('shot'); | ||
}); | ||
}); | ||
it('handles reconnects gracefully', function (done) { | ||
it('uses specified hostname', function (done) { | ||
var server = new Hapi.Server(0); | ||
var server = new Hapi.Server(0); | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
reply('1'); | ||
} | ||
}); | ||
reply('1'); | ||
} | ||
}); | ||
server.pack.register({ plugin: Tv, options: { port: 0, host: 'localhost' }}, function (err) { | ||
server.pack.register({plugin: Tv, options: { host: '127.0.0.1', port: 0 } }, function (err) { | ||
expect(err).to.not.exist; | ||
expect(err).to.not.exist(); | ||
done(); | ||
}); | ||
}); | ||
server.inject('/debug/console', function (res) { | ||
it('uses specified public hostname', function (done) { | ||
expect(res.statusCode).to.equal(200); | ||
expect(res.result).to.contain('Debug Console'); | ||
var server = new Hapi.Server(0); | ||
var host = res.result.match(/var host = '([^']+)'/)[1]; | ||
var port = res.result.match(/var port = (\d+)/)[1]; | ||
var ws1 = new Ws('ws://' + host + ':' + port); | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
ws1.once('open', function () { | ||
ws1.send('*'); | ||
ws1.close(); | ||
var ws2 = new Ws('ws://' + host + ':' + port); | ||
ws2.once('open', function () { | ||
ws2.send('*'); | ||
setTimeout(function () { | ||
server.inject('/?debug=123', function (res) { | ||
expect(res.result).to.equal('1'); | ||
}); | ||
}, 100); | ||
}); | ||
// Shouldn't get called | ||
ws2.once('message', function (data, flags) { | ||
expect(JSON.parse(data).data.agent).to.equal('shot'); | ||
done(); | ||
}); | ||
}); | ||
}); | ||
}); | ||
reply('1'); | ||
} | ||
}); | ||
it('uses specified hostname', function (done) { | ||
server.pack.register({ plugin: Tv, options: { port: 0, host: 'localhost', publicHost: '127.0.0.1' }}, function (err) { | ||
var server = new Hapi.Server(0); | ||
expect(err).to.not.exist(); | ||
server.route({ | ||
method: 'GET', | ||
path: '/', | ||
handler: function (request, reply) { | ||
server.inject('/debug/console', function (res) { | ||
reply('1'); | ||
} | ||
}); | ||
expect(res.statusCode).to.equal(200); | ||
expect(res.result).to.contain('Debug Console'); | ||
server.pack.register({plugin: Tv, options: { host: '127.0.0.1', port: 0 } }, function (err) { | ||
var host = res.result.match(/var host = '([^']+)'/)[1]; | ||
expect(err).to.not.exist; | ||
expect(host).to.equal('127.0.0.1'); | ||
done(); | ||
}); | ||
}); | ||
}); | ||
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
537412
21
12633
44
3
+ Addedhandlebars@2.0.0(transitive)
- Removedhandlebars@1.3.0(transitive)
Updatedhandlebars@2.0.x